How to Print Output in the Immediate Window in VBA
- Open the Immediate Window in Excel VBA
-
Print Information in the
Immediate Window
in Excel VBA -
Use
Immediate Window
to Ask Information About the Active Workbook in Excel VBA
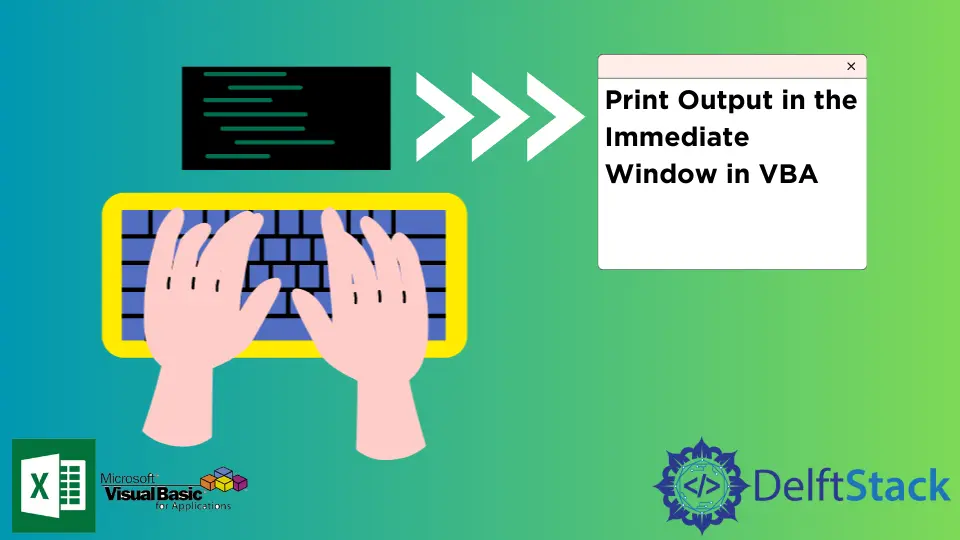
Debugging is one of the most complex parts of developing software in computer programming. The good thing is the creator of VBA allowed us to debug VBA code with ease.
Several debugging tools are at a place like the Immediate Window
, Breakpoints
, Steps
, Variable watchers
, etc., to help the user during the debugging phase of the program development.
Of the several debugging tools, VBA’s Immediate Window
(VBA’s debugging window) is a popular option. It enables the user to print, ask, and run methods and variables while executing the code.
It allows the user to get immediate answers about variables and steps. It is built into the Visual Basic Editor and has different uses, which can be very helpful when debugging the code and displaying the code results.
This tutorial will dive into the use and the benefits of using the Immediate Window
of VBA.
Open the Immediate Window in Excel VBA
Follow these steps below if the Immediate Window
in VBA is not visible in the Visual Basic Editor.
-
Open Excel file.
-
From the
Developer Tab
, open theVisual Basic
editor. -
From the
View
toolbar, clickImmediate Window
. You can also press CTRL+G to show the window.
You can now see the Immediate Window
of Excel VBA.
Print Information in the Immediate Window
in Excel VBA
One of the tool’s uses is its ability to print strings and values. It’s very helpful when it comes to program development. It will allow you to test the code on a more granular level.
Syntax:
Debug.Print [Strings to print]
The code below demonstrates how to print using the Debug.Print
property.
Sub PrintToImmediateWindow()
Debug.Print "This will be printed on the Immediate Window."
End Sub
PrintToImmediateWindow
Output:
Immediate Window:
This will be printed on the Immediate Window.
The code block below will demonstrate printing the value of a variable.
Sub PrintVariableValue(toPrint As String)
Debug.Print (toPrint)
End Sub
Sub testPrint()
Call PrintVariableValue("testPrint123")
End Sub
testPrint
Output:
Immediate Window:
testPrint123
The code block below will print Success!
in the Immediate Window
when a randomly generated number is divisible by 3.
Sub StopWhenDivisible3()
Dim n As Integer
Dim isSuccess As Boolean
Do Until isSuccess = True
n = Int((6 * Rnd) + 1)
Debug.Print n & " is the current number"
If n Mod 3 = 0 Then
Debug.Print "Success!"
isSuccess = True
End If
Loop
End Sub
StopWhenDivisible3
Output:
Immediate Window:
2 is the current number
4 is the current number
4 is the current number
2 is the current number
2 is the current number
5 is the current number
5 is the current number
4 is the current number
6 is the current number
Success!
Use Immediate Window
to Ask Information About the Active Workbook in Excel VBA
One of the Immediate Window
abilities is to return answers based on the current information of the active workbook. The first character in the line should be a question mark ?
to start an ask command.
The below blocks will show how to utilize the Immediate Window
to get information about the active workbook.
To get the number of a worksheet in the active workbook:
Immediate Window
?Worksheets.Count
3
Get the name of the active workbook:
Immediate Window:
?ActiveWorkbook.FullName
Book1
Get the name of the active worksheet:
Immediate Window:
?ActiveSheet.Name
Sheet1