The do Keyword in Ruby
-
Use the
do
Keyword to Define an Argument in Ruby -
Use the
each_with_index
Method With thedo
Keyword to Accept a Block of Multiple Arguments in Ruby -
Use the
map
Method With thedo
Keyword to Transform Elements in Ruby - Conclusion
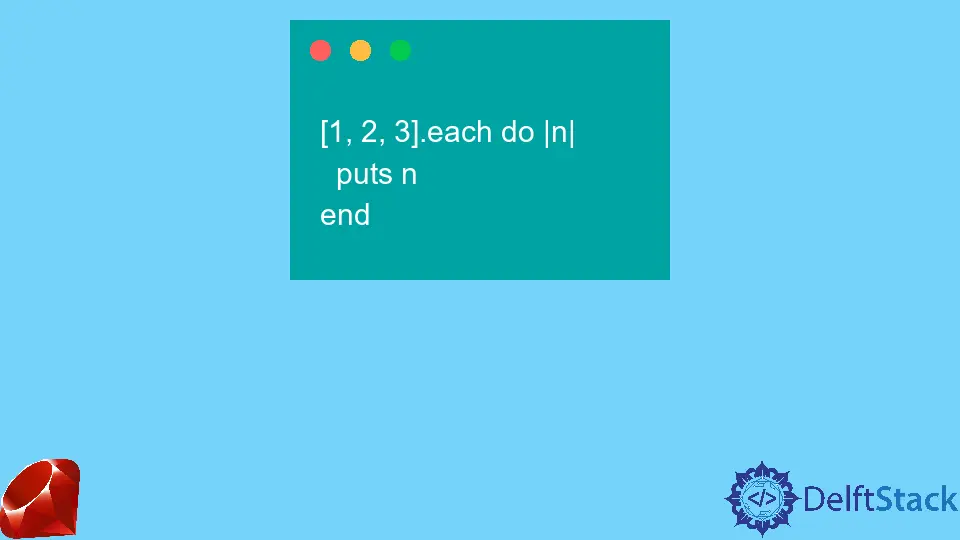
The do
keyword comes into play when defining an argument for a multi-line Ruby block. Ruby blocks are anonymous functions that are enclosed in a do-end
statement or curly braces{}
.
Usually, they are enclosed in a do-end
statement if the block spans through multiple lines and {}
if it’s a single-line block.
In Ruby, the do
keyword is used to define a block of code. The basic syntax for using the do keyword is as follows.
# Single-line block
method_or_iterator do |parameter|
# Code to be executed for each iteration
end
# Multi-line block
method_or_iterator do |parameter|
# Code line 1
# Code line 2
# ...
end
The parameter
is a variable that represents the value(s) yielded to the block during each iteration. You can choose any valid variable name.
The block starts with the do
keyword, followed by the block parameters within vertical bars (| |
). The code inside the block is executed for each iteration, either on a single line or multiple lines.
Use the do
Keyword to Define an Argument in Ruby
In Ruby, the do
keyword is often used to define a block of code, especially when passing a block as an argument to a method.
When you want to pass a block of code as an argument to a method, you use the do
keyword to start the block and end
to finish it. The block is a set of instructions that can be executed within the context of the method.
Example:
# Example method that takes a block
def do_something
puts 'Before block'
yield # Executes the block passed to the method
puts 'After block'
end
# Using the method with a block defined using `do` and `end`
do_something do
puts 'Inside the block'
end
Output:
Before block
Inside the block
After block
In this example, do_something
is a method that takes a block. When calling the method, a block is provided using the do
and end
keywords.
The block contains the code to be executed within the method.
The do
keyword is often used in conjunction with method calls that accept blocks, providing a clean and readable way to define the code to be executed within the context of that method.
Use the each_with_index
Method With the do
Keyword to Accept a Block of Multiple Arguments in Ruby
The each_with_index
method is a common method in programming languages like Ruby, particularly in the context of iterating over elements in a collection, such as an array or a hash. This method is often used when you need both the element and its index during the iteration.
This method is typically used with the do
keyword. The do
keyword, in this case, is used to start a block of code that will be executed for each iteration.
To understand better, below is an example of how the each_with_index
is used together with the do
keyword.
Example:
fruits = %w[apple banana orange]
# Using each_with_index with do for multiple arguments
fruits.each_with_index do |fruit, index|
puts "Index: #{index}, Fruit: #{fruit}"
end
Output:
Index: 0, Fruit: apple
Index: 1, Fruit: banana
Index: 2, Fruit: orange
In this example, each_with_index
iterates over the fruits
array, and for each iteration, the block (defined by do...end
) is executed. Inside the block, fruit
represents the current element, and index
represents its index. The code prints out both the index and the corresponding fruit for each iteration.
This method is useful when you need to perform some operation on each element of an enumerable and also need to know the index of each element in the process.
Use the map
Method With the do
Keyword to Transform Elements in Ruby
Another common use of the do
keyword in Ruby is when working with the map
method.
The map
method transforms each element into an array based on the provided block. When combined with the do
keyword, it allows you to define the transformation logic.
Example:
original_array = [1, 2, 3]
transformed_array = original_array.map do |n|
n * 2
end
puts transformed_array
Output:
2
4
6
In this code, we use the map
method to iterate through each element of the original_array
. Within the block specified by do |n| ... end
, we perform a transformation on each element.
Specifically, we multiply each element by 2
. The resulting array, named transformed_array
, captures these modified values.
Our use of the do
keyword, combined with the map
method, proves to be a potent approach for generating a new array. This new array contains elements that have been derived from the original array, with the transformation dictated by the provided block.
Conclusion
In summary, the do
keyword in Ruby is pivotal for defining multi-line blocks, especially when passing blocks as arguments to methods.
It is commonly used with methods like each_with_index
for iterating over collections with multiple arguments and map
for transforming array elements. The choice between do-end
and curly braces {}
depends on the block’s structure.
Overall, the do
keyword enhances readability and functionality in various Ruby programming scenarios.