How to Use of Arrow Functions in React Components
- Arrow Functions in React Class Components
- Arrow Functions in React Functional Components
- Syntax of the Arrow Function in React
- Advantages of Using the Arrow Function in React
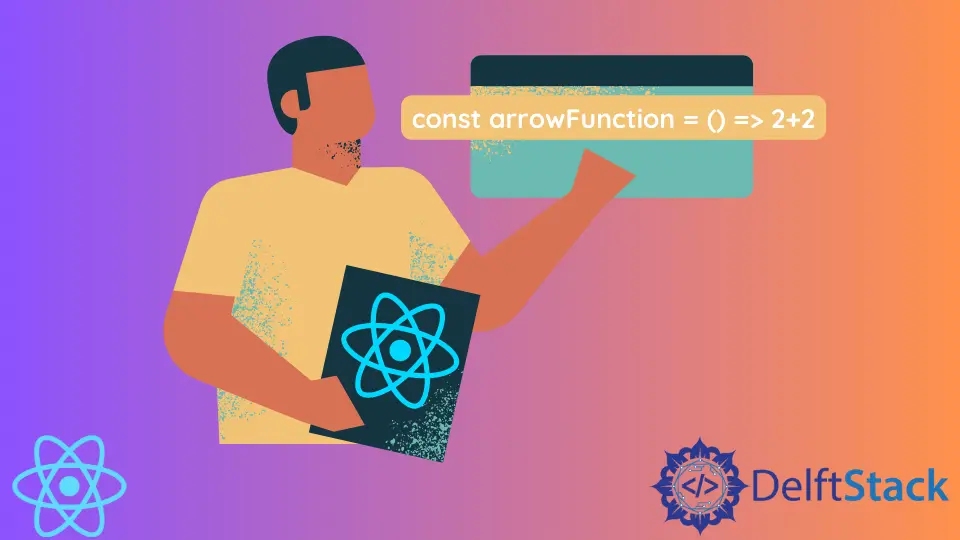
Arrow functions are a recent addition to JavaScript, but they have already changed how millions of developers write JavaScript code.
As you may know, React web applications are made up of multiple components. Often components are reused; for example, an eCommerce store might have multiple instances of a <Product>
child component.
Arrow Functions in React Class Components
React allows you to define components using class syntax. If you want to use the this
keyword in React, you need to bind it in the constructor; otherwise, normal functions referencing the this
keyword will return undefined.
Code:
export default class App extends React.Component {
constructor(props) {
super(props);
}
handleClick(e) {
console.log(this);
}
render() {
return <button onClick={this.handleClick}>Log to the console</button>;
}
}
If you need to reference the this
keyword in regular functions, you will need to bind it to the constructor. If you don’t do this, the handleClick()
function will not log the App
component to the console but is undefined
.
Arrow functions in class components work differently. Using the this
keyword is much more straightforward.
The keyword refers to the environment where the arrow function exists, the <App>
component itself. For example, a small rewrite of the component above can significantly change its behavior:
Code:
export default class App extends React.Component {
constructor(props) {
super(props);
}
render() {
return (
<button onClick={(e) => console.log(this)}>Log to the console</button>
);
}
}
If you open the live demo on CodeSandbox and click the button, you’ll see that the console shows the class component.
Arrow Functions in React Functional Components
Functional components do not have the this
keyword, so the distinction between the arrow and normal syntax is much smaller. In functional components, arrow functions are a cleaner, more succinct way to write event handlers in React.
This function is often used in functional components to write inline event handlers in JavaScript. Let’s look at an example:
export default function App() {
return (
<div className="App">
<button onClick={() => alert("hello!")}>Create an alert</button>
</div>
);
}
You can also store arrow functions in a variable and pass down these event handlers as props
.
export default function App() {
const handler = () => console.log("An example string");
return (
<div className="App">
<ChildComponent handler={handler} />
</div>
);
}
It’s also possible to write the functional component as an arrow function.
const App = () => {
return (
<div className="App">
<ChildComponent handler={handler} />
</div>
);
}
Syntax of the Arrow Function in React
React is a JavaScript-based library. So, you can use arrow functions outside of JSX.
The syntax for using arrow functions in React components is fairly simple. Let’s look at a simple example of arrow functions that do not take any arguments and return one expression:
const arrowFunction = () => 2+2
In this case, we stored a reference to our function definition in the arrowFunction
variable.
The function doesn’t take any parameters, so we use empty parenthesis ()
, followed by an arrow =>
between function arguments and its body. If the function body is only one expression on one line, you don’t need to use curly braces to separate the function body.
Also, you don’t need to write the return
statement explicitly; it is implied. The function above returns the result of the 2+2
expression.
When Passing One Parameter
The syntax of arrow functions follows the pattern described above, with minor differences. Let’s look at an example:
const arrowFunction = argument => argument+2
When you pass just one parameter, you can wrap it with parenthesis or skip using them. Either way, the arrow function will work.
When Passing Two or More Parameters
When you pass multiple parameters, you have to wrap them with a parenthesis.
const arrowFunction = (argumentOne, argumentTwo) => argumentOne+argumentTwo
You could have an array with many items and pass them all at once using the spread operator:
const args = [5,10]
const arrowFunction = (...args) => argumentOne+argumentTwo
When Using Multiple Statements
Now, let’s look at arrow functions that are a bit more complex. The body of most functions you write in React contains multiple statements on multiple lines.
When writing arrow functions with multiple statements, you need to enclose the function body with curly braces:
const arrowFunction = (argumentOne, argumentTwo) => {
console.log("doing calculation")
return argumentOne+argumentTwo
}
In this case, you must also explicitly write the return
statement.
Advantages of Using the Arrow Function in React
Arrow functions have many advantages in React. Perhaps the biggest is that they provide an easier syntax to create functional components in React; you can use arrow functions to define a simple component within minutes.
Arrow functions are also easy to use as callbacks in class components. They often prevent bugs associated with the this
keyword because arrow functions do not change the value of this
within their function body; it always refers to an instance of the class.
Otherwise, arrow functions offer time savings for writing many simple inline event handlers and callback functions.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn