理解 Ruby 中的類 << self
Nurudeen Ibrahim
2023年1月30日
Ruby
Ruby Class
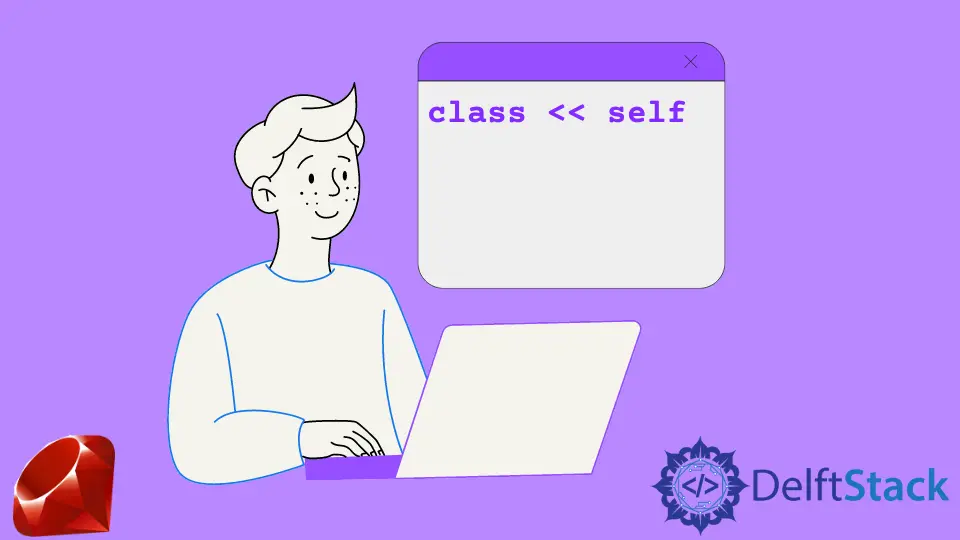
在 Ruby OOP 中,class << self
是你經常遇到的語法。它通常用於定義類方法。
在本文中,我們將瞭解 class << self
語法以及為什麼使用它來定義類方法。
Ruby 中的例項方法和類方法
例項方法和類方法是 Ruby 最常見的兩種方法型別。
示例程式碼:
class Employee
def initialize(last_name, first_name)
@last_name = last_name
@first_name = first_name
end
def full_name
"#{@first_name} #{@last_name}"
end
def self.company_name
"Ruby LLC"
end
end
employee1 = Employee.new("Mark", "David")
puts employee1.full_name
puts Employee.company_name
輸出:
David Mark
Ruby LLC
full_name
是上面示例中的 instance
方法,company_name
是 class
方法。full_name
特定於 Employee
的一個例項,而 company
應該為類的每個例項返回相同的值,定義為 self.company_name
。
示例中的 self
指的是類本身,因此,我們也可以將其寫為:
class Employee
def Employee.company_name
"Ruby LLC"
end
end
Ruby 中的 singleton
方法
還有另一種方法稱為 singleton
,它僅針對特定物件定義。它通常用於圖形使用者介面 (GUI) 設計,其中不同的元素需要採取不同的操作。
如下所示,我們可以為 employee1
物件編寫一個單例方法。
示例程式碼:
class Employee
def initialize(last_name, first_name)
@last_name = last_name
@first_name = first_name
end
def full_name
"#{@first_name} #{@last_name}"
end
def self.company_name
"Ruby LLC"
end
end
employee1 = Employee.new("Mark", "David")
def employee1.responsiblity
"Handle recruitment"
end
puts employee1.responsiblity
輸出:
Handle recruitment
下面是上面程式碼的另一種寫法:
示例程式碼:
class Employee
def initialize(last_name, first_name)
@last_name = last_name
@first_name = first_name
end
def full_name
"#{@first_name} #{@last_name}"
end
def self.company_name
"Ruby LLC"
end
end
employee1 = Employee.new("Mark", "David")
class << employee1
def responsiblity
"Handle recruitment"
end
end
puts employee1.responsiblity
輸出:
Handle recruitment
上面的例子是 class << self
發揮作用的地方,上面例子中的 self
指的是 employee1
物件。
因此,我們可以重寫 Employee
類以使用 class << self
語法來定義其類方法。
示例程式碼:
class Employee
def initialize(last_name, first_name)
@last_name = last_name
@first_name = first_name
end
def full_name
"#{@first_name} #{@last_name}"
end
class << self
def company_name
"Ruby LLC"
end
end
end
employee1 = Employee.new("Mark", "David")
puts Employee.company_name
輸出:
Ruby LLC
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe