How to Use the Infer Keyword in TypeScript
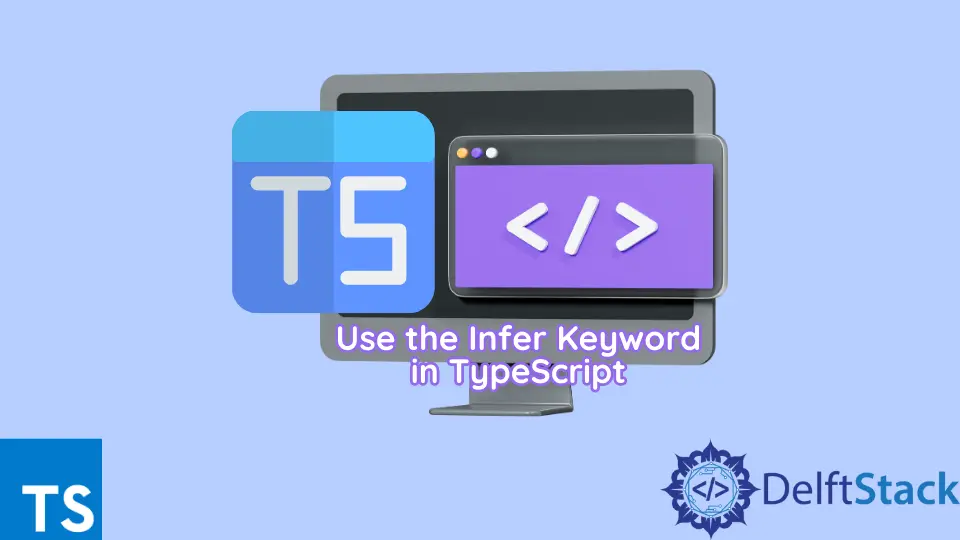
This article explains and demonstrates the type inference and implementation of conditional types in TypeScript. This discusses the importance of the infer keyword and how to use it.
Type Inference in TypeScript
TypeScript is known as a typed superset of the JavaScript programming language. Therefore, TypeScript users can explicitly annotate the types as shown in the following.
Variables:
let numberTypeVariable: number = 2;
let stringTypeVariable: string = 'This is a string';
let booleanTypeVariable: boolean = true;
Function Parameters:
function addTwoNumbers(numberOne: number, numberTwo: number){
return numberOne + numberTwo;
}
Function Return Types:
function addTwoNumbers(numberOne: number, numberTwo: number): number{
return numberOne + numberTwo;
}
This makes TypeScript a type-safe language, and programmers can detect errors at compile time. Also, there is no need to explicitly annotate your variables, function parameters, or return types.
Since TypeScript is a typed language, the TypeScript compiler is intelligent enough to find the correct types implicitly. This is called Type Inference.
TypeScript uses the Best Common Type algorithm to select the best possible type compatible with all the candidates.
Example:
let stringValueInferred = 'This is a string literal';
Output (in Visual Studio Code):
Since the stringValueInferred
variable has been assigned with a string literal, the TypeScript compiler infers its types as a string.
When you assign the function parameters with default values, the TypeScript compiler also infers the type.
Example:
function addTwoNums(numOne=0, numTwo=0){
return numOne + numTwo
}
Output:
Also, you do not need to annotate the function return type explicitly. It has been inferred too.
The addTwoNums
function return type has been inferred as a number
type. This is a very robust feature that TypeScript offers out of the box.
Implement Conditional Types in TypeScript
The conditional type is another powerful feature of the type inference. TypeScript can select one of two possible types based on a specified condition.
Syntax:
type myPreferredType = T extends U ? X : Y
The meaning of the above syntax is that if some type T
is assignable to some type U
, choose true path type X
; else, choose type Y
.
Now, let’s create our type called myOwnType
.
type myOwnType = number;
Then, we will create a conditional type called myOwnConditionalType
.
type myOwnConditionalType = myOwnType extends number? number : any;
Output:
The myOwnConditionalType
is inferred as a number. This is because the myOwnType
is a number type, and it is assignable to the number type in the condition given: number ? number : any
.
If we change the myOwnType
to string. Then, the myOwnConditionalType
should become any
type.
Output:
The same concept is valid with complex generic types too. Let’s take a look at a generic type.
type myStringOrNullType<T> = T extends string ? string : null;
We will use this myStringOrNullType<T>
type to infer another custom type.
type myInferredType = myStringOrNullType<boolean>;
Output:
The myInferredType
has been inferred as null
because the boolean is not assignable to a string type, and the given condition follows the false path, which returns null.
Output:
Since T
is now a string literal, it is assignable to string type. Therefore, the condition evaluates to true, and that path returns the string type.
Use the infer
Keyword in TypeScript
The infer
keyword makes conditional type used more flexibly. It allows users to infer some type as a whole or a part of that type which is very powerful in some cases.
Let’s define a new type with the infer
keyword.
type inferSomeType<T> = T extends infer U ? U : null;
In this case, we will infer the whole T
type and return it.
type myInferredType = inferSomeType<'This is going to be inferred as the type'>
Output:
Now, the myInferredType
type has been inferred as "This is going to be inferred as the type"
coming from the infer U
part. That means we have inferred the whole type of T
.
Now, let’s focus on another example where we can infer parts of the type T
.
type inferPartsOfSomeType<T> = T extends { propA: infer TypeA, propB: number } ? TypeA : null;
Here, we check whether the type T
is assignable to a given object type. If that object is the same as the T
, then the condition evaluates to true and returns the inferred typeA
; otherwise, it returns null
.
Let’s create a new custom type called inferredType
using the above-declared type inferPartsOfSomeType
.
type inferredType = inferPartsOfSomeType<{ propA: 'NewTypeA' }>
In the above case, T
is not assignable to the given object { prop1: 'NewTypeA' }
because another number type property is missing within this object. So, the condition should evaluate to false and returns null
as the inferredType
value.
Output:
Let’s give an identical object to the defined object in inferPartsOfSomeType
- something like this.
{ propA: NewTypeA, propB: 500 }
Output:
This time the new object { propA: NewTypeA, propB: 500 }
is assignable to type T
; hence, the condition evaluates to true, and it infers the type of the propA
, which is "NewTypeA"
. This summarizes how you can infer parts of the type T
.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.