How to Convert String to Date in Angular2 TypeScript
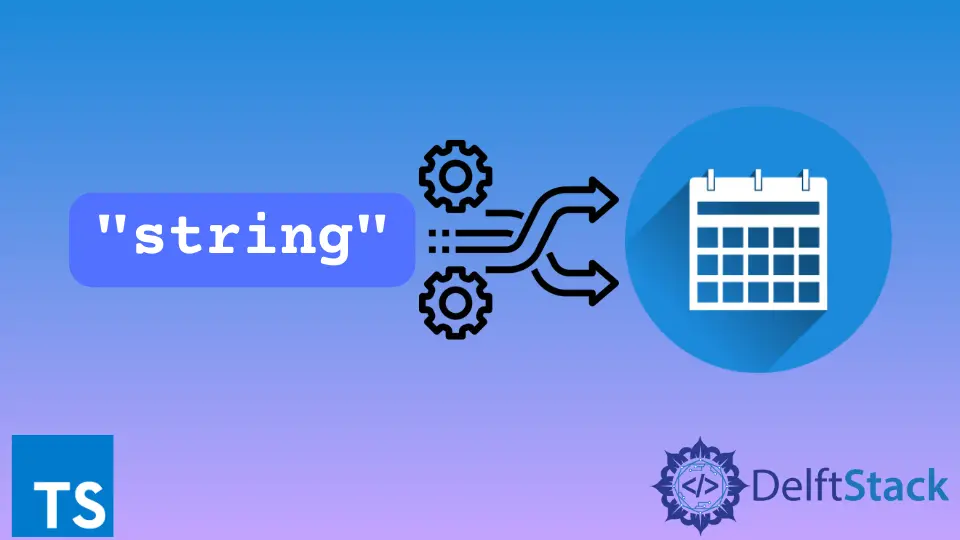
This tutorial provides brief guidelines about converting String to Date in Angular2 TypeScript. It also explains the Date object and the purpose of this method in TypeScript.
Date
Object in TypeScript
The Date
object in TypeScript represents a date and time functionality. The Date
object allows us to get the day, month, year, millisecond, second, minute and hour.
If the Date
object is being called without any argument passed to its constructor, it will return the time and date of the user’s computer. Date
object provides us with the functions which deal with Coordinated Universal Time (UTC)
time.
Following are some ways to create a new date object. Using the Date
object returns the current date and time.
Code:
let date: Date = new Date();
console.log("Date = " + date); //Date
Output:
The following way creates a new date object as zero time plus milliseconds.
let date: Date = new Date(500000000000);
console.log("Date = " + date);
Output:
Use DatePipe
Method to Convert String to Date
in TypeScript
To convert the String to the Date
filter, you can use the date filter that helps convert the Date
and displays it in a specific format. Let’s consider the following example in which the DatePipe
method is used, an Angular
method that formats a date value according to locale rules.
DatePipe
on official Angular documentation is executed only when it detects a pure change to the input value. The String can be converted into Date
format using the following method.
// format date in typescript
getFormatedDate(date: Date, format: string) {
const datePipe = new DatePipe('en-US');
return datePipe.transform(date, format);
}
To convert the Date
to the user timezone, we can do manual calculations on the date as performed below.
Code:
// Convert date to user timezone
const localDate: Date = this.convertToLocalDate('01/01/2021');
convertToLocalDate(responseDate: any) {
try {
if (responseDate != null) {
if (typeof (responseDate) === 'string') {
if (String(responseDate.indexOf('T') >= 0)) {
responseDate = responseDate.split('T')[0];
}
if (String(responseDate.indexOf('+') >= 0)) {
responseDate = responseDate.split('+')[0];
}
}
responseDate = new Date(responseDate);
const newDate = new Date(responseDate.getFullYear(), responseDate.getMonth(), responseDate.getDate(), 0, 0, 0);
const userTimezoneOffset = newDate.getTimezoneOffset() * 60000;
const finalDate: Date = new Date(newDate.getTime() - userTimezoneOffset);
return finalDate > Util.minDateValue ? finalDate : null;
} else {
return null;
}
} catch (error) {
return responseDate;
}
}
Output:
Ibrahim is a Full Stack developer working as a Software Engineer in a reputable international organization. He has work experience in technologies stack like MERN and Spring Boot. He is an enthusiastic JavaScript lover who loves to provide and share research-based solutions to problems. He loves problem-solving and loves to write solutions of those problems with implemented solutions.
LinkedIn