Difference Between :: And ::: In Scala
- Understanding the :: Operator
- Understanding the ::: Operator
- Key Differences Between :: and :::
- Conclusion
- FAQ
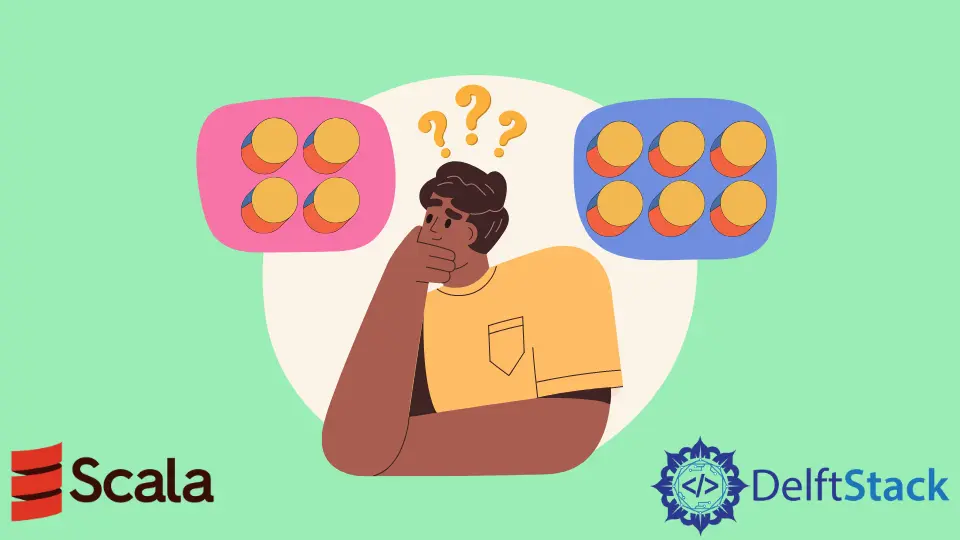
Understanding the nuances of Scala can significantly enhance your programming skills, especially when it comes to list manipulation. Among the many operators Scala offers, ::
and :::
are two that often confuse developers, particularly beginners. While they may seem similar at first glance, they serve distinct purposes when it comes to list operations.
In this article, we will delve into the differences between these two operators, providing clear explanations and examples to help you grasp their unique functionalities. By the end, you’ll have a solid understanding of how to use ::
and :::
effectively in your Scala projects.
Understanding the :: Operator
The ::
operator, also known as the “cons” operator, is used to prepend an element to the front of a list. This operator is particularly useful when you want to build lists in a functional programming style. When you use ::
, you’re effectively creating a new list that consists of the element you added and the original list.
Here’s a simple example to illustrate how the ::
operator works in Scala:
val list1 = List(2, 3, 4)
val newList = 1 :: list1
In this code, we start with list1
, which contains the elements 2, 3, and 4. By using 1 :: list1
, we create a new list called newList
that now contains 1 at the front, followed by the elements of list1
.
Output:
List(1, 2, 3, 4)
The ::
operator is right-associative, meaning that if you chain multiple ::
operations, Scala evaluates them from right to left. For instance:
val anotherList = 0 :: 1 :: list1
This creates a list that starts with 0, followed by 1 and then the elements of list1
.
Output:
List(0, 1, 2, 3, 4)
Using ::
is efficient because it doesn’t create a copy of the original list; it simply points to it. This makes it a preferred choice when you want to build lists incrementally.
Understanding the ::: Operator
On the other hand, the :::
operator is used for concatenating two lists. Unlike ::
, which adds a single element to the front of a list, :::
combines entire lists into one. This operator is very handy when you want to merge multiple lists together.
Let’s see how :::
works with a practical example:
val listA = List(1, 2, 3)
val listB = List(4, 5, 6)
val combinedList = listA ::: listB
In this example, we have two lists, listA
and listB
. By using listA ::: listB
, we create a new list called combinedList
that contains all elements from both lists.
Output:
List(1, 2, 3, 4, 5, 6)
The :::
operator is left-associative, which means it evaluates from left to right. If you have multiple lists to concatenate, you can chain them together like this:
val listC = List(7, 8)
val allCombined = listA ::: listB ::: listC
This creates a single list that includes elements from listA
, listB
, and listC
.
Output:
List(1, 2, 3, 4, 5, 6, 7, 8)
Using :::
is straightforward, but keep in mind that it creates a new list that contains all the elements from the lists being merged. This can have performance implications if you are working with large lists, as it requires additional memory allocation.
Key Differences Between :: and :::
Now that we’ve explored both operators, let’s summarize the key differences between ::
and :::
:
- Functionality:
::
is used to prepend a single element to a list, while:::
is used to concatenate two lists. - Associativity:
::
is right-associative, evaluating from right to left, whereas:::
is left-associative, evaluating from left to right. - Performance:
::
is generally more efficient as it doesn’t create a copy of the original list, while:::
creates a new list containing elements from both lists.
Understanding these differences will help you choose the right operator based on your specific needs when working with lists in Scala.
Conclusion
In summary, while both ::
and :::
are essential operators in Scala for list manipulation, they serve different purposes. The ::
operator allows you to prepend an element to the front of a list, making it a powerful tool for building lists in a functional programming style. In contrast, the :::
operator is used for concatenating two lists into one. By mastering these operators, you can enhance your Scala programming skills and write more efficient code. So, the next time you find yourself working with lists in Scala, remember the distinct roles of ::
and :::
to make your coding experience smoother and more intuitive.
FAQ
-
What does the :: operator do in Scala?
The::
operator is used to prepend a single element to the front of a list in Scala. -
How does the ::: operator differ from :: in Scala?
The:::
operator concatenates two lists, while::
adds a single element to the front of a list. -
Is :: more efficient than ::: in Scala?
Yes,::
is generally more efficient as it doesn’t create a copy of the original list, whereas:::
creates a new list containing elements from both lists. -
Can I use :: and ::: with other data types in Scala?
Yes, both operators can be used with any data type that is compatible with Scala’s list structures. -
How do I chain multiple :: operators in Scala?
You can chain multiple::
operators together, and they will be evaluated from right to left, allowing you to build a list incrementally.