How to Create Field for Multiple Choices in Django
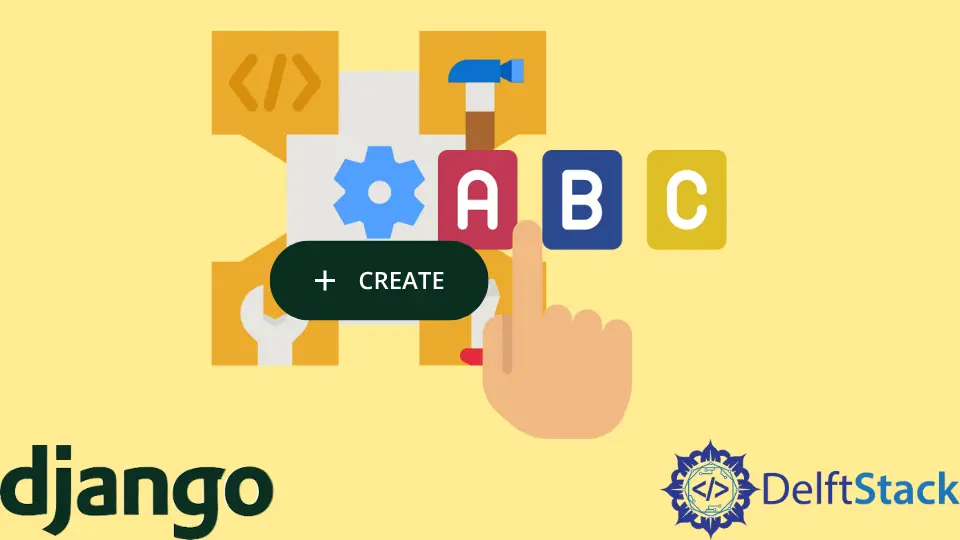
This article will present how to make a field for multiple choices and show you how to allow users to select multiple options in Django.
Create Field for Multiple Choices in Django
Suppose you have a demosite
website where users add their favorite books. Django has a default way that when you make choices, they will be a drop-down menu and limit a user to choose only one item.
Suppose we want to see multiple options for choosing one book; we will have more than one book option. Let’s see how we will do this.
So, first of all, we will get our Book
model class ready. Open the models.py
file and create a tuple with different book names.
Code:
from django.db import models
class Book(models.Model):
BOOK_CHOICES = (
("Parks of being a wallflower", "Parks of being a wallflower"),
("All the bright places", "All the bright places"),
("The girl on the train", "The girl on the train"),
("Django", "Django"),
)
Now, we need to create the first column or field in the database, which would be a title
. The title
field would be a CharField
.
In this title
field, we will pass the BOOK_CHOICES
tuple to the choices
argument. The choices
argument will allow us to choose an option from the drop-down menu.
Code:
title = models.CharField(max_length=100, choices=BOOK_CHOICES)
Register this Book
model in the admin.py
file. So first, we need to import our Book
model and then register it using admin.site.register()
.
Code:
from .models import Book
admin.site.register(Book)
Now, we need to open the setting.py
file, find the INSTALLED_APPS
list, and add our Django app to this list before migration. In this project, we are using a demo
app.
Code:
INSTALLED_APPS = [
"demo",
]
Let’s open a terminal where the manage.py
file directory exists and run the following commands to make migrations.
Commands:
python manage.py makemigrations demo
python manage.py migrate
Now, we have to create a superuser before running the server.
Command:
python manage.py createsuperuser
After running this command, we need to fulfill the user name, email, and password requirements. Then, we are good to run the server using the following command.
Command:
python manage.py runserver
After running the project, we need to open the admin page and log in to the page to access the admin panel.
Output:
Here, you can see that we can only choose one option.
If you want your user to choose more than one option, you must follow the instructions.
We will need a django-multiselectfield
module to use the multiple option feature in our project. It is easy to use; follow this link to be able to use this feature.
At this moment, our models.py
file contains the following code.
Code:
from multiselectfield import MultiSelectField
from django.db import models
class Book(models.Model):
BOOK_CHOICES = (
("Parks of being a wallflower", "Parks of being a wallflower"),
("All the bright places", "All the bright places"),
("The girl on the train", "The girl on the train"),
("Django", "Django"),
)
title = MultiSelectField(choices=BOOK_CHOICES)
Output:
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn