How to Update State Array in React
- What is a State in React
-
Use
setState()
Method in React - Update Array State Values in React
- Other Ways to Clone the Array or Add the Element in React
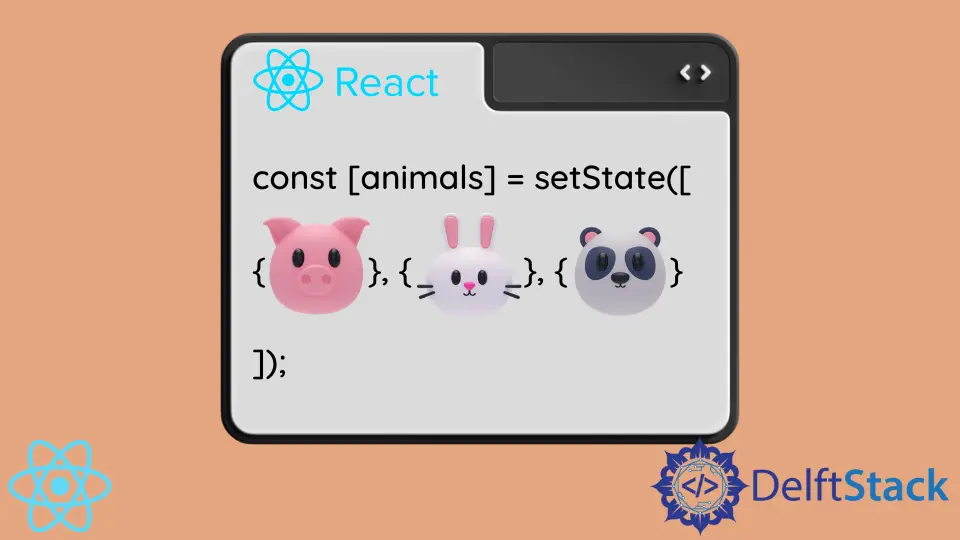
This article wants to explore the state
object and the underlying reasons for limitations on directly mutating the state.
What is a State in React
React is a library that gives you the freedom to develop and style reusable components. Web applications built in React are faster and more customizable than common CMS websites like WordPress or website builder software like SquareSpace.
React is not an opinionated library, and the team behind it gave React developers free to do things their way. Still, the library is founded on certain principles, such as props’ immutability and the necessary procedures for updating the state.
Some React components maintain their state to store data expected to change due to user actions, loaded data, or other events. It is an object, and users can assign their key-value pairs to this object.
One of the most important features of a state
is that the changes to state
values automatically trigger the re-render. If you use a changeable value to render your application, a state
is a perfect place to store it.
If you need a variable to do calculations outside the render
method, you can define a simple variable or set a property on the component instance.
The biggest difference between the state
and props
of a component is that a state
is supposed to change by design.
For React Virtual DOM features to work, you must use built-in methods for updating the state. You can’t use methods like .push()
to directly mutate the state object.
Use setState()
Method in React
React components internally use the setState()
method to modify the state. It is a strong recommendation from React team to only mutate the state using the setState()
method and not bypass it.
It takes one argument: an object that’s supposed to take the place of the existing state. React developers often have to copy the existing state object, make the necessary changes to the copy, call the setState()
method, and provide the copy as an argument.
Update Array State Values in React
There is no limitation on the type of value for state properties. It can be anything: a string, number, object, or even array. It’s common to have arrays as state values in React.
Let’s use a practical example. Let’s imagine your state value is an array containing multiple objects, each of which contains animal data.
Let’s see how to use that state
value in practice.
import "./styles.css";
import { useState } from "react";
export default function App() {
const [animals, setAnimals] = useState([
{ type: "Lion" },
{ type: "Rabbit" },
{ type: "Wolf" }
]);
return (
<div className="App">
{animals.map((animal) => (
<h1>{animal.type}</h1>
))}
</div>
);
}
So far, everything is simple. We use the useState()
hook to set the animals
state variable’s default value to an array. Then we use the .map()
method to render an <h1>
element for each animal.
But what happens when you need another animal to an array? For instance, let’s add a form where users can enter the type of animal.
Once we access the value from the input
element, we will have to somehow add that value to the animals
state variable, which is an array.
export default function App() {
const [animals, setAnimals] = useState([
{ type: "Lion" },
{ type: "Rabbit" },
{ type: "Wolf" }
]);
const handleClick = () => setAnimals([...animals, { type: "Sparrow" }]);
return (
<div className="App">
{animals.map((animal) => (
<h1>{animal.type}</h1>
))}
<button onClick={() => handleClick()}>Update the State</button>
</div>
);
}
If you tried to do animals.push({type:"cat"})
directly, it would lead to errors in your code.
Instead, in this example, we add a simple button that, when clicked, uses the spread syntax to copy all the values of the original animals
state array, add one more object, then update the state. You can check out the live demo on CodeSandbox.
It might seem inefficient to copy the array, modify it, and use the mutated array to update the state variable. Still, it’s the most straightforward approach while aligning with React recommendations.
Other Ways to Clone the Array or Add the Element in React
In the above example, we used the spread syntax to copy the array, add one more object and update the state. However, it’s also possible to use the .concat()
method to add specific values to the array.
const newAnimalsArray = animals.concat([{ type: "Sparrow" }])
Then we can call the setAnimals()
updater function and provide the newAnimalsArray
as an argument.
Similarly, we can use the .push()
method to add certain items to the end of the array.
Alternatively, we can use the .from()
method on the Array
namespace to copy an array. Let’s take a look.
const copiedArray = Array.from(animals)
copiedArray.push({type: "Sparrow"})
setAnimals(copiedArray)
Class Components in React
Class components were the only type that could maintain the state in the past. setState()
is the only valid method for updating the state in class components.
However, it is slightly more verbose than the useState()
hook.
Functional Components in React
Since the introduction of hooks in React version 16.8, it has become possible to maintain the state in functional components. This has been a very popular feature and prompted the React developer community to use functional components more frequently.
In functional components, we define two variables together. The first variable refers to the state value itself, and the second value returned by the useState()
hook is the updater function.
Let’s pretend we have a number
state variable and another setNumber
function to update.
const [number, setNumber] = useState(0)
The useState
hook takes care of the rest. It assigns the state value to the number
variable and the updater function to the setNumber
variable.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn