TypeScript 中的 Hashmap 和字典接口
- TypeScript 中的接口
- TypeScript 中的 Hashmap 或字典
-
在 TypeScript 中使用
Map
类创建 Hashmap 或字典 -
在 TypeScript 中使用
Record
类型创建 Hashmap 或字典
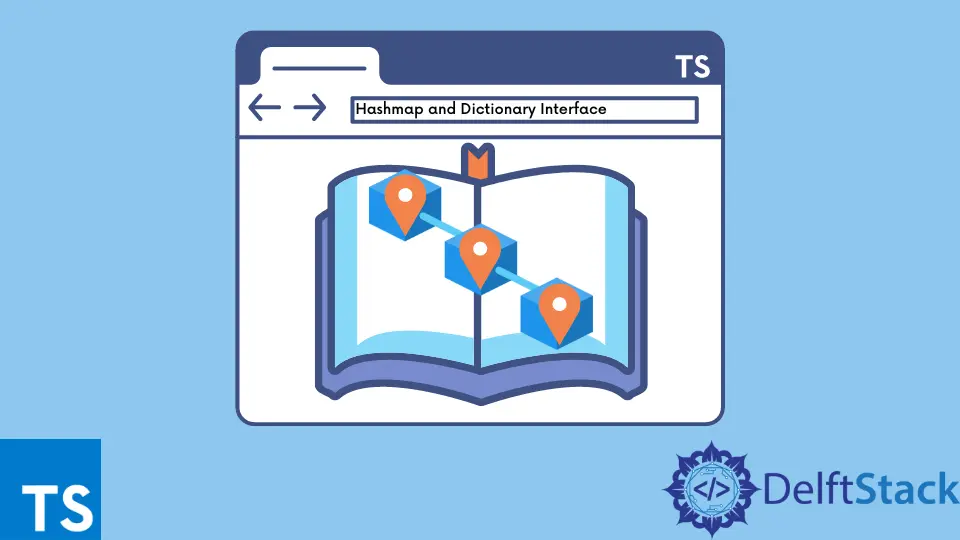
本教程提供了有关在 TypeScript 中实现 Hashmap 或 Dictionary 接口的指南。
TypeScript 中的接口
TypeScript 有一个类型检查的核心原则,它侧重于值的形状,有时称为鸭子类型
或结构子类型
。TypeScript 中的接口充当了在代码内和项目外定义合约的角色。
代码片段:
interface LabeledVal {
label: string;
}
function printLabelTraces(labeledObject: LabeledVal) {
console.log(labeledObject.label);
}
let myObject = { size: 10, label: "Size 10 Object" };
printLabelTraces(myObject);
输出:
该对象实现接口 LabeledVal
,我们传递给 printLabelTraces
。如果我们传递给函数的对象成功满足接口中列出的要求,那么它是允许的。
TypeScript 中的 Hashmap 或字典
TypeScript 中的 Hashmap 是一个简单的对象,它接受符号或字符串作为其键。静态类型编程语言中的字典是键/值
对集合,其中包含一个非常灵活的类型,称为对象
。
让我们看看如何在 TypeScript 中定义 Hashmap 或 Dictionary 接口。
代码片段:
let objVal:IHash={
firstVal:"Ibrahim",
secondVal:"Alvi"
}
console.log(objVal)
输出:
objVal
对象实现 IHash
接口,该接口限制对象的键和值是 string
类型。否则会抛出错误。
你还可以定义具有不同类型的特定值的接口。让我们参考下面的 PersonInterface
示例及其一般信息。
代码片段:
let objVal:PersonInterface={
firstName:"Ibrahim",
lastName:"Alvi",
gender:"Male",
age:22
}
console.log(objVal)
输出:
在 TypeScript 中使用 Map
类创建 Hashmap 或字典
我们可以利用 Map
类创建 Hashmap 或 Dictionary 接口,这是一种新的 TypeScript 数据结构,它存储 key-value
对并记住键的原始插入顺序。
代码片段:
let mapValue = new Map<object, string>();
let key = new Object();
mapValue.set(key, "Ibrahim Alvi");
console.log(mapValue.get(key));
输出:
在 TypeScript 中使用 Record
类型创建 Hashmap 或字典
TypeScript 中的记录
是可用的实用程序类型之一。此实用程序还可以将类型的属性映射到另一种类型。
代码片段:
const hashMap : Record<string, string> = {
key1: 'val1',
key2: 'val2',
}
console.log(hashMap);
输出:
最后,我们将使用 Record
类型创建一个示例程序,其接口用于在 TypeScript 中创建 Hashmap 或类似字典的对象。
代码片段:
interface CatInformation {
age: number;
breed: string;
}
type CatName = "Muhammad" | "Ibrahim" | "Alvi";
const cats: Record<CatName, CatInformation> = {
Muhammad: { age: 10, breed: "Persian" },
Ibrahim: { age: 5, breed: "Maine Coon" },
Alvi: { age: 16, breed: "British Shorthair" },
};
console.log(`Muhammad's cat details are :${JSON.stringify(cats.Muhammad)},Ibrahim cat details are :${JSON.stringify(cats.Ibrahim)},Alvi cat details are :${JSON.stringify(cats.Alvi)},`);
上面的代码有一个 CatInformation
接口和一个 CatName
类型的对象,具有三个特定的类别; cats
对象的类型为 Record
,其键类型为 CatName
,值类型为 CatInformation
。
输出:
Ibrahim is a Full Stack developer working as a Software Engineer in a reputable international organization. He has work experience in technologies stack like MERN and Spring Boot. He is an enthusiastic JavaScript lover who loves to provide and share research-based solutions to problems. He loves problem-solving and loves to write solutions of those problems with implemented solutions.
LinkedIn