Understanding Class << Self in Ruby
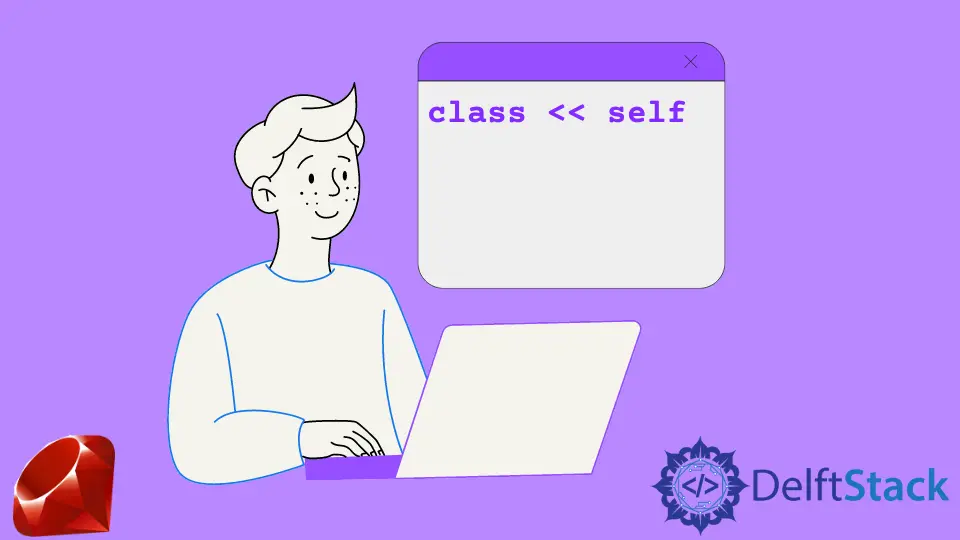
In Ruby OOP, class << self
is a syntax you would often encounter. It’s usually used to define class methods.
We will learn about the class << self
syntax and why it’s used to define class methods in this article.
Instance Method and Class Method in Ruby
The instance method and the class method are two of Ruby’s most common types of methods.
Example Code:
class Employee
def initialize(last_name, first_name)
@last_name = last_name
@first_name = first_name
end
def full_name
"#{@first_name} #{@last_name}"
end
def self.company_name
'Ruby LLC'
end
end
employee1 = Employee.new('Mark', 'David')
puts employee1.full_name
puts Employee.company_name
Output:
David Mark
Ruby LLC
full_name
is an instance
method in the above example and company_name
is the class
method. full_name
is specific to an instance of the Employee
whereas company
should return the same value for every instance of the class, which is defined as self.company_name
.
The self
in the example refers to the class itself, and as a result, we can also write it as:
class Employee
def self.company_name
'Ruby LLC'
end
end
Output:
Handle recruitment
The following is another way to write the above code:
Example Code:
class Employee
def initialize(last_name, first_name)
@last_name = last_name
@first_name = first_name
end
def full_name
"#{@first_name} #{@last_name}"
end
def self.company_name
'Ruby LLC'
end
end
employee1 = Employee.new('Mark', 'David')
class << employee1
def responsiblity
'Handle recruitment'
end
end
puts employee1.responsiblity
Output:
Handle recruitment
The above example is where the class << self
comes into play, self
in the example above refers to the employee1
object.
Therefore, we can rewrite the Employee
class to use the class << self
syntax to define its class method.
Example Code:
class Employee
def initialize(last_name, first_name)
@last_name = last_name
@first_name = first_name
end
def full_name
"#{@first_name} #{@last_name}"
end
class << self
def company_name
'Ruby LLC'
end
end
end
employee1 = Employee.new('Mark', 'David')
puts Employee.company_name
Output:
Ruby LLC