The implements Keyword in TypeScript
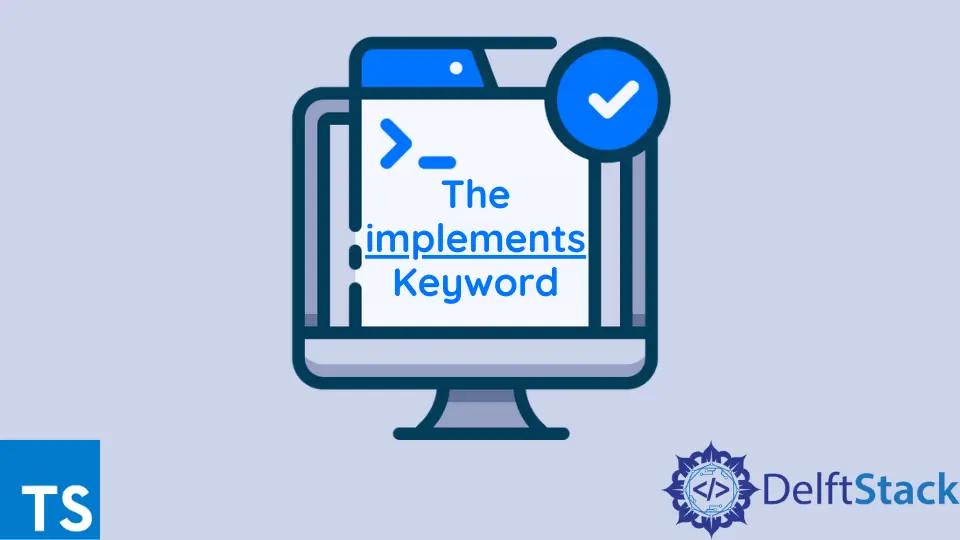
This article explains the TypeScript implements
keyword.
TypeScript Type Checking
TypeScript is a superset of JavaScript which includes type checking at compile time. The compile-time type checking mainly focuses on whether a variable or argument value has the shape it’s supposed to be.
This technique is called duck typing in TypeScript. As a result of duck typing, the TypeScript compiler checks whether the two objects are identical.
It ensures the type safety in a TypeScript program.
The type checking checks whether two given objects contain the same properties and methods. If not, the TypeScript compiler will throw an error.
Let’s assume that we have three TypeScript classes, as shown in the following.
class Person {
gender: string;
}
class Employee {
gender: string;
empId: string;
}
class Manager {
gender: string;
empId: string;
}
Let’s create new Person
and Employee
objects.
let person1: Person = new Person();
let employee1: Employee = new Employee();
This code will be successfully compiled because it doesn’t violate type-checking rules.
Let’s try to assign the employee1
object to a Person
type variable.
let person2: Person = employee1;
It has been compiled successfully because the employee1
object has the Person
object shape. Since the Person
objects should have only one property called gender
, it complies with the employee1
object.
The additional empId
property is totally fine.
Let’s try to assign the person1
object to the Employee
type variable as shown in the following.
let employee2: Employee = person1;
TypeScript duck typing rules do not permit this. Hence, the TypeScript compiler raises an error.
Usually, the TypeScript interfaces play the role of defining types and contracts in TypeScript code.
TypeScript Interfaces
TypeScript interfaces provide the shape for your classes. Whenever a TypeScript class derives from an interface, it must implement the structure defined by the interface.
It is like a real-world contract; as long as a class accepts the interface, it should adhere to the interface structure. Interfaces do not implement any business logic.
It just declares the properties and methods as shown in the following.
interface IVehicle {
vehicleNumber: string;
vehicleBrand: string;
vehicleCapacity: number;
getTheCurrentGear: () => number;
getFuelLevel(): string;
}
The TypeScript interfaces can be used as a type, function type, array type, etc. It can be used as a contract for a class, as discussed in the following section.
the implements
Clause in TypeScript
The TypeScript implements
keyword is used to implement an interface within a class. The IVehicle
interface doesn’t contain any implementation-level details.
Hence, the IVehicle
interface can be implemented by a new VipVehicle
class.
class VipVehicle implements IVehicle {
}
The VipVehicle
class comes to a contract with the IVehicle
interface, as shown in the above example. If you compile this TypeScript code, it will raise an error, as shown in the following.
TypeScript compiler complains that three properties and two methods have not been implemented within the VipVehicle
class. Hence, it is mandatory to conform to the structure of the IVehicle
interface.
We should add three properties and implement the two methods accordingly.
class VipVehicle implements IVehicle {
vehicleNumber: string;
vehicleBrand: string;
vehicleCapacity: number;
getTheCurrentGear():number {
return 3; // dummy numebr returning here for demo purposes
}
getFuelLevel():string {
return 'full'; // dummy string returning here for demo purposes
}
}
If we compile the code again, it should compile successfully.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.