How to Return a Promise in TypeScript
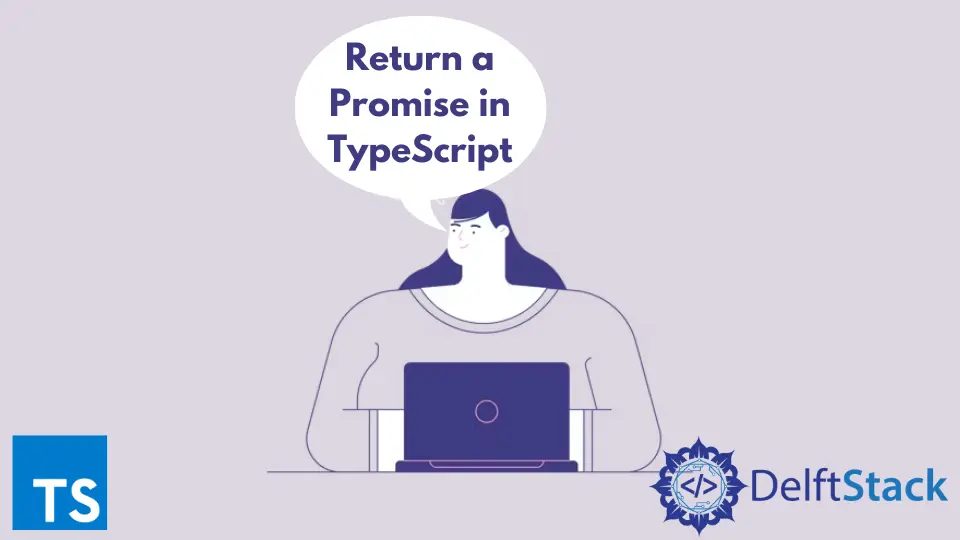
This tutorial will discuss returning a proper Promise in TypeScript with coding examples and outputs. Let’s first see what Promises are and why they are being used.
Promise in TypeScript
The Promise in TypeScript performs asynchronous programming to execute several tasks simultaneously. We can use it when handling numerous jobs at once.
We can skip the current operation and go to the following line of code by using the Promise.
If a function returns a Promise, it means that the result of the function call is not available. And we can not access the real output when it is available using the Promise return by the function.
A promise can be one of the three states below.
- The first state is called the Pending state. When a Promise is created, it will be pending.
- The second is called the Resolved state, in which the Promise is successfully executed
- If any error occurs in the Promise, it will move to the third state called Rejected.
Syntax:
new promise(function(resolve,reject){
//our logic goes here
});
Return a Promise in TypeScript
Multiple parallel calls are handled using Promise. The key advantage of using it is that we may move on to the following line of code without executing the last line.
This also aids in improving the application’s performance.
Let us look at the different outcomes of Promise.
Reject and Resolve in Promise
To manage a Promise’s error response, we must reject the parameter we supply inside the callback function. This rejects parameter will handle the error using the catch()
block.
Syntax:
function mypromise() { var promise = new Promise((resolve, reject) =>
{
// our logic goes here ..
reject();
}
mypromise().then(function(success)
{
// success logic will go here
})
.catch(function(error) { // logic goes here // });
We can also handle the Promise
function’s success response with resolve()
and a successful callback.
Syntax:
function demo()
{ var promise = new Promise((resolve, reject) => {
// logic will go here ..
resolve();
}
demo().then( () => // logic goes here .. );
To better understand the flow of Promise in TypeScript, let’s look at the brief code example.
The Promise will either use .then()
if it gets resolved or .catch()
if it gets rejected.
const promiseTypeScript = new Promise((resolve, reject) => {
resolve("abc");
});
promiseTypeScript.then((res) => {
console.log('I get called:', res === 123); // I get called: true
});
promiseTypeScript.catch((err) => {
// This is never called
});
In the above example, the resolve()
part of the Promise is called and returns a boolean value, whereas the reject()
part is not. Thus, this Promise will always be resolved.
And since the resolve()
part of the Promise is being called, it will execute .then()
.
Output:
The Promise’s reject()
part is called in the following code since it always returns an error. So, .catch()
will be executed.
const promiseReturn = new Promise((resolve, reject) => {
reject(new Error("Something awful happened"));
});
promiseReturn.then((res) => {
// This is never called
});
promiseReturn.catch((err) => {
console.log('I get called:', err.message); // I get called: 'Something awful happened'
});
Output:
In TypeScript, promise chain-ability is the heart of promises’ benefits. Use the .then()
function to create a chain of promises.
Promise.resolve(123)
.then((res) => {
console.log(res); // 123
return 456;
})
.then((res) => {
console.log(res); // 456
return Promise.resolve(123); // Notice that we are returning a Promise
})
.then((res) => {
console.log(res); // 123 : Notice that this `then` is called with the resolved value
return 123;
})
Output:
A single .catch()
can be aggregated for the error handling of any preceding portion of the chain.
Promise.reject(new Error('something bad happened'))
.then((res) => {
console.log(res); // not called
return 456;
})
.then((res) => {
console.log(res); // not called
return 123;
})
.then((res) => {
console.log(res); // not called
return 123;
})
.catch((err) => {
console.log(err.message); // something bad happened
});
Output:
Summary
- Promise is used to make asynchronous calls.
- Keep in mind that you can only call from tasks that aren’t interdependent. Otherwise, there will be a data inconsistency problem.
- When using it, you must pass the inner function; otherwise, you’ll get an error.
Ibrahim is a Full Stack developer working as a Software Engineer in a reputable international organization. He has work experience in technologies stack like MERN and Spring Boot. He is an enthusiastic JavaScript lover who loves to provide and share research-based solutions to problems. He loves problem-solving and loves to write solutions of those problems with implemented solutions.
LinkedIn