Ajax jQuery で get()メソッドを使用する
Sheeraz Gul
2024年2月15日
jQuery
jQuery Ajax
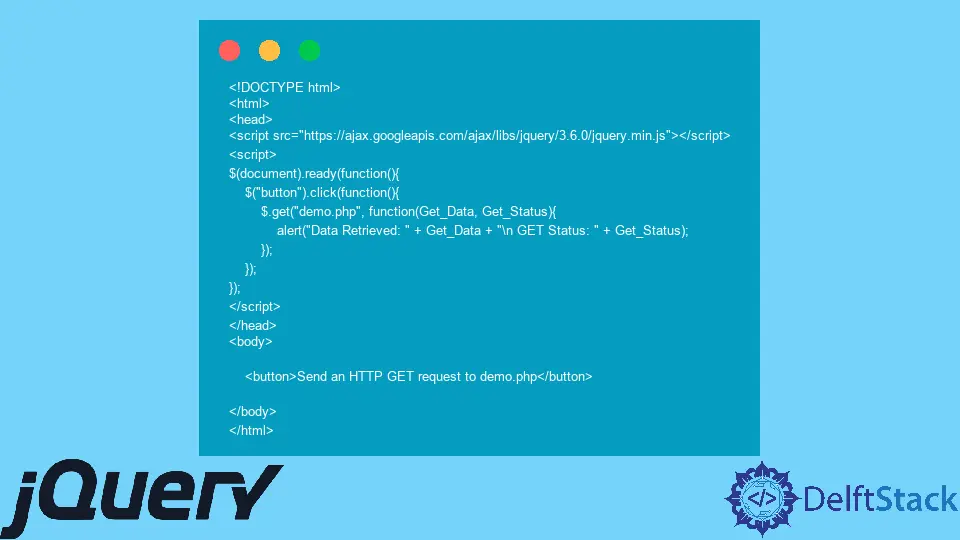
jQuery の get()
メソッドは、データを取得するために非同期 GET 要求をサーバーに送信します。このチュートリアルでは、Ajax jQuery で get()
メソッドを使用する方法を示します。
Ajax jQuery で get()
メソッドを使用する
前述のように、jQuery の get()
は Ajax で使用され、サーバーに GET リクエストを送信します。
構文:
$.get(url, [data],[callback])
url
は、データを取得するリクエスト URL です。data
は GET
リクエストを介してサーバーに送信されるデータであり、callback
はリクエストが成功したときに実行される関数です。
簡単な get()
の例を作成してみましょう。これは demo.php
に移動し、アラートで取得したデータを出力します。
コード-HTML:
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
$.get("demo.php", function(Get_Data, Get_Status){
alert("Data Retrieved: " + Get_Data + "\n GET Status: " + Get_Status);
});
});
});
</script>
</head>
<body>
<button>Send an HTTP GET request to demo.php</button>
</body>
</html>
コード-demo.php
:
<?php
echo "Hello This is GET request data from delftstack.";
?>
出力:
数値の乗算テーブルを表示する get リクエストを送信する別の例を見てみましょう。
コード-HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery Ajax get() Demo</title>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
var Number_Value = $("#input_number").val();
// Send input data to the server using get
$.get("demo.php", {Number: Number_Value} , function(result_data){
// Display the returned data in browser
$("#multiply_result").html(result_data);
});
});
});
</script>
</head>
<body>
<label>Enter a Number: <input type="text" id="input_number"></label>
<button type="button">Press the Button to Show Multiplication Table</button>
<div id="multiply_result"></div>
</body>
</html>
コード-demo.php
:
<?php
$num = htmlspecialchars($_GET["Number"]);
if(is_numeric($num) && $num > 0){
echo "<table>";
for($x=0; $x<11; $x++){
echo "<tr>";
echo "<td>$num x $x</td>";
echo "<td>=</td>";
echo "<td>" . $num * $x . "</td>";
echo "</tr>";
}
echo "</table>";
}
?>
出力:
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
著者: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook