How to Read an Entire File in Scala
Suraj P
Feb 02, 2024
- Method 1: Reading The Whole File at a Time in Scala
- Method 2: Reading The File Line by Line in Scala
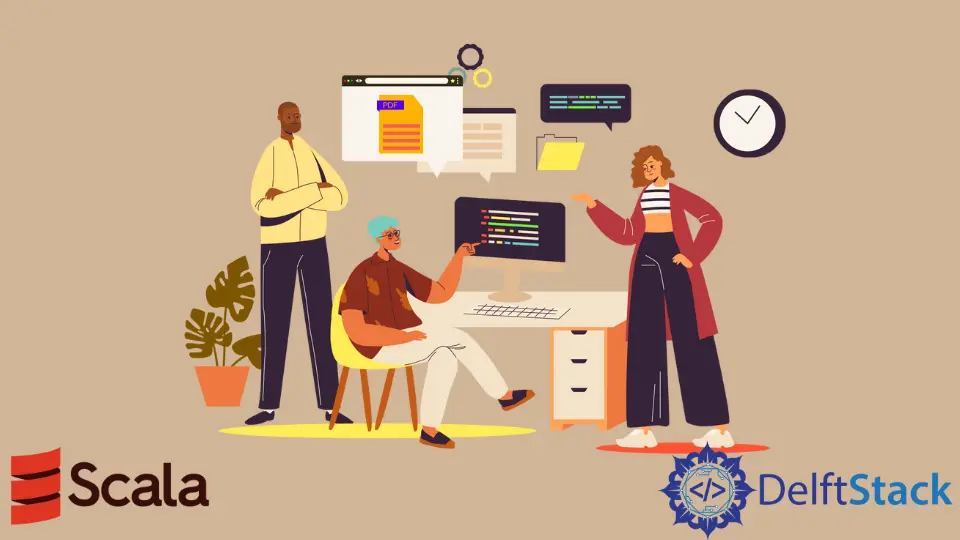
Scala provides a class to read files called Source
. We call the fromFile()
method of class Source for reading the file’s contents, including filename as an argument to read the file’s contents.
Method 1: Reading The Whole File at a Time in Scala
We perform the following steps to read a file in Scala:
- First, we specify the filename along with its full path.
- Use
Source.fromFile
to create a source from where the file will be loaded. - Use the
mkstring
method to make the whole data into a string.
import scala.io.Source
object demo {
def main(args:Array[String]): Unit =
{
val fileName= "C:\\Users\\user\\Desktop\\testFile.txt";
val Str = Source.fromFile(fileName).mkString; //using mkString method
println(Str)
}
}
Output:
[Chester Bennington:]
It's so unreal
[Mike Shinoda:]
It's so unreal, didn't look out below
Watch the time go right out the window
Trying to hold on but didn't even know
I wasted it all to watch you go
[Chester Bennington:]
Watch you go
Process finished with exit code 0
Method 2: Reading The File Line by Line in Scala
We perform the following steps to read a file in Scala:
- First, we specify the filename along with its full path.
- Use
Source.fromFile
to create a source from where the file will be loaded. - Use the
getLines()
method to read data line by line and then print or process it accordingly.
import scala.io.Source
object demo {
def main(args:Array[String]): Unit =
{
val fileName= "C:\\Users\\user\\Desktop\\testFile.txt"; //filepath
val fileSource = Source.fromFile(fileName)
for(lines<-fileSource.getLines()) {
println(lines)
}
fileSource.close(); //closing the file
}
}
The above code reads testFile.txt
, which is present in the Desktop folder.
Output:
"In The End"
[Chester Bennington:]
It starts with one
[Mike Shinoda:]
One thing I don't know why
It doesn't even matter how hard you try
Keep that in mind, I designed this rhyme
To explain in due time
[Chester Bennington:]
All I know
[Mike Shinoda:] Time is a valuable thing
Watch it fly by as the pendulum swings
Watch it count down to the end of the day
The clock ticks life away
Author: Suraj P