在 TypeScript 中的 Implements 關鍵字
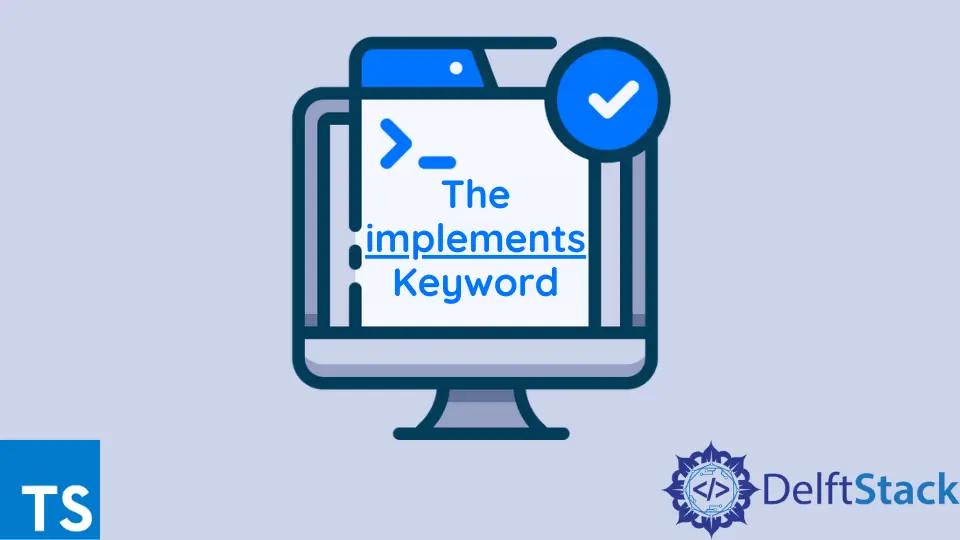
本文解釋了 TypeScript implements
關鍵字。
TypeScript 型別檢查
TypeScript 是 JavaScript 的超集,其中包括編譯時的型別檢查。編譯時型別檢查主要關注變數或引數值是否具有應有的形狀。
這種技術在 TypeScript 中稱為鴨子型別。作為鴨子型別的結果,TypeScript 編譯器檢查兩個物件是否相同。
它確保了 TypeScript 程式中的型別安全。
型別檢查檢查兩個給定物件是否包含相同的屬性和方法。如果沒有,TypeScript 編譯器會丟擲錯誤。
假設我們有三個 TypeScript 類,如下所示。
class Person {
gender: string;
}
class Employee {
gender: string;
empId: string;
}
class Manager {
gender: string;
empId: string;
}
讓我們建立新的 Person
和 Employee
物件。
let person1: Person = new Person();
let employee1: Employee = new Employee();
此程式碼將成功編譯,因為它不違反型別檢查規則。
讓我們嘗試將 employee1
物件分配給 Person
型別的變數。
let person2: Person = employee1;
它已成功編譯,因為 employee1
物件具有 Person
物件形狀。因為 Person
物件應該只有一個名為 gender
的屬性,所以它符合 employee1
物件。
額外的 empId
屬性完全沒問題。
讓我們嘗試將 person1
物件分配給 Employee
型別變數,如下所示。
let employee2: Employee = person1;
TypeScript 鴨子型別規則不允許這樣做。因此,TypeScript 編譯器會引發錯誤。
通常,TypeScript 介面在 TypeScript 程式碼中扮演定義型別和協定的角色。
TypeScript 介面
TypeScript 介面為你的類提供了形狀。每當 TypeScript 類派生自介面時,它必須實現介面定義的結構。
這就像一個現實世界的合同;只要一個類接受介面,它就應該遵守介面結構。介面不實現任何業務邏輯。
它只是宣告屬性和方法,如下所示。
interface IVehicle {
vehicleNumber: string;
vehicleBrand: string;
vehicleCapacity: number;
getTheCurrentGear: () => number;
getFuelLevel(): string;
}
TypeScript 介面可以用作型別、函式型別、陣列型別等。它可以用作類的契約,如下一節所述。
TypeScript 中的 implements
子句
TypeScript implements
關鍵字用於在類中實現介面。IVehicle
介面不包含任何實現級別的詳細資訊。
因此,IVehicle
介面可以由新的 VipVehicle
類實現。
class VipVehicle implements IVehicle {
}
VipVehicle
類與 IVehicle
介面達成契約,如上例所示。如果編譯此 TypeScript 程式碼,它將引發錯誤,如下所示。
TypeScript 編譯器抱怨在 VipVehicle
類中沒有實現三個屬性和兩個方法。因此,必須符合 IVehicle
介面的結構。
我們應該新增三個屬性並相應地實現這兩個方法。
class VipVehicle implements IVehicle {
vehicleNumber: string;
vehicleBrand: string;
vehicleCapacity: number;
getTheCurrentGear():number {
return 3; // dummy numebr returning here for demo purposes
}
getFuelLevel():string {
return 'full'; // dummy string returning here for demo purposes
}
}
如果我們再次編譯程式碼,它應該編譯成功。
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.