How to Implement Dropdowns in React - Select and onChange
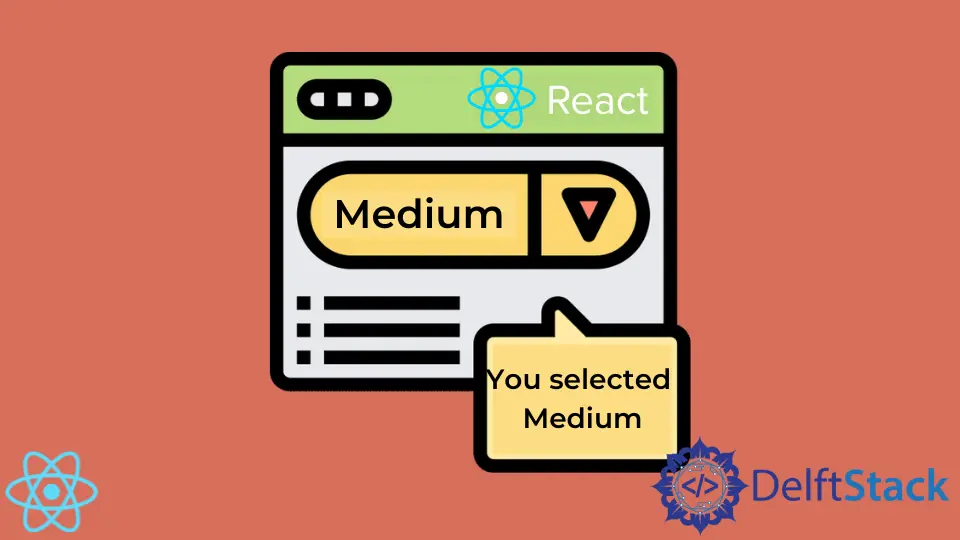
To create interactive applications, React developers must create input elements and handle the events. Dropdown forms are widespread and one of the most useful types of input. They allow the users to select one of the many options or have one selected by default. Once the option is selected, we can access its value and store it in the component state.
The primary purpose of the select form is to collect users’ data. For instance, you might want to ask users to select their gender.
The purpose of event handlers is to track users’ actions to update the component state. When it comes to the <select/>
element, the onChange
event handler is the most useful because it fires when users select a different <option/>
.
Custom Select Component in React
If you need a normal <select/>
element with complex functionalities, it’s probably best to create a separate component. This way, you can set up event handlers, store select values, and, if necessary, trigger changes in the parent component. You can also use props
to feed the data into a custom select
component. Let’s look at a simple example:
class CustomSelect extends Component {
constructor(props){
super(props)
this.state = {
selectedValue: ""
}
}
render() {
const handleChange = (e) => {
this.setState({selectedValue: e.target.value})
}
return (
<div>
<select onChange={(e) => handleChange(e)}>
<option value="1-10">1-10</option>
<option value="10-15">10-15</option>
<option value="15-20">15-20</option>
<option value="20+">20+</option>
</select>
<h1>You chose {this.state.selectedValue}</h1>
</div>
);
}
}
This is a very basic component that encapsulates the <select>
element’s main functionalities. Users can select one of the options, and the selectedValue
state property will store the value of their choice.
Note that the change
event occurs on the <select>
element, not on its children. Users have multiple options to choose from, and whenever they change their choice, the event handler in the onChange
attribute will be executed to update the component state.
Each time the state changes, the component will re-render and will display the updated value in the <h1>
text. Try it out yourself on the playcode.
Rendering an Array of Options
Often components are much more useful if they adjust their output based on data passed via props
. In our example, we have a custom component that incorporates the core functionalities of the <select>
element. However, having a hard-coded set of options in our JSX code defeats the purpose of creating a custom component. It would be much more practical to accept an array of options via props
and render the options accordingly. Here’s an example:
class CustomSelect extends Component {
constructor(props){
super(props)
this.state = {
selectedValue: ""
}
}
render() {
const handleChange = (e) => {
this.setState({selectedValue: e.target.value})
}
return (
<div>
<select onChange={(e) => handleChange(e)}>
{this.props.options.map(arrayItem => <option value={arrayItem}>{arrayItem}</option>)}
</select>
<h1>You chose {this.state.selectedValue}</h1>
</div>
);
}
}
CustomSelect.defaultProps = {
options: ["1-10", "10-15", "15-20", "20+"]
}
This approach gives us more freedom to use our <CustomSelect>
component in many different ways. If we create a select
element multiple times, we’ll only need to pass option arrays as props. For example:
return (
<div>
...
<CustomSelect props=[firstArr]></CustomSelect>
<CustomSelect props=[secondArr]></CustomSelect>
...
</div>
);
Functional Components
Our examples so far only use class components. You can also create custom select components with functions. In fact, since the introduction of hooks, React developers are starting to favor functional components strongly. To learn more about the differences between functional and class components, read this guide.
Using JSX
If you need to use <select>
just once, you can include the element directly in your JSX. In case you don’t know, JSX is a special syntax for React, which looks a lot like regular HTML syntax, but gives developers more power to create dynamic applications.
Here’s what a JSX implementation would look like:
class App extends Component {
constructor(props){
super(props)
this.state = {
selectedValue: ""
}
}
render() {
const handleChange = (e) => {
this.setState({selectedValue: e.target.value})
}
return (
<div>
<p>Choose the size of the t-shirt</p>
<select onChange={(e) => handleChange(e)}>
<option value="S">S</option>
<option value="M">M</option>
<option value="L">L</option>
<option value="XL">XL</option>
</select>
</div>
);
}
}
react-select
Package
If you’re looking for an advanced solution, consider installing the react-select
package, which provides a custom Select
with extensive functionalities like autocomplete and multi-select. Look over their website to gain a better understanding of the features.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn