How to Create Objects in Django
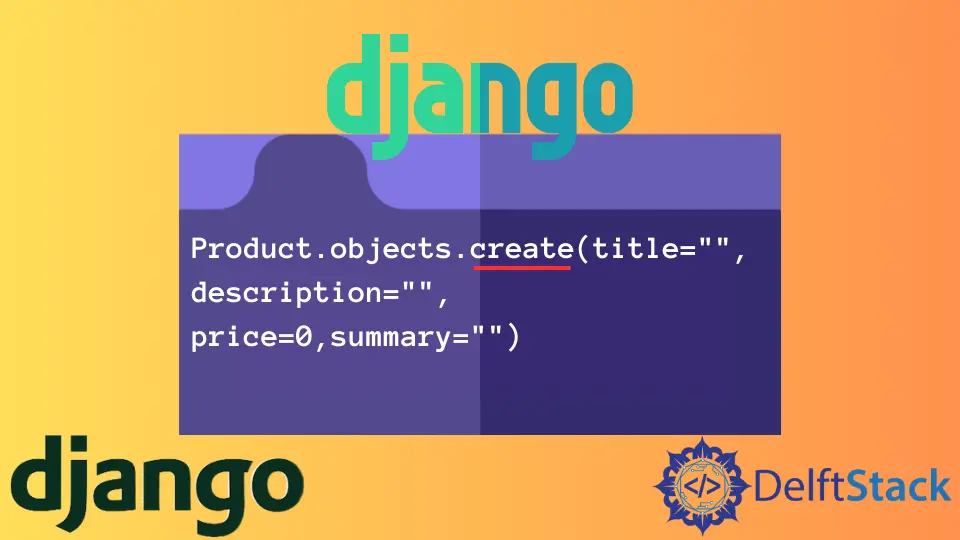
This article explains what a model is and how to create objects using the create()
method. We will see how to work the save()
method in Django.
Create Objects Using the create()
Method in Django
A model in Django is a class that represents a single table in a database. We could have each type of data in our application represented by its model.
Each model then maps to a single table in a database.
For example, if we have four models, articles, users, authors, and books, we would have four tables in a database. A single model maps each table.
Let’s see how to create objects of a model in the database.
We are going to use the Python shell to create new objects. We need to use the admin to ensure that we are at the root of our Django project.
ls
Output:
db.sqlite3 manage.py products trydjango
Now, we are going to run another command. So when we run this command, all of the Django project stuff will work inside a Python interpreter.
We will see a normal Python interpreter when we hit Enter, but it is not because we can run the following command.
python manage.py shell
We have imported the Product
model from the models.py
file. We can use our Product
model in the current shell if we hit Enter.
from products.models import Product
We have built-in Django command to retrieve all objects from the defined model.
Product.objects.all()
Now, we can see there is only one item, and that is because we only saved one.
<QuerySet[<Product: Product object (1)>]>
If we want to see more objects, we only need to create more objects using the following command.
Product.objects.create()
Suppose our Product
model has four fields. These four fields must pass in the create()
method to create a new object.
Let’s create a new object by writing the following command.
Product.objects.create(
title="New product",
description="Another one",
price=1222,
summary="The best product",
)
After writing this command, when we hit the Enter, it will create a new object.
<Product: Product object (2)>
So now, if we try to retrieve all objects, we will see another newly created item.
Product.objects.all()
Now, we can see the second item of the query list.
<QuerySet[<Product: Product object (1)>,<Product: Product object (2)>]>
When we go to the admin panel, we will see new objects there.
Now, we will look at using the save()
method. Suppose we have the following code, and we need to save all fields of the defined model.
We need to pass all fields in our model class and create an object to save this using the save()
method.
We defined the product_id
in the above code, so now we can access the product using its ID. In the product_id
, we set the primary_key
equal to True
.
The save()
method works for two conditions meaning if the given product_id
already exists in the database, the object will be updated with different field values.
If the save()
method does not find the primary_key
attribute set to True
in product_id
, or if the given ID does not exist in the database, it will produce an error. Using the Product.objects.create()
, we will only be able to insert new objects.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn