How to Get Selected Value of a Dropdown Menu in React
- Controlled Components to Get Dropdown Menu Value in React
- Uncontrolled Components to Get Dropdown Menu Value in React
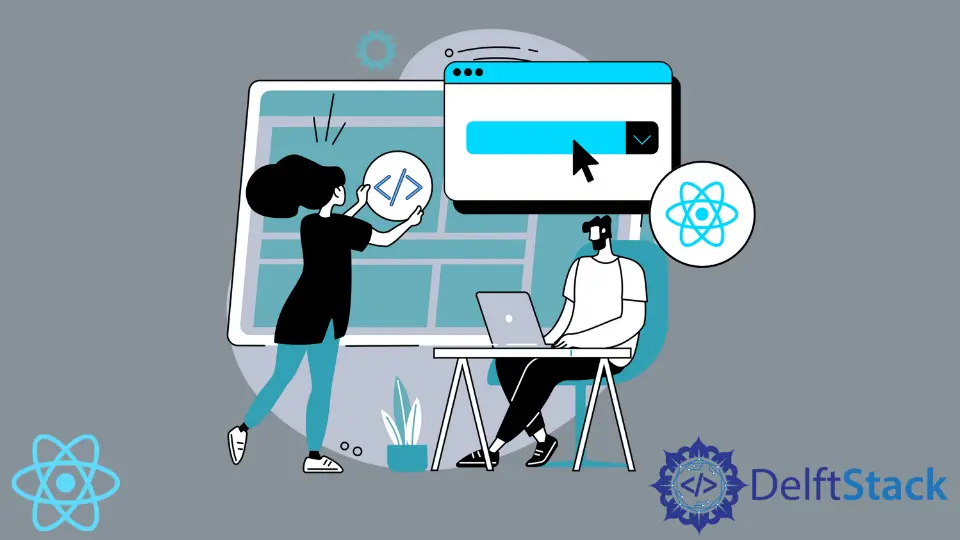
React is a popular JavaScript framework for building dynamic applications. To build a functioning application, you must set up reactivity, so your application responds to the user’s input.
It can be a checkbox to let them subscribe to your emailing list, a radio button to choose the correct answer, a text field to get their full name, or any other types of inputs.
This article will discuss dropdown menus, specifically the <select>
and <option>
HTML elements, how to use them in JSX, and how to get the value from a dropdown menu in React.
Controlled Components to Get Dropdown Menu Value in React
React state
allows you to store user selections as values and use them throughout your application. The components that take the value from user inputs and store it in their internal state
are controlled components.
Let’s take a look at a simple application that lets users choose one of the three fruits, and displays users selection as a text:
export default function App() {
const [fruit, setFruit] = useState();
return (
<div>
<select id="fruits" value={fruit}
onChange={(e) => setFruit(e.target.value)}>
<option value="Apple">Apple</option>
<option value="Pear">Pear</option>
<option value="Pineapple">Pineapple</option>
</select>
<h1>Selected Fruit: {fruit}</h1>
</div>
);
}
We use the useState()
hook to update the state variable in this example.
First, we must set up an event listener on the <select>
element and define an event handler. In our example, it takes one argument - e
(short for event) and calls the setFruit()
function.
To set the state with the selected value, we access the e.target.value
from the event object and pass it to the setFruit()
function.
Finally, to display our selection, we place the state variable fruit
between curly brackets to ensure that JSX interprets it as a JavaScript expression.
You can play around with the code on CodeSandbox.
Uncontrolled Components to Get Dropdown Menu Value in React
Instead of setting the initial value of the select
element in the state
of your component, you can use the defaultValue
attribute to set the default value. In this case, the select
JSX element would look something like this:
export default function App() {
const [fruit, setFruit] = useState();
return (
<div>
<select id="fruits" defaultValue="Select fruit"
onChange={(e) => setFruit(e.target.value)}>
<option value="Apple">Apple</option>
<option value="Pear">Pear</option>
<option value="Pineapple">Pineapple</option>
</select>
<h1>Selected Fruit: {fruit}</h1>
</div>
);
}
With this approach, you only change how you set and access the default value of the select
element. Otherwise, everything is the same: we use the onChange
handler to update the state.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn