How to Parse JSON Strings in TypeScript
-
Use
JSON.parse()
to Parse JSON Strings Into Objects in TypeScript - Use Guards for Safe Conversion From JSON String to Object in TypeScript
-
Use the
Partial
Keyword to Avoid Runtime Errors in TypeScript
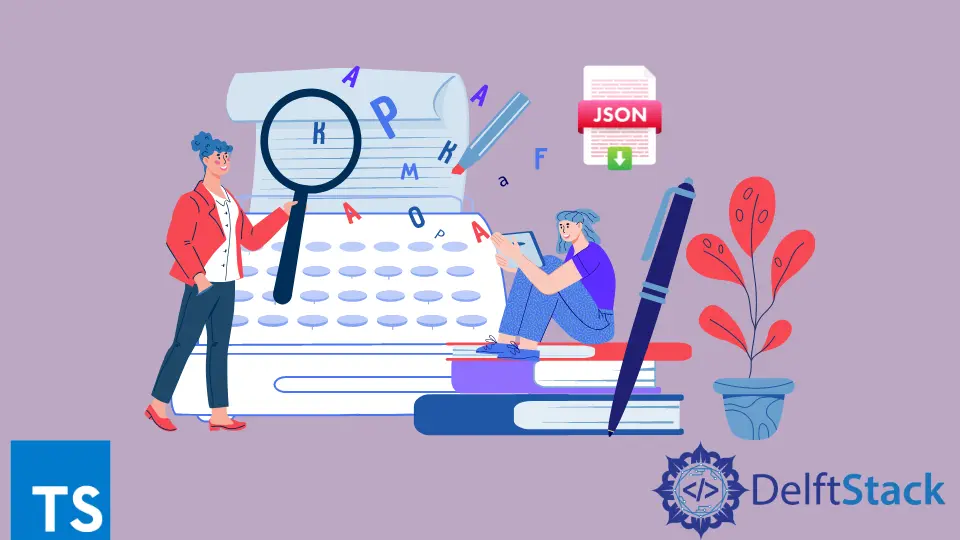
Strings are a means of communication over the internet, and all the data is transferred in a very popular format known as JSON. This JSON representation of the data often represents an object or even a class in TypeScript.
This tutorial will focus on how to safely parse back the JSON string into a TypeScript object.
Use JSON.parse()
to Parse JSON Strings Into Objects in TypeScript
The JSON.parse()
function used in JavaScript can also be used in TypeScript as TypeScript is a superset of JavaScript. However, the following method assumes that the JSON string to be converted to the object has all the required attributes associated with the object.
Example Code:
interface Person {
name : string;
age : number;
}
const personString = `{"name" : "david", "age" : 20}`;
const personObj : Person = JSON.parse(personString);
console.log(personObj);
Output:
{
"name": "david",
"age": 20
}
Use Guards for Safe Conversion From JSON String to Object in TypeScript
User-defined guards can check if the JSON string contains all or some of the properties required and thus process it further according to immediate needs or reject it.
Example Code:
interface Person {
name : string;
age : number;
}
function checkPersonType( obj : any ) : obj is Person {
return 'name' in obj && 'age' in obj;
}
const personString1 = `{"name" : "david"}`;
const personString2 = `{"name" : "david", "age" : 20 }`;
const personObj1 : Person = JSON.parse(personString1);
if ( checkPersonType(personObj1)) {
console.log(personObj1);
} else {
console.log(personString1 + ' cannot be parsed');
}
const personObj2 : Person = JSON.parse(personString2);
if ( checkPersonType(personObj2)) {
console.log(personObj2);
} else {
console.log(personString2 + ' cannot be parsed');
}
Output:
"{"name" : "david"} cannot be parsed"
{
"name": "david",
"age": 20
}
Use the Partial
Keyword to Avoid Runtime Errors in TypeScript
Some fields may be absent in the JSON string. In that case, the attributes in the object can be filled with some default values.
The Partial
keyword in TypeScript helps us achieve this by making all the attributes in the object optional and thus can be chained with a null check operator to set default values.
Example Code:
interface Person {
name : string;
age : number;
}
const personString = `{"name" : "david"}`;
const personObj : Partial<Person> = JSON.parse(personString);
console.log(personObj);
console.log( personObj.age ?? 30);
Output:
{
"name": "david"
}
30
Thus although the JSON string doesn’t have the age
attribute as required by the Person
interface, the null check or ??
operator is used to have a default value for the age
attribute.