How to Use Refs in React With TypeScript
- The Hooks in React
-
Use Hook
useRef
in React With TypeScript -
Use
React.createRef()
in React With TypeScript
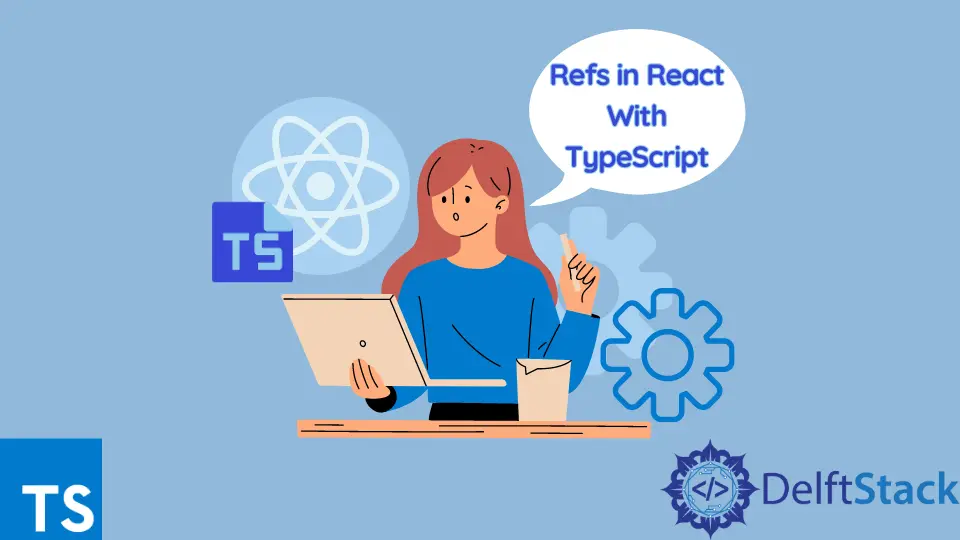
This tutorial provides guidelines about using Refs in React with TypeScript. We will also discuss React Hooks’ purposes and which Hooks help us get the Refs of the element.
First, let’s see what React Hooks are and why they are used.
The Hooks in React
The Hooks are introduced in the React version 16.8
. Hooks allow using state and other features without writing a class, and it does not work inside classes.
Consider the following example where useState()
is utilized, allowing state variables in functional components. The initial state is being passed to the function, and it returns a current state value and the other function to update this value.
Code Sample:
import React, { useState } from 'react';
function Counter() {
// Declare a new state variable, which we'll call "counter"
const [counter, setCounter] = useState(0);
return (
<div>
<p>You clicked {counter} times</p>
<button onClick={() => setCounter(counter + 1)}>
Add
</button>
</div>
);
}
The above code has an initial state variable counter
and a function setCounter
, which sets its value on the button click event. Whenever the button is clicked, the counter
value is increased by one.
Output:
Use Hook useRef
in React With TypeScript
The Hook useRef
allows persisting values between renders. The useRef
stores a mutable value that does not cause a re-render when updated.
useRef
allows access to a DOM element directly. This allows you to directly create a reference to the DOM element in a functional component.
The following example of code in which there is a slider with an image with each having a radio
button to control its motion, of which we need a reference, so we use the React Hook called useRef
, which creates the reference of those radio
buttons which are controlled in the function.
Code - React(App):
import React from "react";
import "./slider.css";
import Cover from "../src/assets/back.png";
import {useRef} from "react";
const Slider = () => {
const input1 = useRef();
const input2 = useRef();
const input3 = useRef();
var counter = 1;
setInterval(function () {
const myInput = eval("input"+counter).current;
if(myInput){
myInput.checked = true;
}
counter++;
if (counter > 3) {
counter = 1;
}
}, 4000);
return (
<div className="slider">
<div className="slides">
<input type="radio" name="radio-btn" id="radio1" ref={input1}/>
<input type="radio" name="radio-btn" id="radio2" ref={input2}/>
<input type="radio" name="radio-btn" id="radio3" ref={input3}/>
<div className="slide first">
<img src={Cover} alt="" />
</div>
<div className="slide">
<img src={Cover} alt="" />
</div>
<div className="slide">
<img src={Cover} alt="" />
</div>
</div>
<div className="navigation-manual flex center-1">
<label htmlFor="radio1" className="manual-btn"></label>
<label htmlFor="radio2" className="manual-btn"></label>
<label htmlFor="radio3" className="manual-btn"></label>
</div>
</div>
);
};
export default Slider;
Code - CSS:
.slider{
position: relative;
border-radius: 5px;
height: 250px;
border-radius: 10px;
overflow: hidden;
margin: 30px auto;
}
.slides{
width: 500%;
height: 280px;
display: flex;
}
.slides input{
display: none;
}
.slide{
width: 20%;
transition: 2s;
}
.slide img{
width: 100%;
height: 100%;
}
/*css for manual slide navigation*/
.navigation-manual{
position: absolute;
width: 100%;
bottom: 20px;
display: flex;
justify-content: center;
}
.manual-btn{
border: 2px solid #40D3DC;
padding: 5px;
border-radius: 10px;
cursor: pointer;
transition: 1s;
}
.manual-btn:not(:last-child){
margin-right: 40px;
}
.manual-btn:hover{
background: #40D3DC;
}
#radio1:checked ~ .first{
margin-left: 0;
}
#radio2:checked ~ .first{
margin-left: -20%;
}
#radio3:checked ~ .first{
margin-left: -40%;
}
#radio4:checked ~ .first{
margin-left: -60%;
}
/*css for automatic navigation*/
.navigation-auto{
position: absolute;
display: flex;
width: 800px;
justify-content: center;
}
.navigation-auto div{
border: 2px solid #40D3DC;
padding: 5px;
border-radius: 10px;
transition: 1s;
}
.navigation-auto div:not(:last-child){
margin-right: 40px;
}
#radio1:checked ~ .navigation-auto .auto-btn1{
background: #40D3DC;
}
#radio2:checked ~ .navigation-auto .auto-btn2{
background: #40D3DC;
}
#radio3:checked ~ .navigation-auto .auto-btn3{
background: #40D3DC;
}
#radio4:checked ~ .navigation-auto .auto-btn4{
background: #40D3DC;
}
Code - React(Main):
import './App.css';
import Slider from './Slider';
function App() {
return (
<div className="App">
<Slider/>
</div>
);
}
export default App;
The other file is the .css
file used for styling the slider. The above component will look like this after rendering:
Use React.createRef()
in React With TypeScript
In the class component for getting the reference of the input field, we use the createRef()
method, which is used to access any DOM element in a component and returns a mutable ref object.
In the following example, the field gets focused when the button is clicked.
Code:
class CustomTextInput extends React.Component {
constructor(props) {
super(props);
// creates a ref to store the element textInput DOM
this.textInput = React.createRef();
this.focusTextInput = this.focusTextInput.bind(this);
}
focusTextInput() {
//Focus the input text using the raw DOM API
// Note: we access "current" to get the node DOM
this.textInput.current.focus();
}
render() {
// tell React, we want to associate the <input> ref
// with `textInput` that was created in the constructor
return (
<div>
<input
type="text"
ref={this.textInput} />
<input
type="button"
value="Focus the text input"
onClick={this.focusTextInput}
/>
</div>
);
}
}
Output:
Ibrahim is a Full Stack developer working as a Software Engineer in a reputable international organization. He has work experience in technologies stack like MERN and Spring Boot. He is an enthusiastic JavaScript lover who loves to provide and share research-based solutions to problems. He loves problem-solving and loves to write solutions of those problems with implemented solutions.
LinkedIn