TypeScript에서 가져오기
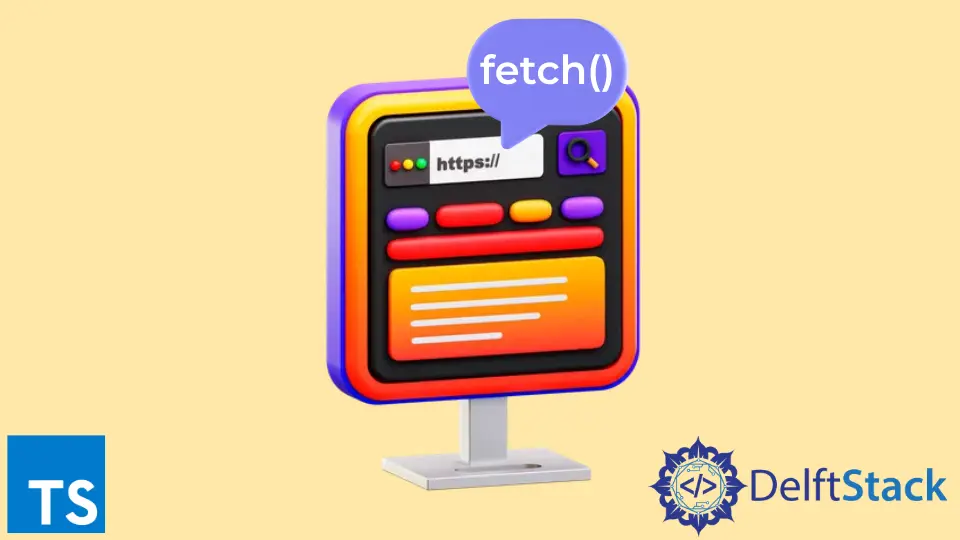
가져오기
는 HTTP 연결을 통해 리소스를 가져올 수 있는 전역적으로 사용 가능한 기본 브라우저 기능입니다. TypeScript에서는 fetch
기능을 사용하여 입력된 응답 데이터를 사용할 수 있습니다.
TypeScript의 fetch()
메서드
Web API는 Window
및 WorkerGlobalScope
를 통해 글로벌 fetch
메서드를 제공합니다. 이 두 인터페이스는 fetch
메서드가 정의된 WindowOrWorkerGlobalScope
를 구현합니다.
따라서 fetch
메서드는 네트워크를 통해 리소스를 가져오는 기본 브라우저 기능으로 식별할 수 있습니다. 일반적으로 fetch
메서드는 약속을 반환합니다.
따라서 HTTP 응답을 처리하려면 then
핸들러를 사용해야 합니다. fetch
메서드 응답은 기존 Jquery.ajax()
와 다릅니다.
Promise는 네트워크 오류 또는 권한 문제로 인해 거부되기 때문에 404
또는 500
과 같은 HTTP 오류는 거부하지 않습니다.
다음은 fetch
메서드의 구문을 보여줍니다.
fetch(resource, [,custom_settings_per_request]);
리소스
- 네트워크에서 가져올 리소스입니다. 문자열이어야 합니다. 기본적으로 리소스의 URL입니다.custom_settings_per_request
- 선택적 매개변수입니다. HTTP 메서드, 헤더, 본문 등과 같은 지정된 HTTP 요청에 대한 사용자 지정 설정을 전달할 수 있습니다.
ToDo
개체의 배열을 검색하는 더미 HTTP 끝점을 사용해 봅시다. 다음은 fetch
호출을 수행하는 방법을 보여줍니다.
fetch("https://jsonplaceholder.typicode.com/todos");
이 요청은 Response
개체로 확인되는 응답을 반환합니다. 따라서 then
핸들러를 사용하여 데이터를 검색해야 합니다.
json()
메서드를 사용하여 응답 본문을 조작해 보겠습니다.
fetch("https://jsonplaceholder.typicode.com/todos")
.then(
(response) => response.json()
);
그러면 응답 본문 데이터가 포함된 또 다른 약속이 반환됩니다. 따라서 다음과 같이 다른 then
핸들러를 사용하여 실제 데이터에 액세스할 수 있습니다.
fetch("https://jsonplaceholder.typicode.com/todos")
.then(
(response) => response.json()
)
.then(
(toDoListArray) => console.log(toDoListArray)
);
출력:
TypeScript의 강력한 형식의 Fetch 응답
fetch()
의 단점은 일반 함수가 아니며 형식화된 응답 데이터를 사용하기 어렵다는 것입니다. 따라서 TypeScript에 래퍼가 있는 것이 좋습니다.
동일한 더미 HTTP 엔드포인트를 사용하여 하나의 ToDo
항목을 가져오겠습니다. 반환된 response
개체는 다음과 같습니다.
{
"userId": 1,
"id": 2,
"title": "quis ut nam facilis et officia qui",
"completed": false
}
가져온 response
개체를 처리하기 위해 Todo
유형을 만들어 봅시다.
// Todo type interface
interface Todo {
userId: number;
id: number;
title: string;
completed: boolean;
}
일반 유형 응답으로 fetch
메서드를 처리하는 새 함수를 만들 것입니다.
function fetchToDo<T>(resourceUrl: string): Promise<T> {
return fetch(resourceUrl).then(response => {
// fetching the reponse body data
return response.json<T>()
})
}
이 함수는 데이터를 사용하기 위해 호출할 수 있으며 이제 응답으로 유형이 지정된 Todo
개체를 받고 있습니다. Todo
유형 변수에 직접 할당할 수 있습니다.
// Consuming the fetchToDo to retrieve a Todo
fetchToDo<Todo>("https://jsonplaceholder.typicode.com/todos/2")
.then((toDoItem) => {
// assigning the response data `toDoItem` directly to `myNewToDo` variable which is
// of Todo type
let myNewToDo:Todo = toDoItem;
// It is possible to access Todo object attributes easily
console.log('\n id: '+ myNewToDo.id + '\n title: ' + myNewToDo.title + '\n completed: ' + myNewToDo.completed + '\n User Id: ' + myNewToDo.userId);
});
출력:
"id: 2
title: quis ut nam facilis et officia qui
completed: false
User Id: 1"
이 방법론은 POST
, DELETE
등과 같은 모든 HTTP 메소드에 사용할 수 있습니다.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.