TypeScript fetch() Method
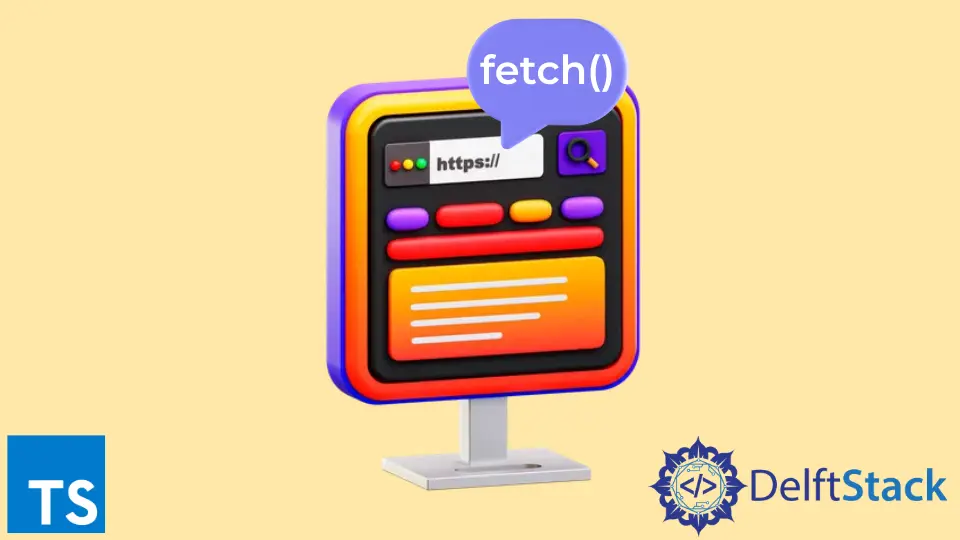
The fetch
is a globally available native browser function that can fetch resources over an HTTP connection. In TypeScript, we can use the fetch
function to consume typed response data.
the fetch()
Method in TypeScript
The Web API offers a global fetch
method via Window
and WorkerGlobalScope
. Those two interfaces implement the WindowOrWorkerGlobalScope
, where the fetch
method has been defined.
Therefore, the fetch
method can be identified as a native browser function to fetch resources over a network. Normally, the fetch
method returns a promise.
Therefore, you need to use then
handlers to handle the HTTP response. The fetch
method response differs from the old Jquery.ajax()
.
Because the promise will only reject due to a network failure or a permission issue, it doesn’t reject HTTP errors like 404
or 500
.
The following shows the syntax of the fetch
method.
fetch(resource, [,custom_settings_per_request]);
resource
- This is the resource you will fetch from a network. It should be a string; basically, the URL of the resource.custom_settings_per_request
- This is an optional parameter. You can pass custom settings for a given HTTP request, such as HTTP method, headers, body, etc.
Let’s use a dummy HTTP endpoint that retrieves an array of ToDo
objects. The following shows how to make a fetch
call.
fetch("https://jsonplaceholder.typicode.com/todos");
This request returns a response that resolves to a Response
object. Therefore, we need to use then
handlers to retrieve the data.
With the json()
method, let’s manipulate the response body.
fetch("https://jsonplaceholder.typicode.com/todos")
.then(
(response) => response.json()
);
This will return another promise with response body data. Therefore, as shown in the following, we can use another then
handler to access the real data.
fetch("https://jsonplaceholder.typicode.com/todos")
.then(
(response) => response.json()
)
.then(
(toDoListArray) => console.log(toDoListArray)
);
Output:
the Strongly-Typed Fetch Response in TypeScript
The drawback of fetch()
is it’s not a generic function, and it is hard to consume typed response data. Therefore, it is good to have a wrapper in TypeScript.
Let’s use the same dummy HTTP endpoint to fetch one ToDo
item. The returned response
object would look like the following.
{
"userId": 1,
"id": 2,
"title": "quis ut nam facilis et officia qui",
"completed": false
}
Let’s create a Todo
type to handle the fetched response
object.
// Todo type interface
interface Todo {
userId: number;
id: number;
title: string;
completed: boolean;
}
We will be creating a new function that handles the fetch
method with a generic type response.
function fetchToDo<T>(resourceUrl: string): Promise<T> {
return fetch(resourceUrl).then(response => {
// fetching the reponse body data
return response.json<T>()
})
}
This function can be called to consume the data, and now we are getting a typed Todo
object as the response. It can be assigned to the Todo
type variable directly.
// Consuming the fetchToDo to retrieve a Todo
fetchToDo<Todo>("https://jsonplaceholder.typicode.com/todos/2")
.then((toDoItem) => {
// assigning the response data `toDoItem` directly to `myNewToDo` variable which is
// of Todo type
let myNewToDo:Todo = toDoItem;
// It is possible to access Todo object attributes easily
console.log('\n id: '+ myNewToDo.id + '\n title: ' + myNewToDo.title + '\n completed: ' + myNewToDo.completed + '\n User Id: ' + myNewToDo.userId);
});
Output:
"id: 2
title: quis ut nam facilis et officia qui
completed: false
User Id: 1"
This methodology can be used for any HTTP method like POST
, DELETE
, etc.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.