How to Render Multiple Components in React
- Why You Can’t Render Multiple Components in React
-
Render Multiple Components in React With
<div>
- Render Multiple Components in React With Fragments
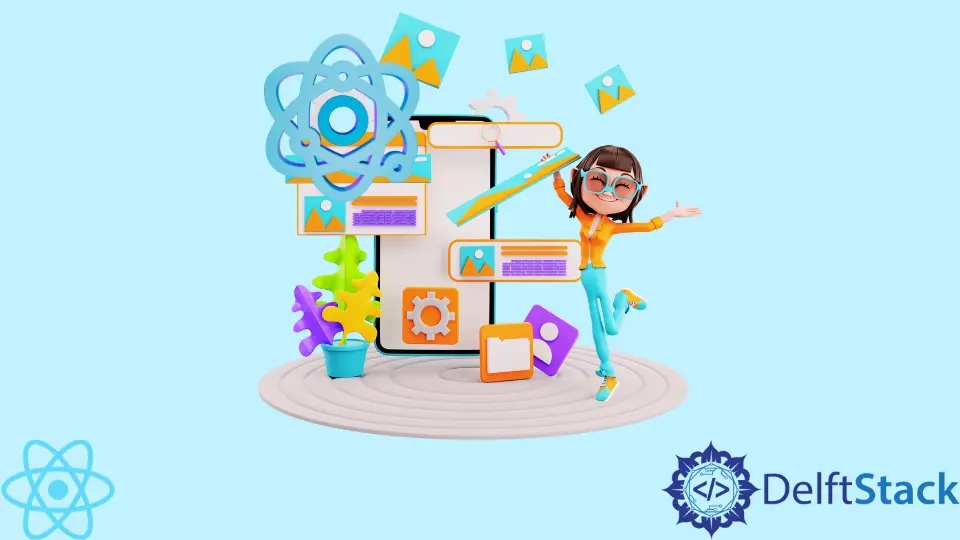
Most beginners who try to learn React are usually confused about one thing. React has a strict rule; you cannot render multiple components in one call.
Do not be confused. You can render an entire component tree that contains hundreds of components, but all of them must have only one parent element.
In this article, we want to show the easiest ways to render multiple components in React.
Why You Can’t Render Multiple Components in React
The ReactDOM.render()
method does not allow you to render multiple components in one call because every call to this method needs a root container. If you want to render many components, as is often the case, you must wrap them all in one element or a container.
Render Multiple Components in React With <div>
Let us look at a practical example. Here, we have an App
component, which returns some headers:
export default function App() {
return (
<h1>Hello CodeSandbox</h1>
<h1>Title for the first blog post</h1>
<h1>Title for the second blog post</h1>
<h1>Title for the third blog post</h1>
);
}
As you can probably guess, this component produces a SyntaxError:
This is fairly descriptive. We know that these elements need to be wrapped with one parent element.
The solution is simple: wrap all of them with a <div>
HTML element.
export default function App() {
return (
<div>
<h1>Hello CodeSandbox</h1>
<h1>Title for the first blog post</h1>
<h1>Title for the second blog post</h1>
<h1>Title for the third blog post</h1>
</div>
);
}
Look at the live CodeSandbox demo; everything works as it should. Now, remove the <div>
, and you will get the error again.
Render Multiple Components in React With Fragments
With React v16.2, developers can use React fragments to group children under one element without unnecessarily creating a DOM node.
The syntax is simple:
export default function App() {
return (
<React.Fragment>
<h1>Hello CodeSandbox</h1>
<h1>Title for the first blog post</h1>
<h1>Title for the second blog post</h1>
<h1>Title for the third blog post</h1>
</React.Fragment>
);
}
Many people use the shorthand syntax, which is much more convenient:
export default function App() {
return (
<>
<h1>Hello CodeSandbox</h1>
<h1>Title for the first blog post</h1>
<h1>Title for the second blog post</h1>
<h1>Title for the third blog post</h1>
</>
);
}
This is far more elegant and efficient than creating a new <div>
wrapper.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn