JavaFX 媒体播放器
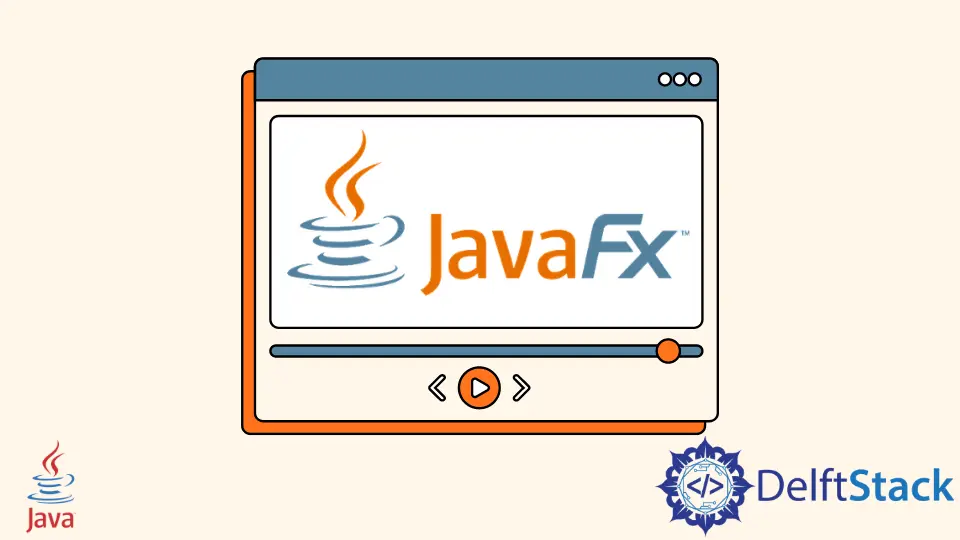
在本文中,我们将学习如何使用 JavaFX 在 Java 中制作媒体播放器。为此,我们将使用内置的 JavaFX 并手动进行设置。
使用内置 JavaFX 制作媒体播放器
要使用内置的 JavaFX,我们需要 Java 8,因为其中包含 JavaFX。我们不必单独安装它。
对于本节,我们使用以下工具。
- Java 8
- NetBeans 13(你可以使用你选择的任何 IDE)
示例代码(Main.java
,主类):
// write the package name (yours may be different)
package com.mycompany.main;
// import necessary libraries
import static javafx.application.Application.launch;
import java.net.URL;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.Pane;
import javafx.scene.media.Media;
import javafx.scene.media.MediaPlayer;
import javafx.scene.media.MediaView;
import javafx.stage.Stage;
// Main Class
public class Main extends Application {
// main method
public static void main(String[] args) {
launch(args);
} // end main method
/**
*
* @param primaryStage
* @throws Exception
*/
@Override
public void start(Stage primaryStage) throws Exception {
// path to the file
final URL resource = getClass().getResource("/music/audio.mp3");
// create Media Object and pass it the path of the audio file
Media mediafile = new Media(resource.toString());
// create MediaPlayer Object and pass the mediafile instance to it
MediaPlayer player = new MediaPlayer(mediafile);
// Add a mediaView, to display the media. Its necessary !
// This mediaView is added to a Pane
MediaView mediaView = new MediaView(player);
// Add to scene
Scene scene = new Scene(new Pane(mediaView), 400, 200);
// Show the stage
primaryStage.setTitle("Media Player");
primaryStage.setScene(scene);
primaryStage.show();
// Play the media once the stage is shown
player.play();
} // end start
} // end Main Class
要使用 JavaFX,我们需要一个主启动类,它必须扩展 Application
类(自 Java 8 以来 Java 中的标准类)。主启动类的名称是 Main
,它也扩展
了 Application
类。
我们可以说 Main
类是 Application
类的子类。因此,它需要实现所有抽象方法,这就是 Main
类覆盖 start()
方法的原因。
start()
函数接受一个 Stage
类型参数。它是显示 JavaFX 应用程序的所有可视部分的地方。
Stage
类型对象是由 JavaFX 运行时为我们创建的。我们不必手动创建它。
在 start()
方法中,我们获取音频文件的路径并将其保存在 resource
变量中,该变量以字符串格式传递给 Media
构造函数,并进一步传递给 MediaPlayer
构造函数.接下来,我们添加一个 mediaView
来呈现/显示媒体,这是必要的。
然后,这个 mediaView
被添加到 Pane
。我们需要将 Scene
添加到 Stage
对象中,该对象将用于在 JavaFX 应用程序的窗口中显示某些内容。
请记住,需要在 JavaFX 应用程序中显示的所有组件都必须位于 Scene
内。对于这个例子,我们将一个 Scene
对象与媒体视图一起添加到 Stage
。
之后,我们设置标题,设置场景,并在舞台展示后播放媒体。
现在,来到 main
方法。你知道我们可以在没有 main()
函数的情况下启动 JavaFX 应用程序,但是当我们需要将命令行参数传递给应用程序时很有用。
设置 JavaFX 并使用它来制作媒体播放器
要手动安装 JavaFX,我们需要具备以下条件。
- Java 18
- NetBeans 版本 13(你可以使用你选择的任何 IDE)
- 更新
module-info.java
和pom.xml
文件以安装javafx.controls
和javafx.media
(每个文件的完整代码如下所示) - 我们正在使用 Maven 来安装依赖项。你可以使用 Gradle。
示例代码(module-info.java
文件):
module com.mycompany.test {
requires javafx.controls;
requires javafx.media;
exports com.mycompany.test;
}
示例代码(pom.xml
文件):
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="https://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.mycompany</groupId>
<artifactId>Test</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.release>11</maven.compiler.release>
<javafx.version>16</javafx.version>
<javafx.maven.plugin.version>0.0.6</javafx.maven.plugin.version>
</properties>
<dependencies>
<dependency>
<groupId>org.openjfx</groupId>
<artifactId>javafx-controls</artifactId>
<version>${javafx.version}</version>
</dependency>
<dependency>
<groupId>org.openjfx</groupId>
<artifactId>javafx-media</artifactId>
<version>${javafx.version}</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<release>${maven.compiler.release}</release>
</configuration>
</plugin>
<plugin>
<groupId>org.openjfx</groupId>
<artifactId>javafx-maven-plugin</artifactId>
<version>${javafx.maven.plugin.version}</version>
<configuration>
<mainClass>com.mycompany.test.App</mainClass>
</configuration>
</plugin>
</plugins>
</build>
</project>
示例代码(App.java
,主类):
// write the package name (yours may be different)
package com.mycompany.test;
// import necessary libraries
import java.net.URL;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.Pane;
import javafx.scene.media.Media;
import javafx.scene.media.MediaPlayer;
import javafx.scene.media.MediaView;
import javafx.stage.Stage;
/**
* JavaFX App
*/
public class App extends Application {
/**
*
* @param primaryStage
*/
@Override
public void start(Stage primaryStage) {
// path to the file
final URL resource = getClass().getResource("/music/video.mkv");
// create Media Object and pass it the path of the video/audio file
Media mediafile = new Media(resource.toString());
// create MediaPlayer Object and pass the mediafile instance to it
MediaPlayer player = new MediaPlayer(mediafile);
// Add a mediaView, to display the media. Its necessary !
// This mediaView is added to a Pane
MediaView mediaView = new MediaView(player);
// Add to scene
Scene scene = new Scene(new Pane(mediaView), 1080, 750);
// Show the stage
primaryStage.setTitle("Media Player");
primaryStage.setScene(scene);
primaryStage.show();
// Play the media once the stage is shown
player.play();
}
// main method
public static void main(String[] args) {
launch(args);
} // end main method
} // end App class
在 Java 应用程序中,module-info.java
和 pom.xml
文件位于 default package
和 Project Files
中。下面是一个 Java 应用程序中所有文件的截图,以便清楚地了解。
这段代码和上一节使用内置 JavaFX 制作媒体播放器
相同,不同的是我们手动安装 JavaFX 并播放视频文件。