How to Export Function in React
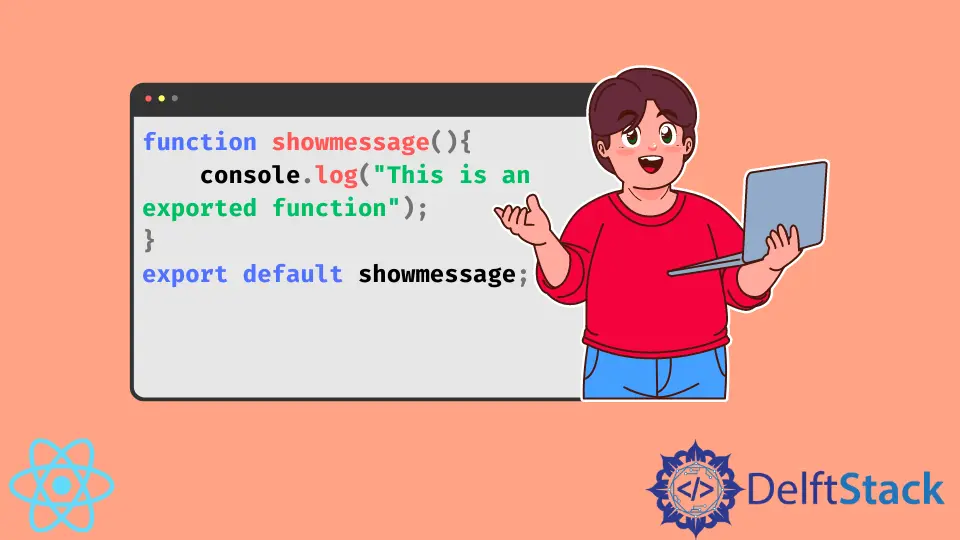
When working with React, one of the fundamental tasks you might encounter is exporting functions. This process allows you to share functionality across different components, making your code modular and easier to maintain. In this short tutorial, we will explore how to export functions in React, focusing on both named and default exports. Understanding these concepts is crucial for any React developer looking to create scalable applications. Whether you’re building a simple project or a complex application, mastering the art of exporting functions will enhance your coding efficiency and improve collaboration within your team. Let’s dive in and learn how to export functions effectively in React!
Understanding Exports in React
In React, you can export functions in two primary ways: named exports and default exports. Each method serves a specific purpose and can be used depending on your needs.
Named Exports
Named exports allow you to export multiple functions from a single file. This approach is useful when you have several related functions that you want to share. Here’s how you can implement named exports in a React component.
// utils.js
export function greet(name) {
return `Hello, ${name}!`;
}
export function farewell(name) {
return `Goodbye, ${name}!`;
}
In the above code, we have two functions, greet
and farewell
, both exported from the utils.js
file. You can import these functions in another file as follows:
// App.js
import { greet, farewell } from './utils';
console.log(greet('Alice'));
console.log(farewell('Alice'));
Output:
Hello, Alice!
Goodbye, Alice!
By using named exports, you can import only the functions you need, which can help keep your code clean and efficient. It also allows for better code organization, especially in larger projects where multiple functions are defined in a single file.
Default Exports
Default exports, on the other hand, allow you to export a single function or component from a file. This method is particularly useful when you want to define a primary function or component that represents the main functionality of the module.
// greeting.js
export default function greet(name) {
return `Hello, ${name}!`;
}
In this example, the greet
function is exported as the default export from the greeting.js
file. You can import it like this:
// App.js
import greet from './greeting';
console.log(greet('Bob'));
Output:
Hello, Bob!
Using default exports simplifies the import statement since you don’t need to use curly braces. It’s a great way to streamline your code when you have a primary function or component that you want to highlight.
Combining Named and Default Exports
You can also combine named and default exports in a single file. This approach allows you to export a main function as the default export while still providing additional utility functions as named exports.
// utils.js
export function farewell(name) {
return `Goodbye, ${name}!`;
}
export default function greet(name) {
return `Hello, ${name}!`;
}
In this case, greet
is the default export, while farewell
is a named export. You can import them as follows:
// App.js
import greet, { farewell } from './utils';
console.log(greet('Charlie'));
console.log(farewell('Charlie'));
Output:
Hello, Charlie!
Goodbye, Charlie!
This flexibility allows you to structure your modules in a way that best fits your application’s architecture. By combining both export types, you can provide a clear and organized API for others to use.
Conclusion
Exporting functions in React is a vital skill that enhances your ability to write modular, maintainable code. By understanding the differences between named and default exports, you can effectively share functionality across your components. Whether you’re working on a small project or a large application, mastering these export techniques will streamline your development process and improve collaboration with your team. So go ahead and experiment with different export methods to see how they can benefit your React projects!
FAQ
-
What is the difference between named and default exports?
Named exports allow you to export multiple functions from a file, while default exports enable you to export a single function or component. -
Can I combine named and default exports in the same file?
Yes, you can combine both export types in a single file, allowing you to export a primary function as the default export and additional functions as named exports. -
How do I import a named export?
You can import a named export using curly braces, like this:import { functionName } from './filePath';
. -
Can I rename a named export when importing it?
Yes, you can rename a named export during import using theas
keyword, like this:import { functionName as newName } from './filePath';
. -
What happens if I don’t use curly braces when importing a named export?
If you don’t use curly braces, JavaScript will look for a default export in that file, resulting in an error if none exists.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn