The Modulo Operator in Scala
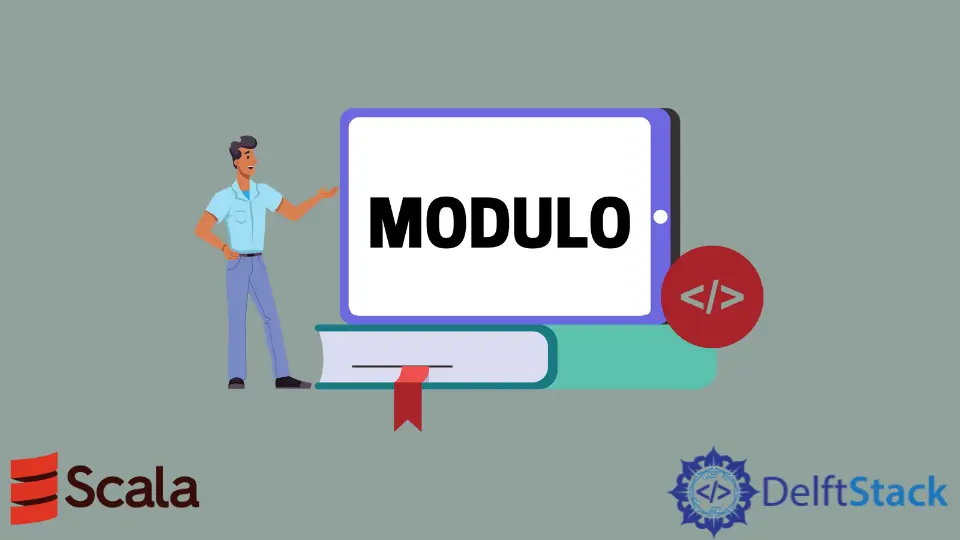
In this article, we will learn how to work with the modulo operator (%
) and some other methods related to modulo in Scala.
the Modulo Operator in Scala
The modulo operator %
is used to give the remainder, specifically the signed remainder, when two numbers are divided in Scala.
Syntax:
val z = x % y
z
contains the remainder when x
is divided by y
. Let’s see an example to understand it better.
Example code:
object MyClass {
def main(args: Array[String]) {
val x = 15
val y = 4
val z = x % y
println(z)
}
}
Output:
3
When 15 is divided by 4, the remainder is 3, which is stored in the variable z
and then printed on the console,
the floorMod()
Method in Scala
In Scala, Math floorMod()
is used to return the floor modulo of the passed arguments. We can overload this method to take an int and long argument.
If the divisor is zero, it throws the ArithmeticException divided by zero
.
Syntax:
Math.floorMod(data_type x, data_type y)
Here, the parameter a
is the dividend, and the parameter y
is the divisor. These parameters can either be an int or long.
This method returns the floor modulo of (x - (floorDiv(x,y)*y)
.
Example code:
object MyClass {
def main(args: Array[String]) {
val x = 15
val y = 4
val z = Math.floorMod(x,y)
println(z)
}
}
Output:
3
One common question is: what is the difference between the floorMod()
method and the %
operator in Scala?.
Both are the same when our arguments, i.e., the dividend and divisor, are positive
, but they behave differently when one or both are negative. This mainly happens because the method returns the floor modulo of (x - (floorDiv(x,y)*y)
, and it is dependent on the floorDiv()
method for its answer.
In simple words, the floorMod()
method gives the same sign as its divisor
, i.e., whatever the sign y
has, the same sign will be followed when returning the result.
Example code:
object MyClass {
def main(args: Array[String]) {
println("first: ")
println(Math.floorMod(+4, -3));
println((+4 % -3))
println("second: ")
println(Math.floorMod(-4, +3))
println((-4 % +3))
println("Third: ")
println(Math.floorMod(-4, -3))
println((-4 % -3))
}
}
Output:
first:
-2
1
second:
2
-1
Third:
-1
-1
the %(y:Float)
Method in Scala
In Scala, %(y:Float)
is used when we want to return the remainder when a double is divided by a float value.
Syntax:
double_value.%(y : Float)
Example code:
object MyClass {
def main(args: Array[String]) {
val rem = (16.50012.toDouble).%(13:Float)
println("The remainder is")
println(rem)
}
}
Output:
The remainder is
3.500119999999999