Ajax jQuery와 함께 get() 메소드 사용
Sheeraz Gul
2024년2월15일
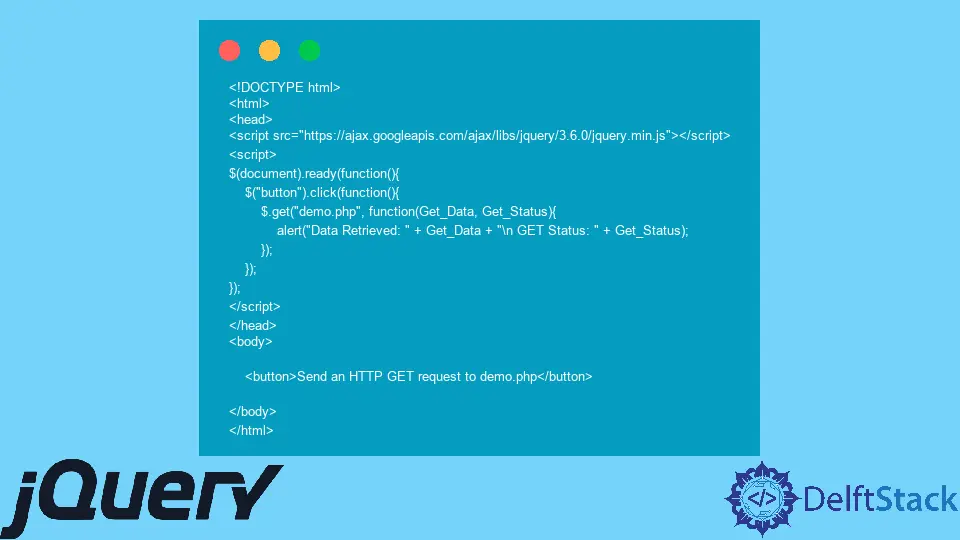
jQuery의 get()
메소드는 데이터를 검색하기 위해 서버에 비동기 GET 요청을 보냅니다. 이 튜토리얼은 Ajax jQuery에서 get()
메소드를 사용하는 방법을 보여줍니다.
Ajax jQuery에서 get()
메소드 사용
위에서 언급했듯이 jQuery의 get()
은 Ajax에서 서버에 GET 요청을 보내는 데 사용됩니다.
통사론:
$.get(url, [data],[callback])
url
은 데이터를 검색할 요청 URL입니다. data
는 GET
요청을 통해 서버로 보낼 데이터이고 callback
은 요청이 성공했을 때 실행될 함수입니다.
간단한 get()
예제를 만들어 demo.php
로 이동하고 경고에서 검색된 데이터를 인쇄해 보겠습니다.
코드 - HTML:
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
$.get("demo.php", function(Get_Data, Get_Status){
alert("Data Retrieved: " + Get_Data + "\n GET Status: " + Get_Status);
});
});
});
</script>
</head>
<body>
<button>Send an HTTP GET request to demo.php</button>
</body>
</html>
코드 - demo.php
는 다음과 같습니다.
<?php
echo "Hello This is GET request data from delftstack.";
?>
출력:
숫자에 대한 곱셈 테이블을 표시하기 위해 get 요청을 보내는 또 다른 예가 있습니다.
코드 - HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery Ajax get() Demo</title>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
var Number_Value = $("#input_number").val();
// Send input data to the server using get
$.get("demo.php", {Number: Number_Value} , function(result_data){
// Display the returned data in browser
$("#multiply_result").html(result_data);
});
});
});
</script>
</head>
<body>
<label>Enter a Number: <input type="text" id="input_number"></label>
<button type="button">Press the Button to Show Multiplication Table</button>
<div id="multiply_result"></div>
</body>
</html>
코드 - demo.php
:
<?php
$num = htmlspecialchars($_GET["Number"]);
if(is_numeric($num) && $num > 0){
echo "<table>";
for($x=0; $x<11; $x++){
echo "<tr>";
echo "<td>$num x $x</td>";
echo "<td>=</td>";
echo "<td>" . $num * $x . "</td>";
echo "</tr>";
}
echo "</table>";
}
?>
출력:
작가: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook