How to Switch Frames in Tkinter
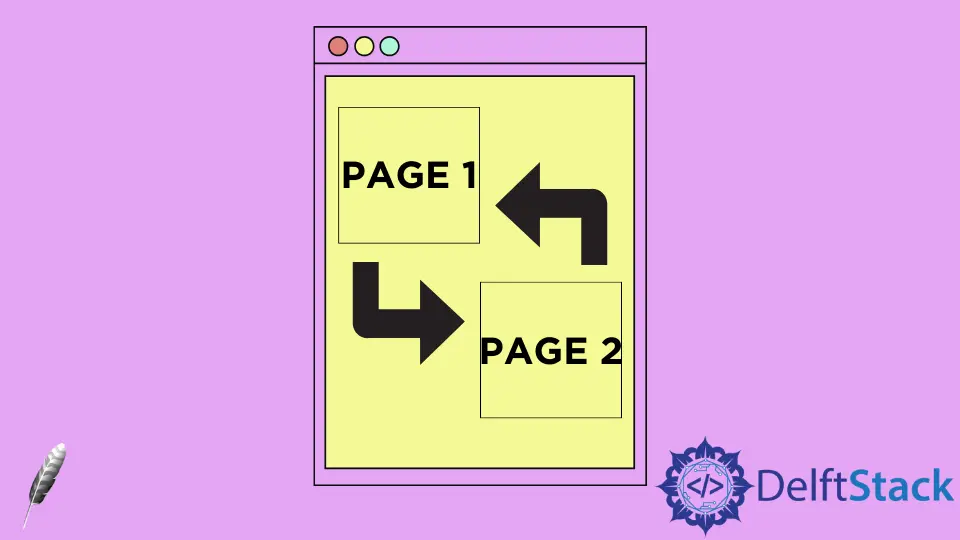
We need to move to a different section of the Tkinter GUI by clicking a Tkinter button and then could return to the main section after clicking the button in this new section.
Switch Frames by Destroying the Old Frame and Replacing With the New One
try:
import Tkinter as tk
except:
import tkinter as tk
class SampleApp(tk.Tk):
def __init__(self):
tk.Tk.__init__(self)
self._frame = None
self.switch_frame(StartPage)
def switch_frame(self, frame_class):
new_frame = frame_class(self)
if self._frame is not None:
self._frame.destroy()
self._frame = new_frame
self._frame.pack()
class StartPage(tk.Frame):
def __init__(self, master):
tk.Frame.__init__(self, master)
tk.Label(self, text="Start page", font=("Helvetica", 18, "bold")).pack(
side="top", fill="x", pady=5
)
tk.Button(
self, text="Go to page one", command=lambda: master.switch_frame(PageOne)
).pack()
tk.Button(
self, text="Go to page two", command=lambda: master.switch_frame(PageTwo)
).pack()
class PageOne(tk.Frame):
def __init__(self, master):
tk.Frame.__init__(self, master)
tk.Frame.configure(self, bg="blue")
tk.Label(self, text="Page one", font=("Helvetica", 18, "bold")).pack(
side="top", fill="x", pady=5
)
tk.Button(
self,
text="Go back to start page",
command=lambda: master.switch_frame(StartPage),
).pack()
class PageTwo(tk.Frame):
def __init__(self, master):
tk.Frame.__init__(self, master)
tk.Frame.configure(self, bg="red")
tk.Label(self, text="Page two", font=("Helvetica", 18, "bold")).pack(
side="top", fill="x", pady=5
)
tk.Button(
self,
text="Go back to start page",
command=lambda: master.switch_frame(StartPage),
).pack()
if __name__ == "__main__":
app = SampleApp()
app.mainloop()
switch_frame(self, frame_class)
method destroys the old frame and then replaces with the new frame frame_class
.
tk.Button(self, text="Go to page one", command=lambda: master.switch_frame(PageOne)).pack()
passes the argument frame PageOne
to the button command switch_frame
. It will call swtich_frame
function after the button is clicked.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook