How to Pass Parameter with onClick Method in React
-
Use the
Pass
Parameter in onClick Using React -
Use the
e.target.value
to Save the Value From the Parameter in React
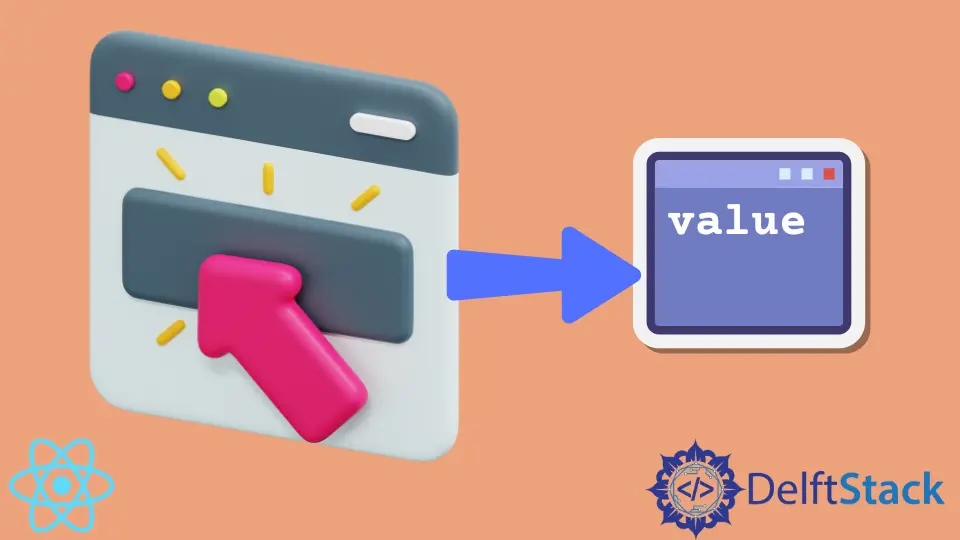
We will introduce a parameter with the onClick
method in react. We will share examples of how to pass a parameter using the onClick
method of the button.
Use the Pass
Parameter in onClick Using React
Most of the junior developers are stuck on how to pass the parameters from the onClick
method to the functions or pass the parameters from the input fields when a user clicks a button on the form.
We will create a couple of buttons in this example and pass the parameters by clicking on them. First, we will create a new application and style it by importing a stylesheet.
# react
npx create-react-app my-app
After creating our new application in react, we will go to our application directory using this command.
# react
cd my-app
Now, let’s run our app to check if all dependencies are installed correctly.
# react
npm start
In App.js
, we will import a stylesheet below.
# react
import "./styles.css";
Use the e.target.value
to Save the Value From the Parameter in React
In return, we will create a template with two buttons that will call to a function getValue
with the onClick
method.
We will set values for both buttons as 1
and react
. getValue
function will take e
as a parameter that will contain the value of the button we click on.
We can easily save the value from the parameter using the e.target.value
. Once we have got the value from the button, we will console.log()
it.
So our code in App.js
will look like below.
# react
import "./styles.css";
export default function App() {
function getValue(e) {
const value = e.target.value;
console.log(value);
}
return (
<div className="App">
<h1>Pass Parameter OnCick</h1>
<div>
<button className="btns" value="1" onClick={getValue}>
Value 1
</button>
<button className="btns" value="React" onClick={getValue}>
Value 2
</button>
</div>
</div>
);
}
Now, let’s add some CSS style to make our buttons centered and change their background colors.
# react
.App {
font-family: sans-serif;
text-align: center;
}
.btns {
background: black;
color: white;
border: none;
padding: 10px;
margin-left: 10px;
}
.btns:hover {
background: white;
color: black;
border: 1px solid black;
}
Output:
As you can see in the above example, when we click on any button, it sends all data to the function getValue
.
We assign the data to the parameter e
we used in the function. Once the data is assigned to the parameter, we can easily get the value from the parameter and use it further.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn