Meta Class in Django Models
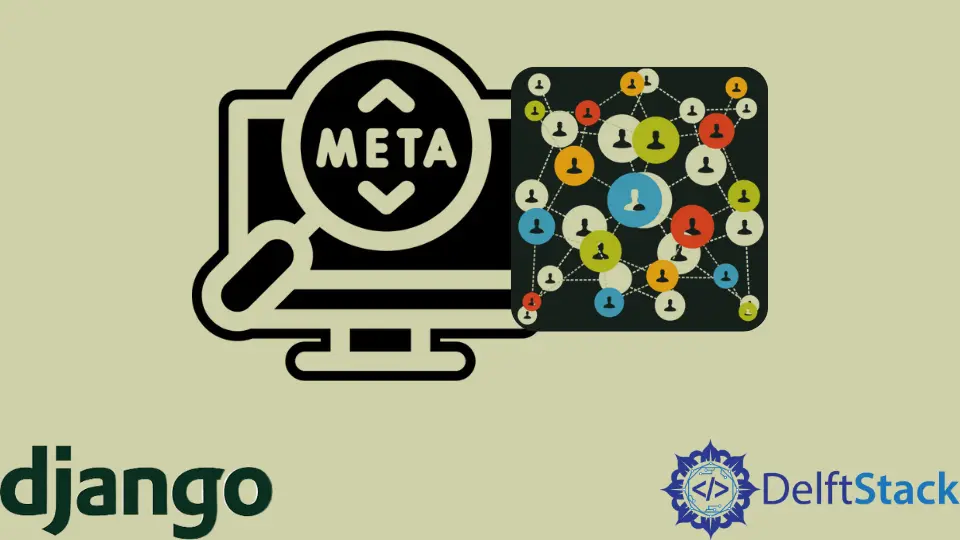
Metadata refers to a specific set of data that provides information about another data. In Django, we use Django Models to design our database’s tables and their fields. If we have to add some data about the model itself, we use the Meta
class. Find out more about the Meta
class in Django models in this article.
the Meta
Class in Django
The Meta
class is an inner class, which means it’s defined inside the model as follows:
from django.db import models
class MyModel(models.Model):
...
class Meta:
...
The Meta
class can be used to define various things about the model such as the permissions, database name, singular and plural names, abstraction, ordering, and etc. Adding Meta
classes to Django models is entirely optional.
This class also comes with many options you can configure. The following are some of the commonly used meta options; you can explore all the meta options here
Django Meta Option - Abstract
This option is used to define whether the model is abstract or not; they work the same as abstract classes. Abstract classes are the ones that can’t be instantiated and can only be extended or inherited.
Models that are set as an abstract can only be inherited. One can use this option if there are multiple models with common fields.
from django.db import models
class Human(models.Model):
genders = (
("M", "Male"),
("F", "Female"),
("NB", "Non-binary"),
("T", "Transgender"),
("I", "Intersex"),
("O", "Other"),
("PNTS", "Prefer not to say"),
)
name = models.CharField(max_length=200)
age = models.IntegerField(default=0)
gender = models.CharField(max_length=50, choices=genders)
class Meta:
abstract = True # Important
class Teacher(Human):
subject = models.CharField(max_length=200)
class Student(Human):
grade = models.IntegerField(default=0)
Here, the Teacher
and the Student
models will have all the fields inside the Human
model. Inside the database, only the Teacher
and Student
models will be created.
Django Meta Option - db_table
This option is used to set the name that should be used to identify the table inside the database. For example: if I do something as follows, the name of my model will be job
in the database.
from django.db import models
class JobPosting(models.Model):
class Meta:
db_table = "job"
Django Meta Option - Ordering
This option takes a list of string values, which are model fields. It’s used to define the ordering of objects of a model. When the objects of this model are retrieved, they will be present in this ordering.
from django.db import models
class JobPosting(models.Model):
dateTimeOfPosting = models.DateTimeField(auto_now_add=True)
class Meta:
ordering = ["-dateTimeOfPosting"]
In the example above, the objects retrieved will be ordered based on the dateTimeOfPosting
field in descending order. (The -
prefix is used to define a descending ordering.)
Django Meta Option - verbose_name
This option is used to define a human-readable singular name for a model and will overwrite Django’s default naming convention. This name will reflect in the admin panel (/admin/
) as well.
from django.db import models
class JobPosting(models.Model):
class Meta:
verbose_name = "Job Posting"
Django Meta Option - Verbose_name_plural
This option is used to define a human-readable plural name for a model, which again will overwrite Django’s default naming convention. This name will reflect in the admin panel (/admin/
) as well.
from django.db import models
class JobPosting(models.Model):
class Meta:
verbose_name_plural = "Job Postings"