OpenCV画像の作成
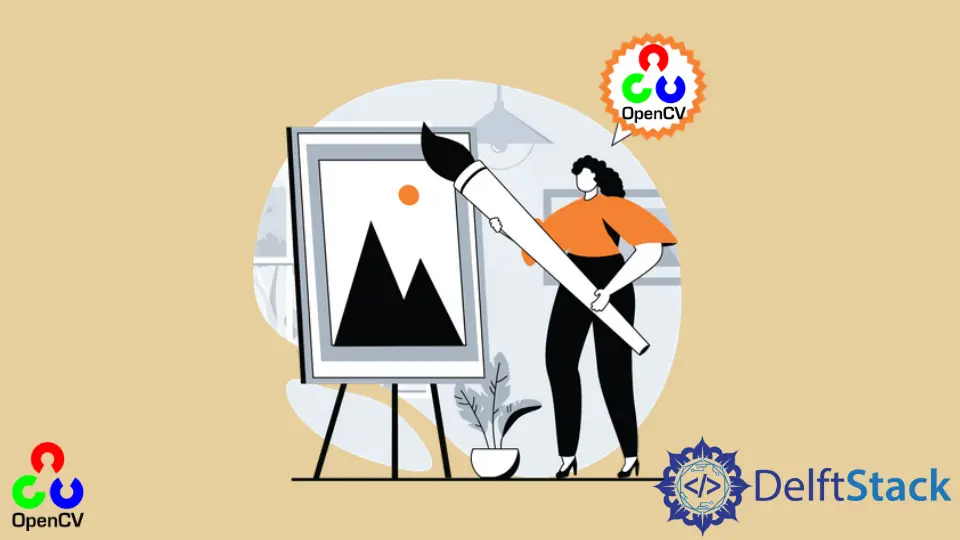
画像処理のタスクの一つは、ゼロから画像を作成するか、既存の画像を読み込んで修正することです。このチュートリアルでは、OpenCVで画像を作成する一般的な方法について説明します。
OpenCVにおいてNumPy
のzeros()
関数を使用した画像の作成
画像は、行列に配置されたピクセルで構成されています。各ピクセルは、BGRの3つ組として表される色の値を含みます。
BGRの3つ組の値は、8ビットの画像では0から255
までの値を取ります。最初の値は青色の強度を表し、2番目と3番目のBRGの値は緑と赤の色に対応します。
これらの3つの色の値を設定することで、任意の色を作ることができます。
8ビットの画像では、1つのピクセルは256
×256
×255
通りの異なる色を持つことができます。私たちはNumPy
のzeros()
関数を使って黒い画像を作ることができます。
zeros()
関数は、行数と列数が与えられた行列を作成します。
サンプルコード1:
import cv2
import numpy as np
# Define the dimensions of the image
height = 512
width = 512
# Create a black image with the specified dimensions
img = np.zeros((height, width, 3), np.uint8)
# Display the image
cv2.imshow("Binary", img)
# Wait until a key is pressed and then close the image window
cv2.waitKey(0)
出力:
上記の画像の各ピクセルはBGRの3つ組の値が(0, 0, 0)
であり、黒い色を表します。各ピクセルの値をBGRの3つ組の値に設定することで、画像の色を変えることもできます。
では、上記の画像の色を青色に変えてみましょう。
サンプルコード2:
import cv2
import numpy as np
# Define the dimensions of the image
height = 512
width = 512
# Create a black image with the specified dimensions
img = np.zeros((height, width, 3), np.uint8)
# Fill the entire image with a blue color
img[:, :] = (255, 0, 0)
# Display the image
cv2.imshow("image", img)
# Wait until a key is pressed and then close the image window
cv2.waitKey(0)
出力:
上記のコードの中のコロン(:)
記号は、全ての行と列に使用されます。最初のコロンは列を、2番目のコロンはimg
行列内の行を表しています。
コロンは0〜100
の範囲を0:100
と定義するような範囲も指定できます。すべてのピクセルの色を設定する代わりに、画像内の一部のピクセルの色を設定することもできます。
例えば、上記の画像の異なる部分に異なる色を設定してみましょう。
サンプルコード3:
import cv2
import numpy as np
# Define the dimensions of the image
height = 512
width = 512
# Create a black image with the specified dimensions
img = np.zeros((height, width, 3), np.uint8)
# Fill the entire image with blue color (BGR format)
img[:, :] = (255, 0, 0)
# Fill a region in the top-left corner with yellow color
img[0:100, 0:100] = (255, 255, 0)
# Fill the right half of the image with red color
img[:, width // 2 : width] = (0, 0, 255)
# Display the resulting image
cv2.imshow("image", img)
# Wait for a key press and close the image window
cv2.waitKey(0)
出力:
上記のコードでは、最初の100
列と最初の100
行の色をシアン色に、画像の右側の色を画像の幅を使用して赤色に設定しています。
OpenCVでの描画関数を使って画像を作成する
OpenCVは、画像に図形要素、線、テキストなどのグラフィカル要素を追加するための様々な描画関数を提供しています。以下の関数を使用することで、形状、線、テキストを含む画像を作成することができます。
-
cv2.line()
: 2点間に直線を描画します。 -
cv2.rectangle()
: 指定した寸法の矩形を描画します。 -
cv2.circle()
: 指定した中心と半径で円を描画します。 -
cv2.ellipse()
: 指定したパラメータで楕円を描画します。 -
cv2.polylines()
: ポリゴンまたは連結された線のシリーズを描画します。 -
cv2.putText()
: 画像にテキストを追加します。
サンプルコード:
import cv2
import numpy as np
# Create a blank image with a white background
width, height = 640, 480
blank_image = np.zeros((height, width, 3), dtype=np.uint8)
blank_image[:] = (255, 255, 255) # White color in BGR format
# Draw a red rectangle
start_point_rect = (100, 100)
end_point_rect = (300, 300)
red_color = (0, 0, 255) # Red color in BGR format
thickness_rect = 2
cv2.rectangle(blank_image, start_point_rect, end_point_rect, red_color, thickness_rect)
# Draw a green circle
center_circle = (400, 150)
radius_circle = 50
green_color = (0, 255, 0) # Green color in BGR format
thickness_circle = -1 # Negative thickness fills the circle
cv2.circle(blank_image, center_circle, radius_circle, green_color, thickness_circle)
# Draw a blue line
start_point_line = (50, 400)
end_point_line = (600, 400)
blue_color = (255, 0, 0) # Blue color in BGR format
thickness_line = 5
cv2.line(blank_image, start_point_line, end_point_line, blue_color, thickness_line)
# Add text to the image
text = "OpenCV Drawing"
font = cv2.FONT_HERSHEY_SIMPLEX
font_scale = 1
font_color = (0, 0, 0) # Black color in BGR format
font_thickness = 2
text_position = (50, 50)
cv2.putText(
blank_image, text, text_position, font, font_scale, font_color, font_thickness
)
# Display the image
cv2.imshow("Image with Drawing", blank_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
出力: