How to Convert ArrayD to Array2 in Rust
- Array in Rust
- Initialization and Declaration of Array in Rust
- ND Array in Rust
- Array 2 in Rust
-
Conversion of
ArrayD
toArray2
in Rust
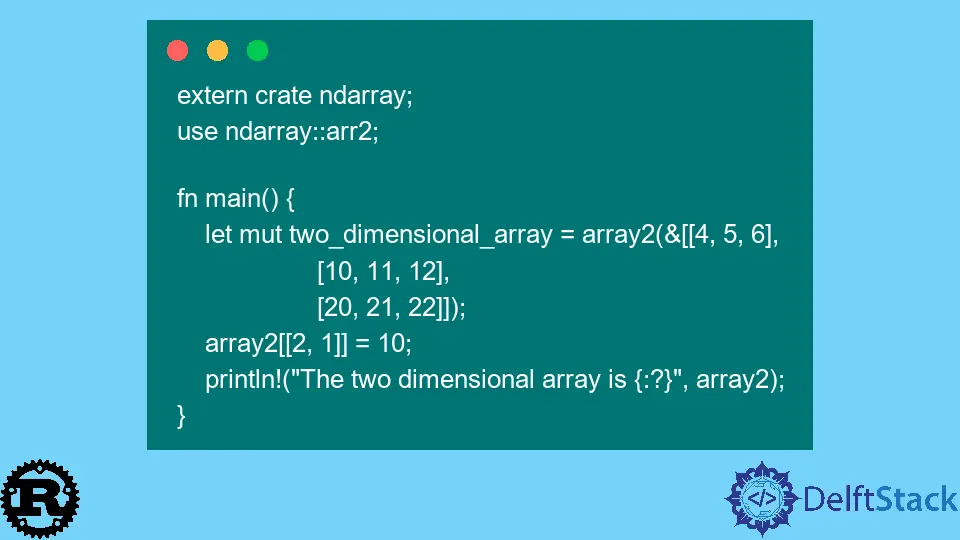
This article is about converting ArrayD
to Array2
in Rust.
Array in Rust
An array is a collection of values of the same type. A group of the importance of the same data type is referred to as an array.
In Rust programming, an array is a fixed-size collection of elements denoted by [T; N]
, where T
represents the element type, and N
denotes the array’s compile-time constant size.
An array can be created in two distinct ways.
- Simply a list of
[x,y,z]
elements. - Repetition of the expression
[N, X]
. This creates an array containingN
instances ofX
.
Initialization and Declaration of Array in Rust
There are multiple syntaxes for creating an array in Rust. Some of them are:
Syntaxes:
Syntax 1:
let var_name = [val_1,val_2,val_3];
Example:
let _cars = ["BMW","Toyota","Ferrari"];
Syntax 2:
let var_name:[dataType;size_of_array] = [val_1,val_2,val_3];
Example:
let _numbers:[i32;3] = [1,2,3];
Syntax 3:
let var_name:[dataType;size_of_array] = [default_value,size_of_array];
Example:
let _num_array:[i32;3] = [0;3];
- In the 1st syntax,
cars
is the variable name andBMW
,Toyota
, andFerrari
are the array’s values. - In the 2nd syntax,
numbers
is the variable name,i32
is the data type,3
is array size, and1
,2
, and3
are the array’s values. - In the 3rd syntax,
num_array
is the variable name,i32
is the data type,3
is array size,0
is the default value for elements, and3
is the array’s size.
ND Array in Rust
The N-dimensional array data structure is frequently utilized in scientific computing and processing.
The ndarray
crate implements N-dimensional
array support in Rust. Other crates make extensive use of it.
Array 2 in Rust
Array 2 is the two-dimensional array. It can be easily created using the arr2
macro.
The example of creating a 3x3
2D array is given below.
extern crate ndarray;
use ndarray::arr2;
fn main() {
let mut two_dimensional_array = array2(&[[4, 5, 6],
[10, 11, 12],
[20, 21, 22]]);
array2[[2, 1]] = 10;
println!("The two dimensional array is {:?}", array2);
}
Output:
The two dimensional array is [[4, 5, 6],
[10, 11, 12],
[20, 21, 22]], shape=[3, 3], strides=[3, 1], layout=Cc (0x5), const ndim=2
Conversion of ArrayD
to Array2
in Rust
The conversion of an array to another is possible with the same type, but the dimensions should be different. The error will occur if the dimensions of the array do not agree.
So, the number of axes must match during the conversion.
To convert an n-dimensional array into a 2d array:
We can follow the following code if the variable type is ArrayD<f32>
.
let arr2d: Array2<f32> = arr2d.into_dimensionality::<Ix2>()?;
The above code is to convert it into a variable of type Array2<f32>
.
use ndarray::{ArrayD, Ix2, IxDyn};
let array = ArrayD::<f32>::zeros(IxDyn(&[5, 5]));
assert!(array.into_dimensionality::<Ix2>().is_ok());
Above is the code for creating a dynamic dimensional array and converting it into an I * 2
dimension.