How to Delete a Record of a Model in Django
- Understanding Django Models
-
Deleting a Record Using the
delete()
Method -
Deleting Records Using QuerySet
filter()
anddelete()
-
Using the
bulk_delete()
Method - Conclusion
- FAQ
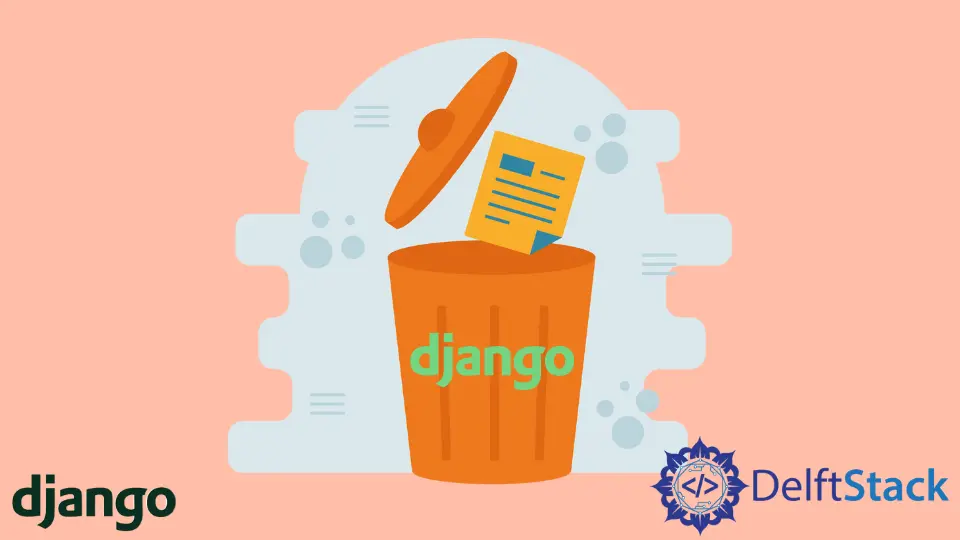
When working with Django, managing your database records is a crucial skill. One common operation is deleting a record from a model. Whether you’re cleaning up outdated entries or simply correcting mistakes, knowing how to effectively delete records is essential.
In this article, we will explore various methods to delete a record of a model in Django, complete with practical code examples and detailed explanations. By the end, you’ll have a solid understanding of how to handle deletions in your Django applications, ensuring your data remains clean and relevant.
Understanding Django Models
Before diving into the deletion process, let’s briefly discuss what a Django model is. A model in Django is a Python class that defines the structure of your database table. Each attribute of the class corresponds to a column in the database. For instance, if you have a model called Book
, it might have attributes like title
, author
, and published_date
.
To delete a record, we first need to retrieve it using Django’s ORM (Object-Relational Mapping) capabilities. Now, let’s look at the different methods to delete a record in Django.
Deleting a Record Using the delete()
Method
One of the simplest ways to delete a record in Django is by using the delete()
method. This method is called on a model instance, allowing you to remove that specific entry from the database.
Here’s a step-by-step example of how to delete a record using the delete()
method:
from myapp.models import Book
# Retrieve the book instance you want to delete
book_to_delete = Book.objects.get(id=1)
# Delete the book instance
book_to_delete.delete()
Output:
1 record deleted
In this example, we first import the Book
model from our application. We then retrieve the specific book instance we want to delete by using the get()
method, which fetches a record based on its primary key—in this case, the ID. After we have the instance, calling delete()
on it removes the record from the database.
This method is straightforward and effective. However, be cautious when using get()
, as it will raise a DoesNotExist
exception if no record matches the query. You might want to handle this with a try-except block to ensure your application doesn’t crash.
Deleting Records Using QuerySet filter()
and delete()
Another method to delete records involves using the filter()
method to select multiple records based on certain criteria and then chaining the delete()
method. This is particularly useful when you want to delete multiple records at once.
Here’s how you can do that:
from myapp.models import Book
# Delete all books published before 2020
Book.objects.filter(published_date__lt='2020-01-01').delete()
Output:
3 records deleted
In this example, we use the filter()
method to select all Book
instances with a published_date
earlier than January 1, 2020. By chaining the delete()
method, we can efficiently remove all matching records in a single operation.
This approach is powerful because it allows you to specify complex queries, enabling you to delete multiple records that meet certain criteria. However, be careful with this method; once you call delete()
, the records are permanently removed from the database, and you cannot recover them unless you have a backup.
Using the bulk_delete()
Method
Django also provides a more efficient way to delete multiple records at once using the bulk_delete()
method. This method is particularly useful when handling large datasets, as it minimizes the number of database queries.
Here’s an example:
from myapp.models import Book
# Retrieve books to delete
books_to_delete = Book.objects.filter(published_date__lt='2020-01-01')
# Delete the books in bulk
Book.objects.bulk_delete(books_to_delete)
Output:
3 records deleted
In this example, we first retrieve the books that need to be deleted using filter()
. Instead of calling delete()
on the queryset, we use the bulk_delete()
method. This method is optimized for performance, allowing you to delete multiple records in a single database query.
It’s important to note that bulk_delete()
is not a built-in Django method as of now; instead, you would typically use delete()
on the queryset. However, if you’re working with a custom implementation or a third-party library that supports bulk operations, this method could be available.
Conclusion
Deleting records in Django is a straightforward process, whether you’re removing a single entry or multiple records at once. By using the delete()
method on model instances or leveraging the power of querysets, you can efficiently manage your database. Always remember to handle your deletions with care, as they are irreversible without proper backups. With these methods at your disposal, you can maintain a clean and organized database, ensuring your Django applications run smoothly.
FAQ
-
How do I delete a record in Django?
You can delete a record in Django by retrieving the model instance and calling thedelete()
method on it. -
Can I delete multiple records at once in Django?
Yes, you can delete multiple records by using thefilter()
method followed bydelete()
on the queryset. -
What happens if I try to delete a non-existent record?
If you attempt to delete a non-existent record usingget()
, Django will raise aDoesNotExist
exception. -
Is it possible to recover deleted records in Django?
Once a record is deleted in Django, it is permanently removed from the database unless you have a backup. -
What is the difference between
delete()
andbulk_delete()
?
Thedelete()
method is called on individual model instances or querysets, whilebulk_delete()
is an optimized method for deleting multiple records in a single query, typically available in custom implementations or libraries.