Pandas loc 대 iloc
-
.loc()
메소드를 사용하여 인덱스 및 열 레이블을 지정하는 DataFrame에서 특정 값 선택 -
.loc()
메소드를 사용하여 데이터 프레임에서 특정 열 선택 -
.loc()
메서드를 사용하여 열에 조건을 적용하여 행 필터링 -
iloc
을 사용하여 인덱스가있는 행 필터링 - DataFrame에서 특정 행과 열 필터링
-
iloc
을 사용하여 DataFrame에서 행 및 열 범위 필터링 -
Pandas
loc
대iloc
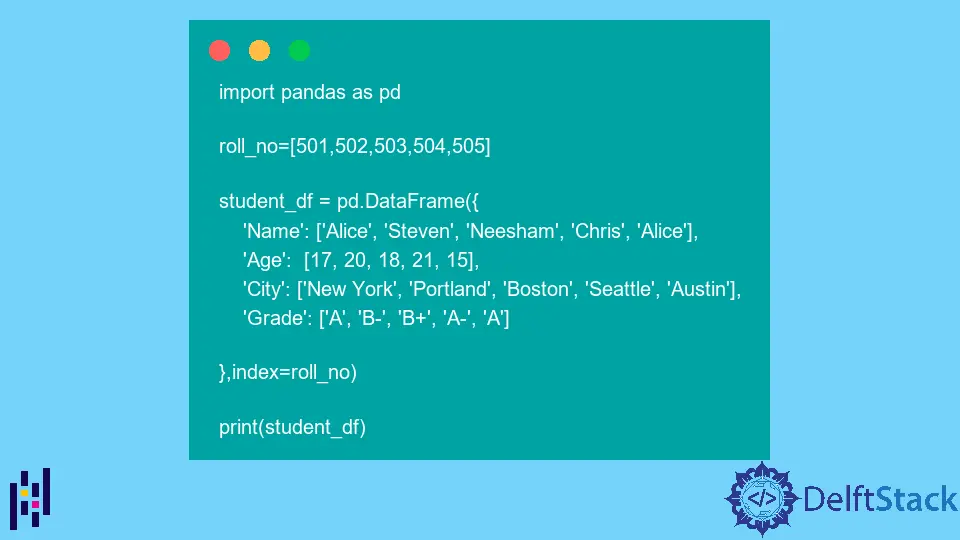
이 튜토리얼은 Python에서loc
및iloc
을 사용하여 Pandas DataFrame에서 데이터를 필터링하는 방법을 설명합니다. iloc
을 사용하여 DataFrame의 항목을 필터링하려면 행과 열에 정수 인덱스를 사용하고loc
을 사용하여 DataFrame의 항목을 필터링하려면 행과 열 이름을 사용합니다.
loc
을 사용한 데이터 필터링을 시연하기 위해 다음 예제에서 설명하는 DataFrame을 사용합니다.
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print(student_df)
출력:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
.loc()
메소드를 사용하여 인덱스 및 열 레이블을 지정하는 DataFrame에서 특정 값 선택
인덱스 레이블과 열 레이블을.loc()
메소드에 인수로 전달하여 주어진 인덱스 및 열 레이블에 해당하는 값을 추출 할 수 있습니다.
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print("The DataFrame of students with marks is:")
print(student_df)
print("")
print("The Grade of student with Roll No. 504 is:")
value = student_df.loc[504, "Grade"]
print(value)
출력:
The DataFrame of students with marks is:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
The Grade of student with Roll No. 504 is:
A-
인덱스 레이블이504
이고 열 레이블이Grade
인 DataFrame에서 값을 선택합니다. .loc()
메소드의 첫 번째 인수는 인덱스 이름을 나타내고 두 번째 인수는 열 이름을 나타냅니다.
.loc()
메소드를 사용하여 데이터 프레임에서 특정 열 선택
.loc()
메소드를 사용하여 DataFrame에서 필요한 열을 필터링 할 수도 있습니다. 필수 열 이름 목록을.loc()
메소드에 두 번째 인수로 전달하여 지정된 열을 필터링합니다.
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print("The DataFrame of students with marks is:")
print(student_df)
print("")
print("The name and age of students in the DataFrame are:")
value = student_df.loc[:, ["Name", "Age"]]
print(value)
출력:
The DataFrame of students with marks is:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
The name and age of students in the DataFrame are:
Name Age
501 Alice 17
502 Steven 20
503 Neesham 18
504 Chris 21
505 Alice 15
.loc()
에 대한 첫 번째 인수는:
이며 DataFrame의 모든 행을 나타냅니다. 마찬가지로["Name", "Age"]
를 두 번째 인수로 DataFrame에서Name
및Age
열만 선택하도록 나타내는.loc()
메소드에 전달합니다.
.loc()
메서드를 사용하여 열에 조건을 적용하여 행 필터링
.loc()
메소드를 사용하여 열 값에 대해 지정된 조건을 충족하는 행을 필터링 할 수도 있습니다.
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print("The DataFrame of students with marks is:")
print(student_df)
print("")
print("Students with Grade A are:")
value = student_df.loc[student_df.Grade == "A"]
print(value)
출력:
The DataFrame of students with marks is:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
Students with Grade A are:
Name Age City Grade
501 Alice 17 New York A
505 Alice 15 Austin A
A
등급으로 DataFrame의 모든 학생을 선택합니다.
iloc
을 사용하여 인덱스가있는 행 필터링
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print("The DataFrame of students with marks is:")
print(student_df)
print("")
print("2nd and 3rd rows in the DataFrame:")
filtered_rows = student_df.iloc[[1, 2]]
print(filtered_rows)
출력:
The DataFrame of students with marks is:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
2nd and 3rd rows in the DataFrame:
Name Age City Grade
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
DataFrame에서 두 번째 및 세 번째 행을 필터링합니다.
행의 정수 인덱스를iloc
메소드에 인수로 전달하여 DataFrame에서 행을 필터링합니다. 여기서 두 번째 및 세 번째 행의 정수 색인은 색인이0
에서 시작하므로 각각1
및2
입니다.
DataFrame에서 특정 행과 열 필터링
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print("The DataFrame of students with marks is:")
print(student_df)
print("")
print("Filtered values from the DataFrame:")
filtered_values = student_df.iloc[[1, 2, 3], [0, 3]]
print(filtered_values)
출력:
The DataFrame of students with marks is:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
Filtered values from the DataFrame:
Name Grade
502 Steven B-
503 Neesham B+
504 Chris A-
첫 번째와 마지막 열, 즉 DataFrame에서 두 번째, 세 번째 및 네 번째 행의Name
및Grade
를 필터링합니다. 행의 정수 인덱스가있는 목록을 첫 번째 인수로 전달하고 열의 정수 인덱스가있는 목록을 두 번째 인수로iloc
메소드에 전달합니다.
iloc
을 사용하여 DataFrame에서 행 및 열 범위 필터링
행과 열의 범위를 필터링하기 위해 목록 슬라이스를 사용하고 각 행과 열의 슬라이스를iloc
메소드에 대한 인수로 전달할 수 있습니다.
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print("The DataFrame of students with marks is:")
print(student_df)
print("")
print("Filtered values from the DataFrame:")
filtered_values = student_df.iloc[1:4, 0:2]
print(filtered_values)
출력:
The DataFrame of students with marks is:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
Filtered values from the DataFrame:
Name Age
502 Steven 20
503 Neesham 18
504 Chris 21
DataFrame에서 두 번째, 세 번째 및 네 번째 행과 첫 번째 및 두 번째 열을 선택합니다. 1:4
는 색인이1
에서3
까지의 행을 나타내고4
는 범위에서 배타적입니다. 마찬가지로0:2
는 색인이0
에서1
까지 인 열을 나타냅니다.
Pandas loc
대iloc
loc()
를 사용하여 DataFrame에서rows
및columns
를 필터링하려면 필터링 할 행과 열의 이름을 전달해야합니다. 마찬가지로iloc()
을 사용하여 값을 필터링하기 위해 필터링 할rows
및columns
의 정수 인덱스를 전달해야합니다.
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Alice", "Steven", "Neesham", "Chris", "Alice"],
"Age": [17, 20, 18, 21, 15],
"City": ["New York", "Portland", "Boston", "Seattle", "Austin"],
"Grade": ["A", "B-", "B+", "A-", "A"],
},
index=roll_no,
)
print("The DataFrame of students with marks is:")
print(student_df)
print("")
print("Filtered values from the DataFrame using loc:")
iloc_filtered_values = student_df.loc[[502, 503, 504], ["Name", "Age"]]
print(iloc_filtered_values)
print("")
print("Filtered values from the DataFrame using iloc:")
iloc_filtered_values = student_df.iloc[[1, 2, 3], [0, 3]]
print(iloc_filtered_values)
The DataFrame of students with marks is:
Name Age City Grade
501 Alice 17 New York A
502 Steven 20 Portland B-
503 Neesham 18 Boston B+
504 Chris 21 Seattle A-
505 Alice 15 Austin A
Filtered values from the DataFrame using loc:
Name Age
502 Steven 20
503 Neesham 18
504 Chris 21
Filtered values from the DataFrame using iloc:
Name Grade
502 Steven B-
503 Neesham B+
504 Chris A-
loc
및iloc
을 사용하여 DataFrame에서 동일한 값을 필터링하는 방법을 표시합니다.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn