How to Initializing TypeScript Object From a JSON Object
- Deserializing of an Object Into a TypeScript Object
- Dynamic Approach in Deserializing a Typescript Object
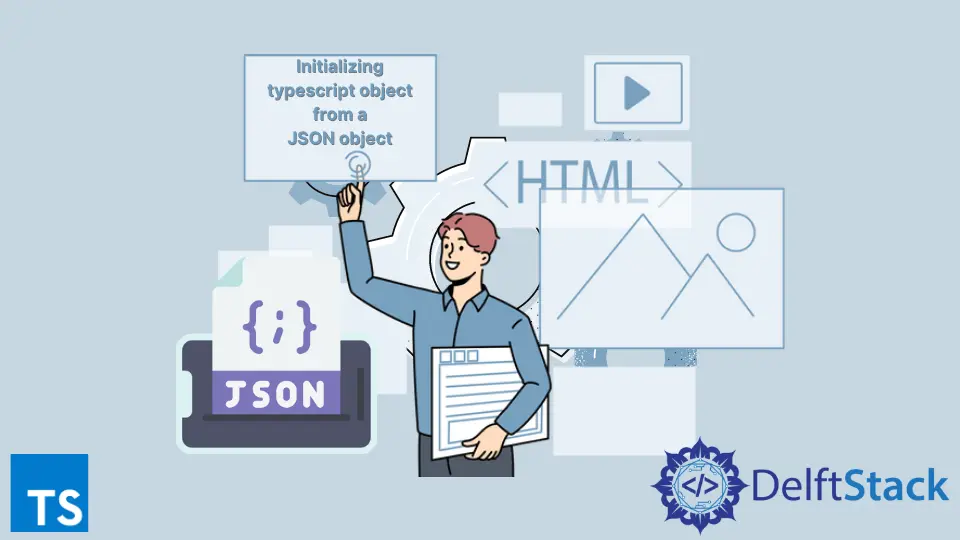
When working with APIs using TypeScript, the data is serialized in strings for transport through internet protocols. However, using the data received from the APIs needs to be deserialized into TypeScript classes or objects as and when required.
This article will demonstrate different ways how to transform the received object into TypeScript types. So that type of support, IDE completions, and other features get accessible.
Deserializing of an Object Into a TypeScript Object
The JSON package in JavaScript does a good job parsing plain Objects into TypeScript Objects. The following code segment demonstrates how the parsing is done from a JSON string to a TypeScript object.
var jsonString : string =`{
"id": 1,
"title" : "The Great Gatsby",
"numPages" : 218
}`
interface BookInfo {
id : number ;
title : string ;
numPages : number ;
}
var Book : BookInfo = JSON.parse(jsonString);
console.log(Book);
console.log(Book.title);
Output:
{
"id": 1,
"title": "The Great Gatsby",
"numPages": 218
}
"The Great Gatsby"
The jsonString
may be part of the API response, which comes as a serialized string. Thus, as shown in this example, the variable Book
will provide completions like a normal TypeScript Object.
However, this does not work in the case of class Objects containing functions and constructors inside them. Consider the following code segment, which demonstrates this.
class Animal{
name : string;
legs : number;
constructor(name : string, legs : number){
this.name = name;
this.legs = legs;
}
getName(){
return this.name;
}
}
var jsonString : string = `{
"name" : "Tiger",
"legs" : 4
}`
var animalObj : Animal = JSON.parse(jsonString)
// this will give an error - animalObj.getName is not a function
animalObj.getName();
Thus from the animalObj
variable, we can access the name
and legs
fields. However, we cannot access the getName()
function as it is part of the Animal
class, which cannot be parsed directly but initialized.
Change the Animal
class so that JSON parsed objects can access the member functions. The parsed object must be initialized.
class Animal{
name : string;
legs : number;
constructor(name : string, legs : number){
this.name = name;
this.legs = legs;
}
getName(){
return this.name;
}
toObject(){
return {
name : this.name,
legs : this.legs.toString()
}
}
serialize() {
return JSON.stringify(this.toObject());
}
static fromJSON(serialized : string) : Animal {
const animal : ReturnType<Animal["toObject"]> = JSON.parse(serialized);
return new Animal(
animal.name,
animal.legs
)
}
}
var jsonString : string = `{
"name" : "Tiger",
"legs" : 4
}`
var animalObj : Animal = Animal.fromJSON(jsonString);
console.log(animalObj)
// this will work now
console.log(animalObj.getName());
Output:
Animal: {
"name": "Tiger",
"legs": 4
}
"Tiger"
Dynamic Approach in Deserializing a Typescript Object
Now, this approach requires knowing exactly the properties of a class. The following discusses a more dynamic approach in deserializing a typescript object.
In this method, the author of the class has complete control over the deserializing logic and can work with multiple classes, as demonstrated.
interface Serializable<T> {
deserialize(input : Object) : T;
}
class Furniture implements Serializable<Furniture> {
price : number;
deserialize(input) {
this.price = Number(input.price);
return this;
}
getPrice(){
return this.price;
}
}
class Chair implements Serializable<Chair> {
id : string;
furniture : Furniture;
deserialize(input) {
this.id = input.id;
this.furniture = new Furniture().deserialize(input.furniture);
return this;
}
}
var jsonString = `{
"id" : "2323",
"furniture" : {
"price": 3000
}
}`
var chair = new Chair().deserialize(JSON.parse(jsonString))
console.log(chair)
Output:
Chair: {
"id": "2323",
"furniture": {
"price": 3000
}
}