Dynamic Component Name in React
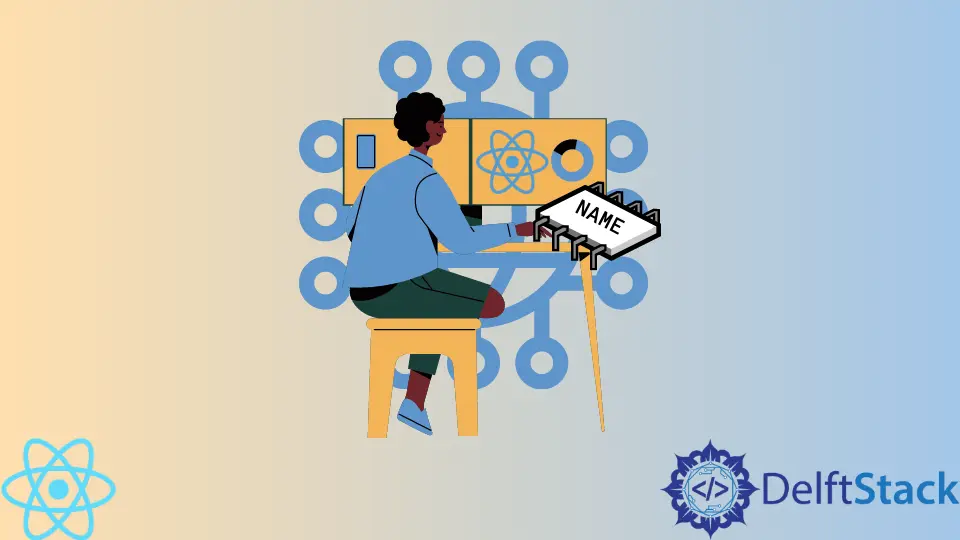
React is one of the most popular choices for building UI. You can use React to immediately respond to users’ actions, such as dynamically displaying and hiding content, applying CSS styles conditionally, and more.
In this article, we will talk about dynamic component names in React.
Dynamic Component Names and JSX
To understand how to dynamically change the name of the rendered component in React, first, we must understand the templating language JSX.
JSX is the default templating language for React. It allows React developers to create a neat hierarchy of components declaratively.
JSX allows developers to build React applications faster than they would be using top-level React API.
It’s important to understand that JSX is only a syntax that is easy to write and read. Under the hood, all React applications are made up of complex calls to methods in the Top-Level API of React, such as createElement()
, createFactory()
, cloneElement()
, and more.
Dynamic Component Name in React
Let’s imagine you have a blog and want to dynamically render different types of components: text, video, or picture.
First, you must have components defined and created before invoking them. You cannot dynamically generate them; however, you can declaratively invoke <SomeComponent>
, which can be interpreted as different components, depending on dynamic values.
Writing <SomeComponent>
in JSX translates to the native-level API of React as a call to the React.createElement(SomeComponent, {})
method. The first argument to React.createElement()
method needs to be an HTML tag or a function; therefore, the SomeComponent
variable needs to reference either an HTML element or a component.
Beginners often mistake generating the component name dynamically and storing it in a variable as a string. This is wrong because the first argument to the React.createElement()
method can not be a plain string.
For example, this is wrong:
var category = "Text";
var SomeComponent = category + "Component";
return <SomeComponent />;
At first glance, everything in the code snippet should work fine, but even if the string value is uppercased, this will not work because the SomeComponent
variable will contain a string value. Instead, it must be a valid React component (or HTML tag).
Correct Way to Have Dynamic Component Name in React
Create an object with property names for each type of component. The value for these properties should reference actual React components.
Then you can have a variable with a dynamically generated string and use this variable to reference one of the components stored in the object.
Let’s look at the actual code:
export default function App() {
const componentNames = {
text: TextComponent,
video: VideoComponent,
picture: PictureComponent
};
var category = "text";
var SomeComponent = componentNames[category];
return (
<div className="App">
<SomeComponent />
</div>
);
}
function TextComponent() {
return (
<div>
<h1>I am a dynamically named Text Component</h1>
</div>
);
}
function VideoComponent() {
return (
<div>
<h1>I am a dynamically named Video Component</h1>
</div>
);
}
function PictureComponent() {
return (
<div>
<h1>I am a dynamically named Picture Component</h1>
</div>
);
}
You can try and edit the code yourself on CodeSandbox.
Here, we have a basic example of one parent and three child components. Technically, the parent only has one child component, but all three components: TextComponent
, VideoComponent
and PictureComponent
can take its place, depending on the value in the category
variable.
Currently, the category
variable is set to text
; we use it to look up the corresponding TextComponent
in the componentNames
object. Once we determine which type of component we need, we store it in the SomeComponent
variable.
In our App
component, we invoke the SomeComponent
variable, which can reference three different components, depending on the string value in the category
variable.
This is a powerful feature you can use to render or not render certain components dynamically. In this case, we have a static string value; however, you can collect and use user input to determine which components need to be rendered and which can be skipped.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn