Use el método get() con Ajax jQuery
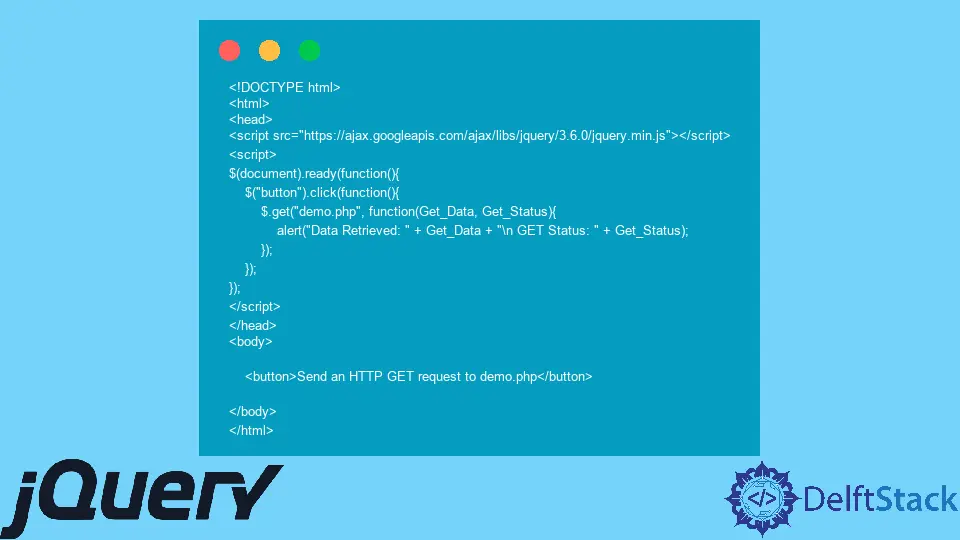
El método get()
en jQuery envía solicitudes GET asíncronas al servidor para recuperar los datos. Este tutorial demuestra el uso del método get()
en Ajax jQuery.
Usa el método get()
en Ajax jQuery
Como se mencionó anteriormente, get()
en jQuery se usa en Ajax para enviar solicitudes GET al servidor.
Sintaxis:
$.get(url, [data],[callback])
La url
es la URL de solicitud desde la que recuperaremos los datos. Los data
son los datos que se enviarán al servidor a través de una solicitud GET
, y la callback
es la función que se ejecutará cuando la solicitud tenga éxito.
Vamos a crear un ejemplo simple de get()
, que irá a demo.php
e imprimirá los datos recuperados en la alerta.
Código - HTML:
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
$.get("demo.php", function(Get_Data, Get_Status){
alert("Data Retrieved: " + Get_Data + "\n GET Status: " + Get_Status);
});
});
});
</script>
</head>
<body>
<button>Send an HTTP GET request to demo.php</button>
</body>
</html>
Código - demo.php
es:
<?php
echo "Hello This is GET request data from delftstack.";
?>
Producción:
Veamos otro ejemplo que enviará la solicitud de obtención para mostrar la tabla de multiplicar de un número.
Código - HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery Ajax get() Demo</title>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
var Number_Value = $("#input_number").val();
// Send input data to the server using get
$.get("demo.php", {Number: Number_Value} , function(result_data){
// Display the returned data in browser
$("#multiply_result").html(result_data);
});
});
});
</script>
</head>
<body>
<label>Enter a Number: <input type="text" id="input_number"></label>
<button type="button">Press the Button to Show Multiplication Table</button>
<div id="multiply_result"></div>
</body>
</html>
Código - demo.php
:
<?php
$num = htmlspecialchars($_GET["Number"]);
if(is_numeric($num) && $num > 0){
echo "<table>";
for($x=0; $x<11; $x++){
echo "<tr>";
echo "<td>$num x $x</td>";
echo "<td>=</td>";
echo "<td>" . $num * $x . "</td>";
echo "</tr>";
}
echo "</table>";
}
?>
Producción:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook