使用 Ajax jQuery 的 get() 方法
Sheeraz Gul
2024年2月15日
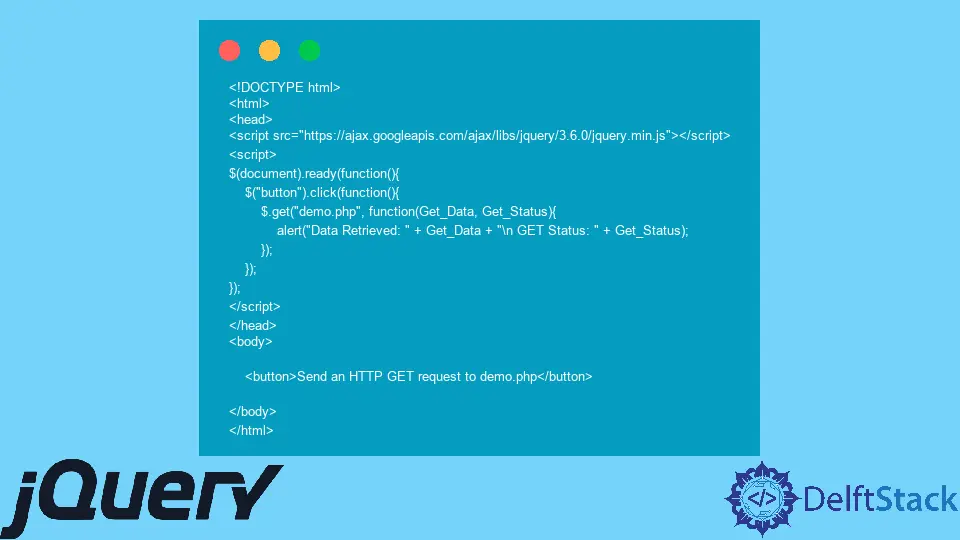
jQuery 中的 get()
方法向伺服器傳送非同步 GET 請求以檢索資料。本教程演示了在 Ajax jQuery 中使用 get()
方法。
在 Ajax jQuery 中使用 get()
方法
如上所述,jQuery 中的 get()
在 Ajax 中用於向伺服器傳送 GET 請求。
語法:
$.get(url, [data],[callback])
url
是我們將從中檢索資料的請求 URL。data
是將通過 GET
請求傳送到伺服器的資料,callback
是將在請求成功時執行的函式。
讓我們建立一個簡單的 get()
示例,它將轉到 demo.php
並列印在警報中檢索到的資料。
程式碼 - HTML:
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
$.get("demo.php", function(Get_Data, Get_Status){
alert("Data Retrieved: " + Get_Data + "\n GET Status: " + Get_Status);
});
});
});
</script>
</head>
<body>
<button>Send an HTTP GET request to demo.php</button>
</body>
</html>
程式碼 - demo.php
是:
<?php
echo "Hello This is GET request data from delftstack.";
?>
輸出:
讓我們再舉一個例子,它會傳送 get 請求來顯示一個數字的乘法表。
程式碼 - HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery Ajax get() Demo</title>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
var Number_Value = $("#input_number").val();
// Send input data to the server using get
$.get("demo.php", {Number: Number_Value} , function(result_data){
// Display the returned data in browser
$("#multiply_result").html(result_data);
});
});
});
</script>
</head>
<body>
<label>Enter a Number: <input type="text" id="input_number"></label>
<button type="button">Press the Button to Show Multiplication Table</button>
<div id="multiply_result"></div>
</body>
</html>
程式碼 - demo.php
:
<?php
$num = htmlspecialchars($_GET["Number"]);
if(is_numeric($num) && $num > 0){
echo "<table>";
for($x=0; $x<11; $x++){
echo "<tr>";
echo "<td>$num x $x</td>";
echo "<td>=</td>";
echo "<td>" . $num * $x . "</td>";
echo "</tr>";
}
echo "</table>";
}
?>
輸出:
作者: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook