Angular2 TypeScript で文字列を日付に変換する
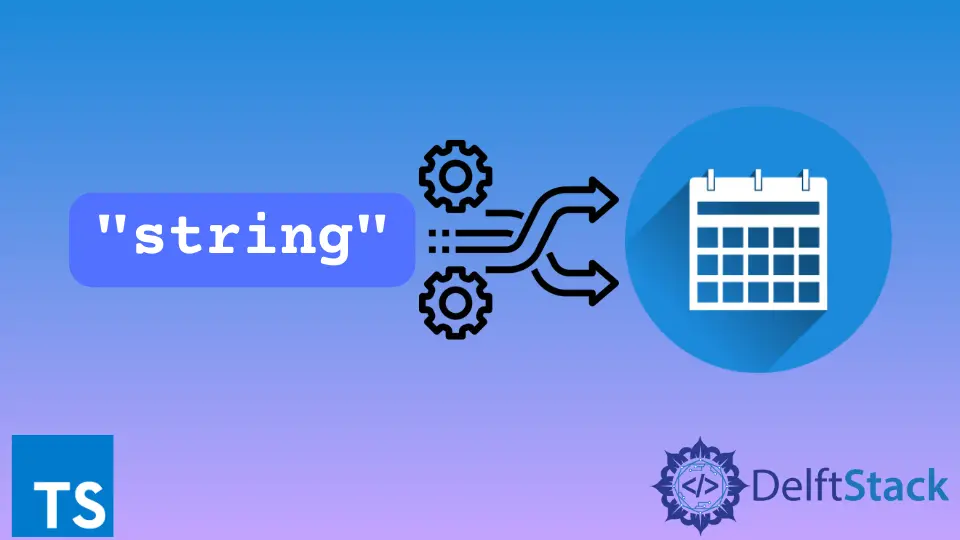
このチュートリアルでは、Angular2 TypeScript で String を Date に変換するための簡単なガイドラインを提供します。 また、Date オブジェクトと TypeScript でのこのメソッドの目的についても説明します。
TypeScript の Date
オブジェクト
TypeScript の Date
オブジェクトは、日付と時刻の機能を表します。 Date
オブジェクトを使用すると、日、月、年、ミリ秒、秒、分、時間を取得できます。
コンストラクターに引数を渡さずに Date
オブジェクトを呼び出すと、ユーザーのコンピューターの時刻と日付が返されます。 Date
オブジェクトは、協定世界時 (UTC)
時刻を処理する関数を提供します。
以下は、新しい日付オブジェクトを作成するいくつかの方法です。 Date
オブジェクトを使用すると、現在の日付と時刻が返されます。
コード:
let date: Date = new Date();
console.log("Date = " + date); //Date
出力:
次の方法では、新しい日付オブジェクトをゼロ時間プラスミリ秒として作成します。
let date: Date = new Date(500000000000);
console.log("Date = " + date);
出力:
TypeScript で DatePipe
メソッドを使用して文字列を Date
に変換する
文字列を Date
フィルターに変換するには、Date
を変換して特定の形式で表示するのに役立つ日付フィルターを使用できます。 DatePipe
メソッドが使用されている次の例を考えてみましょう。これは、ロケール規則に従って日付値をフォーマットする Angular
メソッドです。
公式の Angular ドキュメントの DatePipe
は、入力値への純粋な変更を検出した場合にのみ実行されます。 String は、次のメソッドを使用して Date
形式に変換できます。
// format date in typescript
getFormatedDate(date: Date, format: string) {
const datePipe = new DatePipe('en-US');
return datePipe.transform(date, format);
}
Date
をユーザーのタイムゾーンに変換するには、以下で実行するように日付を手動で計算できます。
コード:
// Convert date to user timezone
const localDate: Date = this.convertToLocalDate('01/01/2021');
convertToLocalDate(responseDate: any) {
try {
if (responseDate != null) {
if (typeof (responseDate) === 'string') {
if (String(responseDate.indexOf('T') >= 0)) {
responseDate = responseDate.split('T')[0];
}
if (String(responseDate.indexOf('+') >= 0)) {
responseDate = responseDate.split('+')[0];
}
}
responseDate = new Date(responseDate);
const newDate = new Date(responseDate.getFullYear(), responseDate.getMonth(), responseDate.getDate(), 0, 0, 0);
const userTimezoneOffset = newDate.getTimezoneOffset() * 60000;
const finalDate: Date = new Date(newDate.getTime() - userTimezoneOffset);
return finalDate > Util.minDateValue ? finalDate : null;
} else {
return null;
}
} catch (error) {
return responseDate;
}
}
出力:
Ibrahim is a Full Stack developer working as a Software Engineer in a reputable international organization. He has work experience in technologies stack like MERN and Spring Boot. He is an enthusiastic JavaScript lover who loves to provide and share research-based solutions to problems. He loves problem-solving and loves to write solutions of those problems with implemented solutions.
LinkedIn