Hashmap and Dictionary Interface in TypeScript
- The Interface in TypeScript
- The Hashmap or Dictionary in TypeScript
-
Create Hashmap or Dictionary Using
Map
Class in TypeScript -
Create Hashmap or Dictionary Using
Record
Type in TypeScript
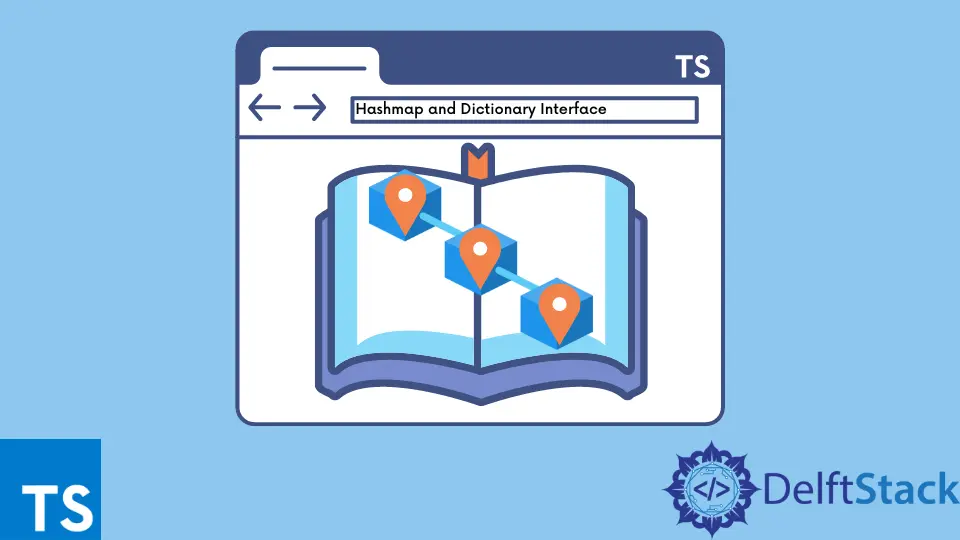
This tutorial provides guidelines about implementing Hashmap or Dictionary interface in TypeScript.
The Interface in TypeScript
TypeScript has a core principle of type checking that focuses on a value’s shape, sometimes called duck typing
or structural subtyping
. Interface in TypeScript fills the role of defining contracts within the code and the code outside of the project.
Code Snippet:
interface LabeledVal {
label: string;
}
function printLabelTraces(labeledObject: LabeledVal) {
console.log(labeledObject.label);
}
let myObject = { size: 10, label: "Size 10 Object" };
printLabelTraces(myObject);
Output:
The object implements the interface LabeledVal
, and we pass to printLabelTraces
. If the object we have passed to the function successfully meets the requirements listed in the interface, then it’s allowed.
The Hashmap or Dictionary in TypeScript
Hashmap in TypeScript is a simple object which accepts a symbol or a string as its key. Dictionary in statically typed programming languages are Key/Value
pair collections which contains a very flexible type called Object
.
Let’s look at how we can define Hashmap or Dictionary interface in TypeScript.
Code Snippet:
let objVal:IHash={
firstVal:"Ibrahim",
secondVal:"Alvi"
}
console.log(objVal)
Output:
The objVal
object implements the IHash
interface, which restricts the object to have its keys and values to be of type string
. Else will throw an error.
You can also define an interface with specific values of different types. Let’s consider the following example of the PersonInterface
with its general information.
Code Snippet:
let objVal:PersonInterface={
firstName:"Ibrahim",
lastName:"Alvi",
gender:"Male",
age:22
}
console.log(objVal)
Output:
Create Hashmap or Dictionary Using Map
Class in TypeScript
We can create Hashmap or Dictionary interface by utilizing the Map
class, a new TypeScript data structure that stores a key-value
pair and remembers the key’s original insertion order.
Code Snippet:
let mapValue = new Map<object, string>();
let key = new Object();
mapValue.set(key, "Ibrahim Alvi");
console.log(mapValue.get(key));
Output:
Create Hashmap or Dictionary Using Record
Type in TypeScript
Record
in TypeScript is one of the available utility types. This utility can also map a type’s properties to another type.
Code Snippet:
const hashMap : Record<string, string> = {
key1: 'val1',
key2: 'val2',
}
console.log(hashMap);
Output:
Lastly, we will create a sample program using the Record
type with the interface for creating a Hashmap or Dictionary-like object in TypeScript.
Code Snippet:
interface CatInformation {
age: number;
breed: string;
}
type CatName = "Muhammad" | "Ibrahim" | "Alvi";
const cats: Record<CatName, CatInformation> = {
Muhammad: { age: 10, breed: "Persian" },
Ibrahim: { age: 5, breed: "Maine Coon" },
Alvi: { age: 16, breed: "British Shorthair" },
};
console.log(`Muhammad's cat details are :${JSON.stringify(cats.Muhammad)},Ibrahim cat details are :${JSON.stringify(cats.Ibrahim)},Alvi cat details are :${JSON.stringify(cats.Alvi)},`);
The above code has a CatInformation
interface and a CatName
type object with three specific categories; the cats
object will be of type Record
with its Keys of type CatName
and value of type CatInformation
.
Output:
Ibrahim is a Full Stack developer working as a Software Engineer in a reputable international organization. He has work experience in technologies stack like MERN and Spring Boot. He is an enthusiastic JavaScript lover who loves to provide and share research-based solutions to problems. He loves problem-solving and loves to write solutions of those problems with implemented solutions.
LinkedIn