How to Re-Render Components on State Change in React
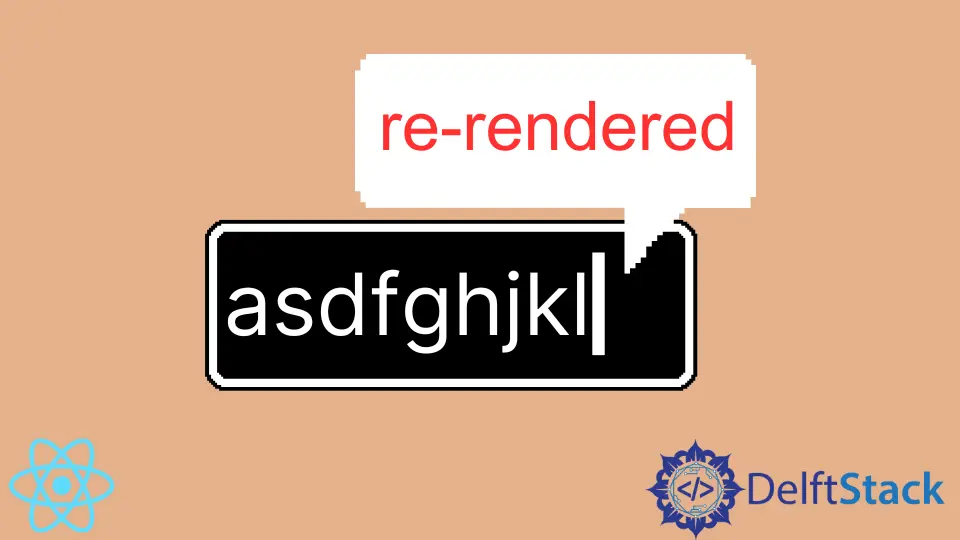
One of the main reasons for the popularity of React is its flexibility, efficiency, and the freedom it affords for building dynamic web applications. The state is one of the foundational innovations of the React library.
Maintain State in React
Every React component can have its internal state object, which stores the changed values. Whenever state values change, applications will be re-rendered as well.
The natural question is, what kind of values should be contained in the state?
There is no limit on what you can store in the state. It can be any data type (string, integer, array, objects).
In practice, the state is often used to store data received from the API and values that depend on the user’s input—for example, theme and authentication settings. In the state object, you could have a darkMode
property.
Then you can apply conditional styles to implement the Dark Mode feature in your web app. When the value of this property is false
, your app will have a light background and dark text; if it is true
, it will have a dark background and white text.
Re-Render in React
React lives up to its name, as state value changes trigger the re-render of the application. By default, every time the state changes, all the components re-render.
At first glance, this default behavior seems inefficient. You might have a component with many children, and changing just one state variable should not re-render all components in the tree.
In the best-case scenario, React should re-render only the components that display the updated value.
The thing is that for all intents and purposes, that’s what React does. In Virtual DOM, a re-render of one state or prop
value leads to re-rendering other components in the tree.
However, these re-renders are limited to Virtual DOM, which is only a shadow of Real DOM, and therefore, re-renders do not consume too many resources.
In the final stage, React compares Virtual DOM with Real DOM and updates only the parts of Real DOM that are different from Virtual DOM. This way, React ensures that all changes are reflected in the DOM and, at the same time, stays efficient.
React Components Re-Render on State Change
React components re-render whenever you use the setState()
method (or the useState()
hook) to update the state.
Let’s look at the live example:
import "./styles.css";
import { useState } from "react";
export default function App() {
console.log("re-rendered");
const [text, setText] = useState("");
return (
<div className="App">
<input type="text" onChange={(e) => setText(e.target.value)} />
<h1>You entered: </h1>
<p>{text}</p>
</div>
);
}
Here, we have a simple application with one <input>
element, one <h1>
, and one <p>
. We set up one state variable, text
, updated every time someone enters the value into the text input.
Go to the live demo on CodeSandbox, where this code is deployed. If you open the console, you’ll see the text re-rendered
output to the console every time we enter something into the field.
the shouldComponentUpdate()
Method
It helps to understand what’s going on under the hood and how React determines that component needs to re-render.
Every component has a shouldComponentUpdate()
method which returns a Boolean value. As the name suggests, this method defines the criteria for when the component should and should not re-render.
By default, the criteria are these: component updates whenever it receives new props
or whenever you make changes to the state.
By default, the shouldComponent()
method has a broad definition. Whenever the current or parent component receives a new prop
value or state
value changes, it returns true
.
This can avoid errors and delays in displaying significant changes by skipping or re-rendering the component.
However, if you are confident that you can follow common React guidelines for working with state values, e.g., not mutating them in place, you can create a custom shouldComponentUpdate()
definition.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn