在 TypeScript 中解析 JSON 字符串
Shuvayan Ghosh Dastidar
2023年1月30日
TypeScript
TypeScript JSON
-
使用
JSON.parse()
将 JSON 字符串解析为 TypeScript 中的对象 - 在 TypeScript 中使用防护将 JSON 字符串安全转换为对象
-
在 TypeScript 中使用
Partial
关键字避免运行时错误
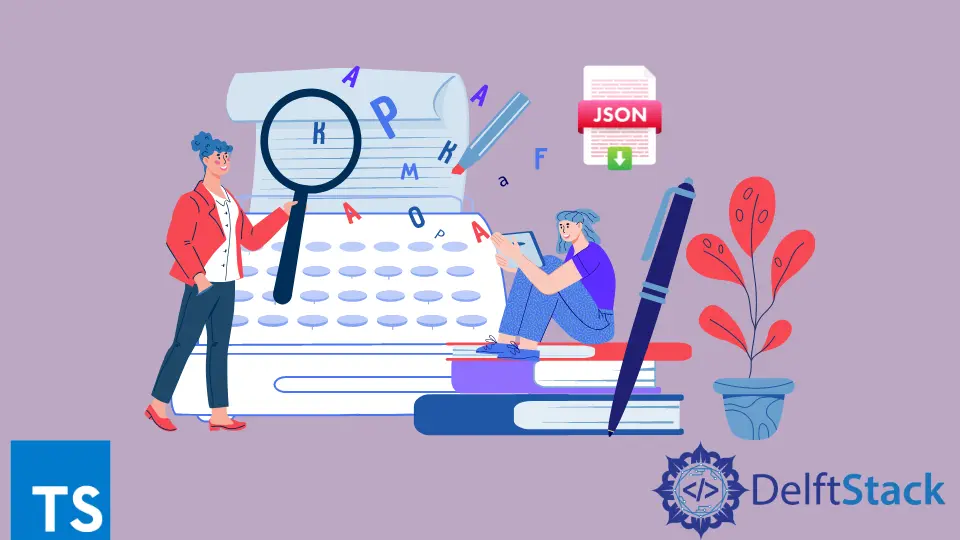
字符串是互联网上的一种通信方式,所有数据都以一种非常流行的格式传输,即 JSON。这种数据的 JSON 表示通常表示 TypeScript 中的一个对象甚至一个类。
本教程将重点介绍如何安全地将 JSON 字符串解析回 TypeScript 对象。
使用 JSON.parse()
将 JSON 字符串解析为 TypeScript 中的对象
JavaScript 中使用的 JSON.parse()
函数也可以在 TypeScript 中使用,因为 TypeScript 是 JavaScript 的超集。但是,以下方法假定要转换为对象的 JSON 字符串具有与对象关联的所有必需属性。
示例代码:
interface Person {
name : string;
age : number;
}
const personString = `{"name" : "david", "age" : 20}`;
const personObj : Person = JSON.parse(personString);
console.log(personObj);
输出:
{
"name": "david",
"age": 20
}
在 TypeScript 中使用防护将 JSON 字符串安全转换为对象
用户定义的守卫可以检查 JSON 字符串是否包含所需的全部或部分属性,从而根据即时需要进一步处理或拒绝它。
示例代码:
interface Person {
name : string;
age : number;
}
function checkPersonType( obj : any ) : obj is Person {
return 'name' in obj && 'age' in obj;
}
const personString1 = `{"name" : "david"}`;
const personString2 = `{"name" : "david", "age" : 20 }`;
const personObj1 : Person = JSON.parse(personString1);
if ( checkPersonType(personObj1)) {
console.log(personObj1);
} else {
console.log(personString1 + ' cannot be parsed');
}
const personObj2 : Person = JSON.parse(personString2);
if ( checkPersonType(personObj2)) {
console.log(personObj2);
} else {
console.log(personString2 + ' cannot be parsed');
}
输出:
"{"name" : "david"} cannot be parsed"
{
"name": "david",
"age": 20
}
在 TypeScript 中使用 Partial
关键字避免运行时错误
JSON 字符串中可能缺少某些字段。在这种情况下,可以用一些默认值填充对象中的属性。
TypeScript 中的 Partial
关键字通过将对象中的所有属性设为可选来帮助我们实现这一点,因此可以与 null 检查运算符链接以设置默认值。
示例代码:
interface Person {
name : string;
age : number;
}
const personString = `{"name" : "david"}`;
const personObj : Partial<Person> = JSON.parse(personString);
console.log(personObj);
console.log( personObj.age ?? 30);
输出:
{
"name": "david"
}
30
因此,虽然 JSON 字符串没有 Person
接口所要求的 age
属性,但空值检查或 ??
运算符用于为 age
属性设置默认值。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe