How to Print Arrays in Rust
- Create Arrays in Rust
- Declare and Initialize Arrays in Rust
-
Use
println!
With the{:?}
Format Specifier to Print an Array in Rust - Use a Loop to Print an Array in Rust
- Conclusion
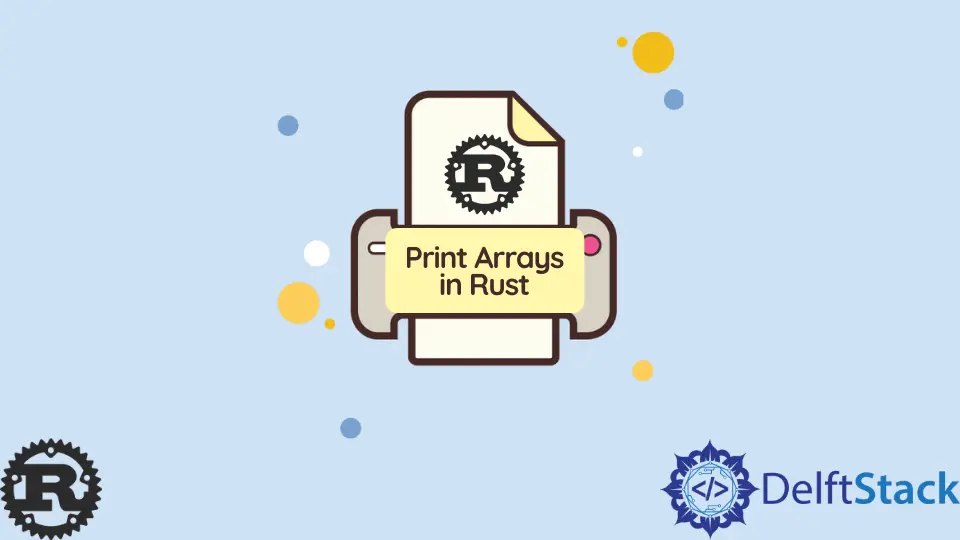
Rust is a robust systems programming language renowned for its speed, memory safety features, and built-in thread safety guarantees. It excels in crafting low-level code, such as device drivers and operating systems.
Furthermore, Rust offers higher-level features that simplify application development. In this tutorial, we’ll explore how to effectively print arrays in Rust programming.
Create Arrays in Rust
A group of things of a similar sort stored in a connected memory area is referred to as an array. Arrays are helpful because they allow us to store many values together in one variable and access them by index.
To create arrays in Rust, you use square brackets []
. The first element specifies the data type of the array’s elements, followed by the individual elements separated by commas.
Example:
let my_array = [1, 2];
let my_string_array = ["hello", "world"];
The first line inside the code block is an example of an integer array containing two elements, 1
and 2
. The second line of code is an example of a string array containing two elements, "hello"
and "world"
.
Rust primarily supports two methods for constructing arrays:
-
An array containing a list of items is used during initialization.
-
Repeat an expression that repeats a specific element several times.
The syntax for a repeat array is [N, X]
, where it generates an array with N
elements, each being a repetition of X
.
Declare and Initialize Arrays in Rust
Declaring an array is as easy as declaring any other variable:
let my_array = [1, 2, 3];
let my_array: Vec = vec![1, 2, 3];
The first method is more verbose but offers the flexibility to add more elements later without recreating the array.
Additionally, the following code declares a new array of characters with a type char
and sets its length to 5:
let letters = ['a', 'b', 'c', 'd', 'e'];
Use println!
With the {:?}
Format Specifier to Print an Array in Rust
The println!
function is used to print the provided string followed by a newline character. When it comes to printing arrays in Rust, the ?
operator is employed within the println!
function.
The ?
operator will print the specified expression if it evaluates to true
; otherwise, it will return nothing.
Below are the steps you can follow to use println!
with the {:?}
format specifier to print an array.
-
Create a variable(
demo
) for the array. -
Create a variable for the size of the array(
[&str; 7]
). -
Initialize the array with values.
-
Print out each value in the array using the
?
operator inside theprintln!
function.
Code:
fn main() {
let demo: [&str; 7] = ["Adil"; 7];
println!("Array {:?}", demo);
}
Output:
Array ["Adil", "Adil", "Adil", "Adil", "Adil", "Adil", "Adil"]
The 'demo'
array will be printed using its Debug trait implementation. The output shows the contents of the demo
array, which consists of seven elements, all containing the string "Adil"
.
Use a Loop to Print an Array in Rust
You can use a loop to iterate through the array’s elements and print each one individually. Here’s how:
-
Declare a variable
'arr'
and initialize it with an array of integers. -
Start a
'for'
loop to iterate over the elements of the'arr'
array. -
Use the
'println!'
macro to print the value of'element'
. -
{}'
is a placeholder for the'element'
value, and it will be replaced with the actual value during each iteration.
Code:
fn main() {
let arr = [1, 2, 3, 4, 5];
for element in arr.iter() {
println!("{}", element);
}
}
Output:
1
2
3
4
5
The output shows all the values of the element
. Meaning the program successfully printed all the contents of the array.
Conclusion
Rust is a versatile language that’s great for many programming tasks, especially when it comes to working with arrays. Whether you like the easy println!
method or the classic loop, knowing how to print arrays in Rust is vital for smooth debugging and development.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook