JavaFX 미디어 플레이어
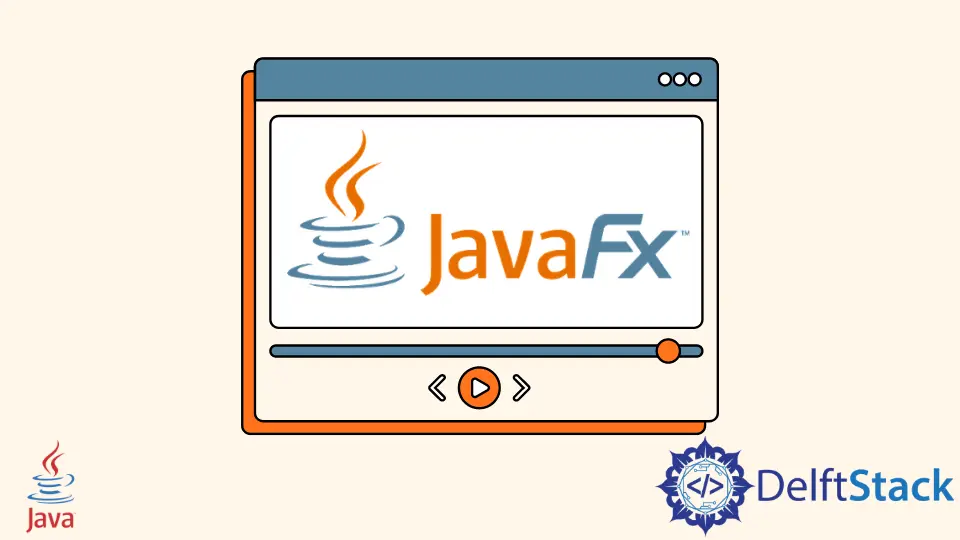
이 기사에서는 JavaFX를 사용하여 Java에서 미디어 플레이어를 만드는 방법을 배웁니다. 이를 위해 내장 JavaFX를 사용하고 수동으로 설정합니다.
내장 JavaFX를 사용하여 미디어 플레이어 만들기
내장된 JavaFX를 사용하려면 JavaFX가 포함되어 있으므로 Java 8이 필요합니다. 따로 설치할 필요가 없습니다.
이 섹션에서는 다음 도구를 사용합니다.
- 자바 8
- NetBeans 13(선택한 IDE를 사용할 수 있음)
예제 코드(Main.java
, 기본 클래스):
// write the package name (yours may be different)
package com.mycompany.main;
// import necessary libraries
import static javafx.application.Application.launch;
import java.net.URL;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.Pane;
import javafx.scene.media.Media;
import javafx.scene.media.MediaPlayer;
import javafx.scene.media.MediaView;
import javafx.stage.Stage;
// Main Class
public class Main extends Application {
// main method
public static void main(String[] args) {
launch(args);
} // end main method
/**
*
* @param primaryStage
* @throws Exception
*/
@Override
public void start(Stage primaryStage) throws Exception {
// path to the file
final URL resource = getClass().getResource("/music/audio.mp3");
// create Media Object and pass it the path of the audio file
Media mediafile = new Media(resource.toString());
// create MediaPlayer Object and pass the mediafile instance to it
MediaPlayer player = new MediaPlayer(mediafile);
// Add a mediaView, to display the media. Its necessary !
// This mediaView is added to a Pane
MediaView mediaView = new MediaView(player);
// Add to scene
Scene scene = new Scene(new Pane(mediaView), 400, 200);
// Show the stage
primaryStage.setTitle("Media Player");
primaryStage.setScene(scene);
primaryStage.show();
// Play the media once the stage is shown
player.play();
} // end start
} // end Main Class
JavaFX를 사용하려면 Application
클래스(Java 8 이후 Java의 표준 클래스)를 확장해야 하는 기본 시작 클래스가 필요합니다. 기본 시작 클래스의 이름은 Main
이며 Application
클래스도 확장
합니다.
Main
클래스는 Application
클래스의 하위 클래스라고 말할 수 있습니다. 따라서 모든 추상 메서드를 구현해야 하며 이것이 Main
클래스가 start()
메서드를 재정의하는 이유입니다.
start()
함수는 하나의 Stage
유형 매개변수를 허용합니다. JavaFX 앱의 모든 시각적 섹션이 표시되는 곳입니다.
Stage
유형 개체는 JavaFX 런타임에 의해 생성됩니다. 수동으로 만들 필요가 없습니다.
start()
메소드 내에서 오디오 파일의 경로를 가져와 resource
변수에 저장합니다. 이 변수는 Media
생성자에 문자열 형식으로 전달되고 MediaPlayer
생성자에는 추가로 전달됩니다. . 다음으로, 미디어를 표시/표시하기 위해 mediaView
를 추가합니다. 이는 필요합니다.
그런 다음 이 mediaView
가 Pane
에 추가됩니다. JavaFX 응용 프로그램의 창에 무언가를 표시하는 데 사용할 Stage
개체에 Scene
을 추가해야 합니다.
JavaFX 앱에 표시되어야 하는 모든 구성 요소는 장면
안에 있어야 합니다. 이 예에서는 미디어 보기와 함께 Scene
개체를 Stage
에 추가합니다.
그 후 제목을 정하고, 장면을 설정하고, 무대가 보여지면 미디어를 재생합니다.
이제 main
메서드로 이동합니다. main()
함수 없이 JavaFX 앱을 시작할 수 있지만 명령줄 매개변수를 애플리케이션에 전달해야 할 때 유용하다는 것을 알고 계십니까?
JavaFX를 설정하고 이를 사용하여 미디어 플레이어 만들기
JavaFX를 수동으로 설치하려면 다음이 필요합니다.
- 자바 18
- NetBeans 버전 13(선택한 IDE를 사용할 수 있음)
module-info.java
및pom.xml
파일을 업데이트하여javafx.controls
및javafx.media
를 설치합니다(모든 파일에 대한 전체 코드는 아래에 나와 있음).- Maven을 사용하여 종속성을 설치합니다. Gradle을 사용할 수 있습니다.
예제 코드(module-info.java
파일):
module com.mycompany.test {
requires javafx.controls;
requires javafx.media;
exports com.mycompany.test;
}
예제 코드(pom.xml
파일):
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="https://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.mycompany</groupId>
<artifactId>Test</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.release>11</maven.compiler.release>
<javafx.version>16</javafx.version>
<javafx.maven.plugin.version>0.0.6</javafx.maven.plugin.version>
</properties>
<dependencies>
<dependency>
<groupId>org.openjfx</groupId>
<artifactId>javafx-controls</artifactId>
<version>${javafx.version}</version>
</dependency>
<dependency>
<groupId>org.openjfx</groupId>
<artifactId>javafx-media</artifactId>
<version>${javafx.version}</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<release>${maven.compiler.release}</release>
</configuration>
</plugin>
<plugin>
<groupId>org.openjfx</groupId>
<artifactId>javafx-maven-plugin</artifactId>
<version>${javafx.maven.plugin.version}</version>
<configuration>
<mainClass>com.mycompany.test.App</mainClass>
</configuration>
</plugin>
</plugins>
</build>
</project>
예제 코드(App.java
, 기본 클래스):
// write the package name (yours may be different)
package com.mycompany.test;
// import necessary libraries
import java.net.URL;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.Pane;
import javafx.scene.media.Media;
import javafx.scene.media.MediaPlayer;
import javafx.scene.media.MediaView;
import javafx.stage.Stage;
/**
* JavaFX App
*/
public class App extends Application {
/**
*
* @param primaryStage
*/
@Override
public void start(Stage primaryStage) {
// path to the file
final URL resource = getClass().getResource("/music/video.mkv");
// create Media Object and pass it the path of the video/audio file
Media mediafile = new Media(resource.toString());
// create MediaPlayer Object and pass the mediafile instance to it
MediaPlayer player = new MediaPlayer(mediafile);
// Add a mediaView, to display the media. Its necessary !
// This mediaView is added to a Pane
MediaView mediaView = new MediaView(player);
// Add to scene
Scene scene = new Scene(new Pane(mediaView), 1080, 750);
// Show the stage
primaryStage.setTitle("Media Player");
primaryStage.setScene(scene);
primaryStage.show();
// Play the media once the stage is shown
player.play();
}
// main method
public static void main(String[] args) {
launch(args);
} // end main method
} // end App class
Java 애플리케이션에서 module-info.java
및 pom.xml
파일은 default package
및 Project Files
에 있습니다. 다음은 명확한 이해를 위해 Java 애플리케이션의 모든 파일을 스크린샷으로 나타낸 것입니다.
이 코드는 이전 섹션 와 동일하지만 차이점은 다음과 같습니다. JavaFX를 수동으로 설치하고 비디오 파일을 재생하십시오.