Asynchronous Behavior of the setState Method in React
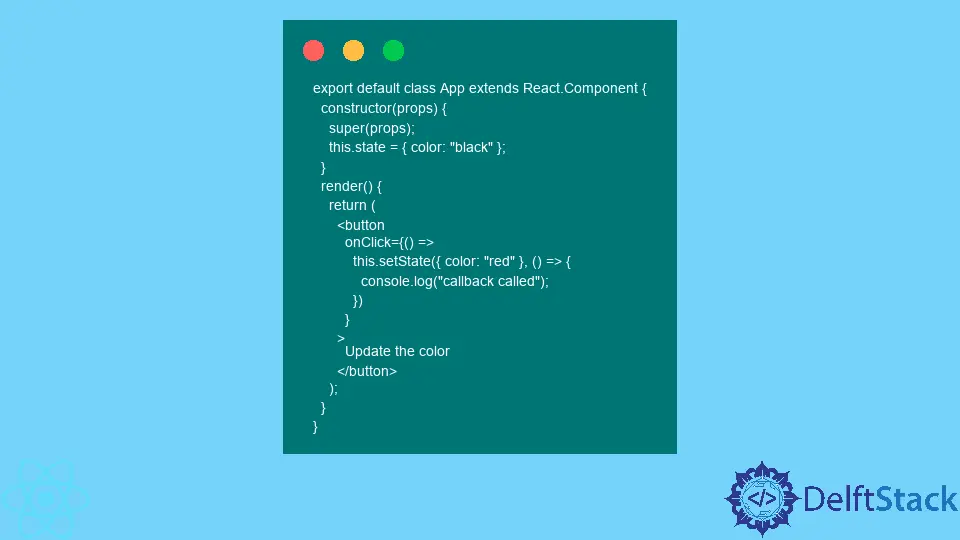
Building dynamic applications is impossible, or at least very difficult, without maintaining a state. Fortunately, every component in React library can have its own internal state.
Inexperienced React developers often don’t understand the intricate behavior of state
objects in React. In this article, we want to clear up some of the confusion.
Async Behavior of the setState()
Method in React
Many React developers don’t know that the setState()
method is asynchronous. If your application has multiple calls to the setState()
method, they will be batched together to improve the performance and speed of the application.
React team made the setState()
method asynchronous because changing the state can be a trigger for re-rendering the application. This can lead to bugs and unresponsiveness if a component has multiple separate calls to the setState()
method.
Pass Callback Function as an Argument to the setState()
Method
In React, the setState()
method is used to update the state object. Many React developers don’t know that it can accept more than one argument.
The first argument is always the object we want to be in the new state. However, the second argument can be the callback function executed right after the state is updated.
Let’s look at an example:
export default class App extends React.Component {
constructor(props) {
super(props);
this.state = { color: "black" };
}
render() {
return (
<button
onClick={() =>
this.setState({ color: "red" }, () => {
console.log("callback called");
})
}
>
Update the color
</button>
);
}
}
The value of the onClick
event handler is a call to this method. In this case, the first argument is an object which will replace the existing state.
In the second argument, the callback function will be executed right after you update the state.
You can go to this live CodeSandbox demo. If you open the console, you’ll see that clicking the button outputs a 'callback called'
string to the console.
This feature is helpful if you want to perform a specific action right after updating the state. Technically, you can chain multiple calls to the setState()
method to update multiple properties of the object’s state at once; however, there are better ways to do so.
Also, if you want to ensure that one state property is updated after another one, you can do the following:
this.setState({color: 'red'}, () => setState{color: 'red', active: true})
In this case, React will first replace the current state object with the object that is the first argument ({color: "red"}
). Once this operation is complete, it will execute the callback function, adding one more property to the state.
Update Multiple State Properties at Once
If you need to update multiple state properties at once, you can do so in one call to the setState()
method.
From the previous example, it’s easier to update the value of the color
state property and add the active
state property in one setState()
call:
this.setState({color: 'red', active: true})
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn