Java Timer
Rashmi Patidar
Oct 12, 2023
Java
Java Timer
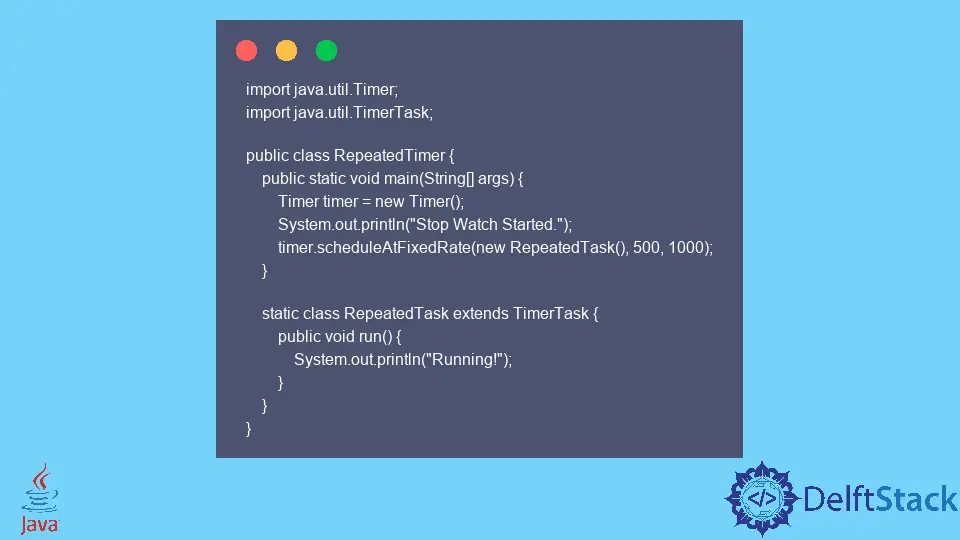
Timer
is a service available in the Java programming language that allows users to schedule a future event. These future events can be one time or repeated at regular time intervals. There can be triggers that we use to initiate future events.
Schedule a One-Time Task With Timer
in Java
import java.util.Timer;
import java.util.TimerTask;
public class StopWatch {
Timer timer;
public StopWatch(int seconds) {
timer = new Timer();
timer.schedule(new StopTask(), seconds * 1000);
}
public static void main(String[] args) {
new StopWatch(10);
System.out.println("StopWatch Started.");
}
class StopTask extends TimerTask {
public void run() {
System.out.println("Time Up!");
timer.cancel();
}
}
}
Below is the never-ending outcome of the future event scheduled.
Running!
Running!
Running!
Running!
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Author: Rashmi Patidar
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn