Controlled and Uncontrolled Components in React
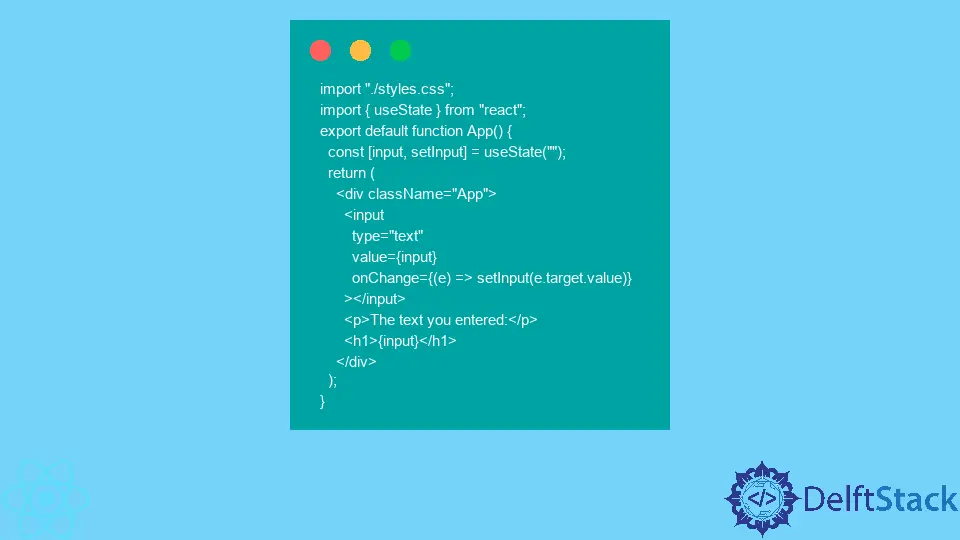
Web developers use JavaScript-based frameworks for many reasons, mainly because they allow us to build interactive web applications to provide the best possible user experience.
This article will discuss the concept of controlled components in React and advise how to implement them and when they are necessary.
The main difference between the two types of components is how they maintain the state and how you access the values in input fields.
Controlled Components in React
For this type of component, user inputs are always stored in the state and only updated using the setState()
method. The value
attribute of the <input>
element is set to this state value.
This ensures that the state is the single source of truth. Entering something into the input field will update the state value, and the value in the input field will reflect the most recent state.
In other words, the text field updates the state and also gets its value from the state.
Here is an example of a controlled component.
import "./styles.css";
import { useState } from "react";
export default function App() {
const [input, setInput] = useState("");
return (
<div className="App">
<input
type="text"
value={input}
onChange={(e) => setInput(e.target.value)}
></input>
<p>The text you entered:</p>
<h1>{input}</h1>
</div>
);
}
We import the useState()
hook from the core React
library in this example. We use the hook to create a state variable input
and the function to update its value.
We initialize the state variable to an empty string.
In JSX, we have a <div>
element that contains three elements: <input>
, <p>
, and <h1>
. We set up the input element so that every time the user enters or deletes something, the onChange
event handler will update the state with the most recent value in the input field.
We also set the value
attribute so that the input field gets its value from the state.
Finally, we have an <h1>
element, which will display a header text representing the string in the state variable.
Check out this live demo on CodeSandbox and see how everything works.
Uncontrolled Components in React
Input elements in the DOM can maintain their state. For uncontrolled components, we access the value directly in the DOM element.
In React, we use refs
to access input elements. In this case, we use the ref.current.value
to access the current value in the input element.
Therefore, you will need to create refs
in React to have uncontrolled components. For that, you are going to need either the useRef()
hook for functional components, or React.createRef()
method in class components.
According to React docs, your application should have controlled components only. Uncontrolled components are unpredictable and difficult to maintain.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn