How to Write Text Into a File in Scala
-
Use
PrintWriter
to Write Text Into a File in Scala -
Use
FileWriter
to Write Text Into a File in Scala - Use the Java NIO (New Input/Output) Package to Write Text Into a File in Scala
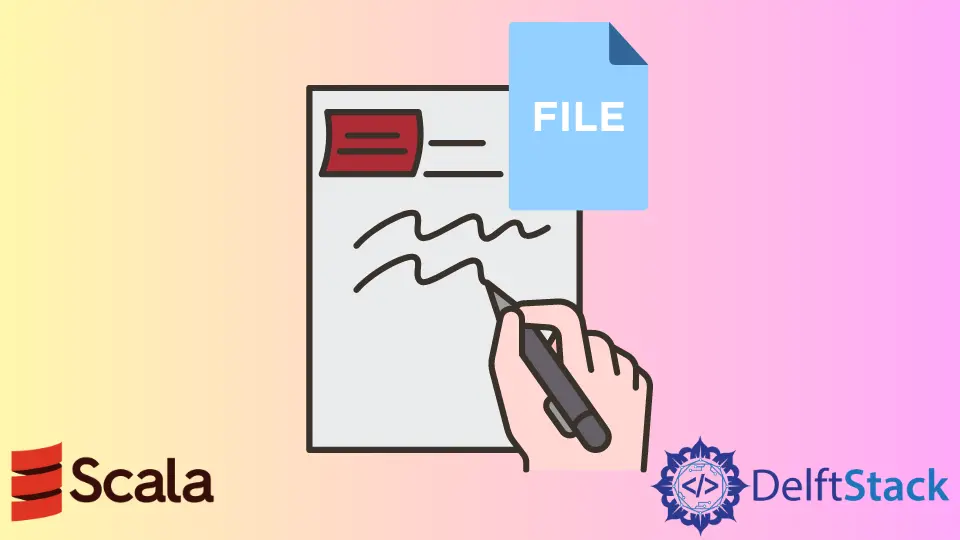
This article will discuss the different methods of writing text into Scala files.
We use the java.io
objects to write text to a file in Scala, as it relies on Java objects for performing various functions.
Use PrintWriter
to Write Text Into a File in Scala
The following steps must be performed to write to a file in Scala.
-
Create a
PrintWriter
object using thefileName
. -
Use the
write()
method to write to a file. -
Use the
close
function to close the file after completing the write operation.
Example:
import java.io._
object MyObject {
def main(args: Array[String]) {
val writer = new PrintWriter(new File("C:\\Users\\srjsu\\OneDrive\\Desktop\\temp.txt")) //specify the file path
writer.write("Iron Man is the best")
writer.close()
}
}
Output:
Iron Man is the best
After executing the program, the output will be written in the temp.txt
file present in the Desktop folder.
Use FileWriter
to Write Text Into a File in Scala
The following steps must be performed to write to a file in Scala using FileWriter
.
-
Create a
FileWriter
object using thefileName
. -
Use the
write()
method to write to a file. -
Use the
close
function to close the file after completing the write operation.
Example:
import java.io._
object MyObject {
def main(args: Array[String]) {
val writeFile = new File("C:\\Users\\srjsu\\OneDrive\\Desktop\\test.txt")
val text = "We are learning Scala programming language"
val writer = new BufferedWriter(new FileWriter(writeFile))
writer.write(text)
writer.close()
}
}
Output:
We are learning Scala programming language
After executing the program, the output above will be written in the test.txt
file present in the Desktop folder.
Use the Java NIO (New Input/Output) Package to Write Text Into a File in Scala
This is the best method to write text into a file in Scala. Using the Java NIO package, we can write to a file in a very concise manner, that is, typing less code.
Example 1:
import java.nio.file.{Paths, Files}
import java.nio.charset.StandardCharsets
object MyObject {
def main(args: Array[String]) {
val inputText = "I remembered black skies\nThe lightning all around me\nI remembered each flash\nAs time began to blur\nLike a startling sign\nThat fate had finally found me\nAnd your voice was all I heard\nThat I get what I deserve\nSo give me reason\nTo prove me wrong\nTo wash this memory clean\nLet the floods cross\nThe distance in your eyes";
Files.write(Paths.get("C:\\Users\\srjsu\\OneDrive\\Desktop\\test.txt"), inputText.getBytes(StandardCharsets.UTF_8))
}
}
Output:
I remembered black skies
The lightning all around me
I remembered each flash
As time began to blur
Like a startling sign
That fate had finally found me
And your voice was all I heard
That I get what I deserve
So give me reason
To prove me wrong
To wash this memory clean
Let the floods cross
The distance in your eyes
In the example above, getBytes()
encodes the string to the UTF-8 charset.
Example 2:
If the input string is small, we can write everything in a single line.
import java.nio.file.{Paths, Files}
import java.nio.charset.StandardCharsets
object MyObject {
def main(args: Array[String]) {
Files.write(Paths.get("C:\\Users\\srjsu\\OneDrive\\Desktop\\test.txt"), "HELLO WORLD!".getBytes(StandardCharsets.UTF_8))
}
}
Output:
HELLO WORLD!
After executing the program, the outputs above will be written in the test.txt
file for both code examples.