How to Push Data Into State Array in React
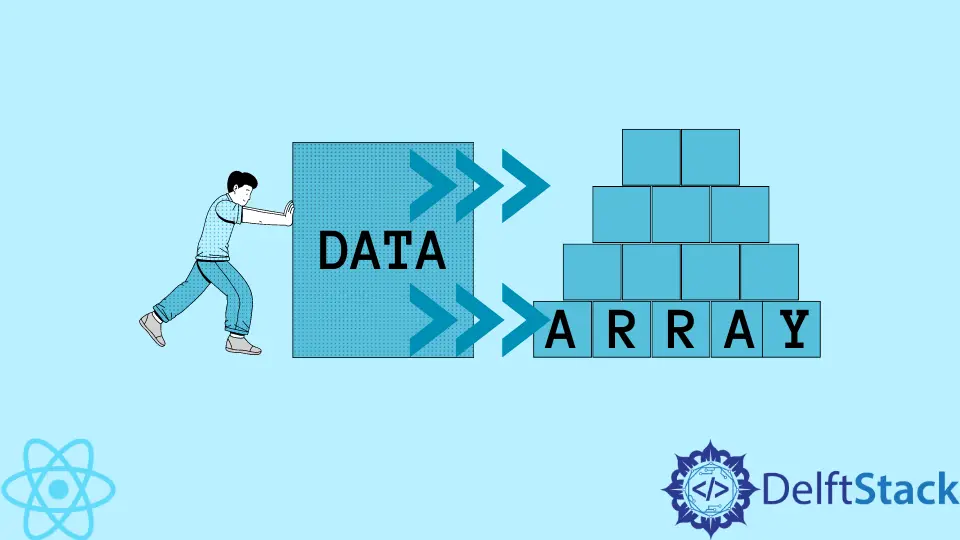
React is the most popular front-end library because it gives developers the freedom to build dynamic, interactive applications with code efficiency. This framework has many great features.
This article will focus on the state
object, which is essential for implementing dynamic functionality in React. More specifically, we will show how to maintain and update the state
in React.
Push Data Into State Array in React
Many JavaScript developers use the .push()
method to add items to the end of the array. This works fine in plain JavaScript, but if the array is a state variable (or a property of a state
object), using the .push()
method can have unexpected results.
When using the .push()
method, we need to understand the difference between the side effects and return values. It returns the length of the updated array, not the updated array, with new items added to the end.
Pushing items into the array is just a side effect.
Not only that, but React has a strict rule against directly mutating the state
object. Therefore, you can’t push items directly into a state
property that contains an array value.
To update the state
object in React, you need to use the setState()
method and supply the updated state
as an argument.
You can also use the useState()
hook, which provides a function to update one state
variable directly.
Let’s take a look at the example:
import "./styles.css";
import { useState } from "react";
export default function App() {
let [arr, setArr] = useState([1, 2, 3, 4]);
// arr.push(5) wrong
const numberToBeAdded = 5
console.log(numberToBeAdded);
return (
<div className="App">
<button onClick={() => setArr((arr) => [...arr, numberToBeAdded])}>Expand array</button>
</div>
);
}
Take a look at the live example. Open the console to see the changes to the state value.
We used the useState()
hook to define the arr
state variable and the setArr
function to update that variable. We supplied one argument to the useState()
hook - the initial value of the array.
React does not allow you to use the .push()
method to mutate the arr
state variable directly. If we want to add an integer value 5 to the array, arr.push()
is not the right way to do it.
Instead, the best way to add an item to the array is to have a function that takes one argument - the old state variable - and returns the new state variable.
Here is the handler function:
() => setArr((arr) => [...arr, numberToBeAdded])
As you can see, the argument to the setArr()
function is a callback function that takes a current value of the arr
state variable and returns the new one.
To add the number at the end of the array, we used the spread operator, also known as three dots. ...arr
takes all the values from the current array, and we add the value contained in the numberToBeAdded
variable.
You can use the same approach to add value at the start:
() => setArr((arr) => [numberToBeAdded, ...arr])
Simply move the value that needs to be added to the front.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn